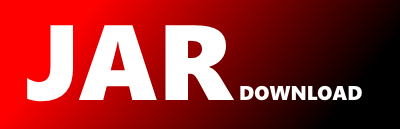
edu.vt.middleware.ldap.handler.TlsConnectionHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: TlsConnectionHandler.java 1616 2010-09-21 17:22:27Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1616 $
Updated: $Date: 2010-09-21 13:22:27 -0400 (Tue, 21 Sep 2010) $
*/
package edu.vt.middleware.ldap.handler;
import java.io.IOException;
import java.util.Hashtable;
import javax.naming.CommunicationException;
import javax.naming.NamingException;
import javax.naming.ldap.InitialLdapContext;
import javax.naming.ldap.LdapContext;
import javax.naming.ldap.StartTlsRequest;
import javax.naming.ldap.StartTlsResponse;
import edu.vt.middleware.ldap.LdapConfig;
import edu.vt.middleware.ldap.LdapConstants;
/**
* TlsConnectionHandler
creates a new LdapContext
* using environment properties obtained from {@link
* LdapConfig#getEnvironment()} and then invokes the startTLS extended operation
* on the context. SSLSocketFactory
and
* HostnameVerifier
properties are used from the
* LdapContext
.
*
* @author Middleware Services
* @version $Revision: 1616 $
*/
public class TlsConnectionHandler extends DefaultConnectionHandler
{
/** Start TLS response. */
private StartTlsResponse startTlsResponse;
/**
* Whether to call {@link StartTlsResponse#close()} when {@link #close()} is
* called.
*/
private boolean stopTlsOnClose;
/** Default constructor. */
public TlsConnectionHandler() {}
/**
* Creates a new TlsConnectionHandler
with the supplied ldap
* config.
*
* @param lc ldap config
*/
public TlsConnectionHandler(final LdapConfig lc)
{
super(lc);
}
/**
* Copy constructor for TlsConnectionHandler
.
*
* @param ch to copy properties from
*/
public TlsConnectionHandler(final TlsConnectionHandler ch)
{
this.setLdapConfig(ch.getLdapConfig());
this.setConnectionStrategy(ch.getConnectionStrategy());
this.setConnectionRetryExceptions(ch.getConnectionRetryExceptions());
this.setConnectionCount(ch.getConnectionCount());
this.setStopTlsOnClose(ch.getStopTlsOnClose());
}
/**
* Returns whether to call {@link StartTlsResponse#close()} when {@link
* #close()} is called.
*
* @return stop TLS on close
*/
public boolean getStopTlsOnClose()
{
return this.stopTlsOnClose;
}
/**
* Sets whether to call {@link StartTlsResponse#close()} when {@link #close()}
* is called.
*
* @param b stop TLS on close
*/
public void setStopTlsOnClose(final boolean b)
{
if (this.logger.isTraceEnabled()) {
this.logger.trace("setting stopTlsOnClose: " + b);
}
this.stopTlsOnClose = b;
}
/**
* This returns the startTLS response created by a call to {@link
* #connect(String, Object)}.
*
* @return start tls response
*/
public StartTlsResponse getStartTlsResponse()
{
return this.startTlsResponse;
}
/** {@inheritDoc} */
protected void connectInternal(
final String authtype,
final String dn,
final Object credential,
final Hashtable env)
throws NamingException
{
if (this.logger.isDebugEnabled()) {
this.logger.debug("Bind with the following parameters:");
this.logger.debug(" authtype = " + authtype);
this.logger.debug(" dn = " + dn);
if (this.config.getLogCredentials()) {
if (this.logger.isDebugEnabled()) {
this.logger.debug(" credential = " + credential);
}
} else {
if (this.logger.isDebugEnabled()) {
this.logger.debug(" credential = ");
}
}
if (this.logger.isTraceEnabled()) {
this.logger.trace(" env = " + env);
}
}
env.put(LdapConstants.VERSION, LdapConstants.VERSION_THREE);
try {
this.context = new InitialLdapContext(env, null);
this.startTlsResponse = this.startTls(this.context);
// note that when using simple authentication (the default),
// if the credential is null the provider will automatically revert the
// authentication to none
this.context.addToEnvironment(LdapConstants.AUTHENTICATION, authtype);
if (dn != null) {
this.context.addToEnvironment(LdapConstants.PRINCIPAL, dn);
if (credential != null) {
this.context.addToEnvironment(LdapConstants.CREDENTIALS, credential);
}
}
this.context.reconnect(null);
} catch (NamingException e) {
if (this.context != null) {
try {
this.context.close();
} finally {
this.context = null;
}
}
throw e;
}
}
/** {@inheritDoc} */
public void close()
throws NamingException
{
try {
if (this.stopTlsOnClose) {
this.stopTls(this.startTlsResponse);
}
} catch (NamingException e) {
if (this.logger.isErrorEnabled()) {
this.logger.error("Error stopping TLS", e);
}
} finally {
this.startTlsResponse = null;
super.close();
}
}
/**
* This will attempt to StartTLS with the supplied LdapContext
.
*
* @param ctx LdapContext
*
* @return StartTlsResponse
*
* @throws NamingException if an error occurs while requesting an extended
* operation
*/
public StartTlsResponse startTls(final LdapContext ctx)
throws NamingException
{
StartTlsResponse tls = null;
try {
tls = (StartTlsResponse) ctx.extendedOperation(new StartTlsRequest());
if (this.config.useHostnameVerifier()) {
if (this.logger.isTraceEnabled()) {
this.logger.trace(
"TLS hostnameVerifier = " + this.config.getHostnameVerifier());
}
tls.setHostnameVerifier(this.config.getHostnameVerifier());
}
if (this.config.useSslSocketFactory()) {
if (this.logger.isTraceEnabled()) {
this.logger.trace(
"TLS sslSocketFactory = " + this.config.getSslSocketFactory());
}
tls.negotiate(this.config.getSslSocketFactory());
} else {
tls.negotiate();
}
} catch (IOException e) {
if (this.logger.isErrorEnabled()) {
this.logger.error("Could not negotiate TLS connection", e);
}
throw new CommunicationException(e.getMessage());
}
return tls;
}
/**
* This will attempt to StopTLS with the supplied
* StartTlsResponse
.
*
* @param tls StartTlsResponse
*
* @throws NamingException if an error occurs while closing the TLS
* connection
*/
public void stopTls(final StartTlsResponse tls)
throws NamingException
{
if (tls != null) {
try {
tls.close();
} catch (IOException e) {
if (this.logger.isErrorEnabled()) {
this.logger.error("Could not close TLS connection", e);
}
throw new CommunicationException(e.getMessage());
}
}
}
/** {@inheritDoc} */
public TlsConnectionHandler newInstance()
{
return new TlsConnectionHandler(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy