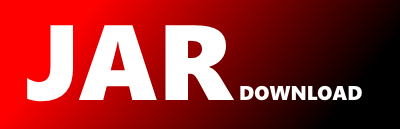
edu.vt.middleware.ldap.pool.DefaultLdapFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: DefaultLdapFactory.java 1330 2010-05-23 22:10:53Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1330 $
Updated: $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
package edu.vt.middleware.ldap.pool;
import java.io.InputStream;
import javax.naming.NamingException;
import edu.vt.middleware.ldap.Ldap;
import edu.vt.middleware.ldap.LdapConfig;
/**
* DefaultLdapFactory
provides a simple implementation of an ldap
* factory.
*
* @author Middleware Services
* @version $Revision: 1330 $ $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
public class DefaultLdapFactory extends AbstractLdapFactory
{
/** Ldap config to create ldap objects with. */
private LdapConfig config;
/** Whether to connect to the ldap on object creation. */
private boolean connectOnCreate = true;
/**
* This creates a new DefaultLdapFactory
with the default
* properties file, which must be located in your classpath.
*/
public DefaultLdapFactory()
{
this.config = LdapConfig.createFromProperties(null);
this.config.makeImmutable();
}
/**
* This creates a new DefaultLdapFactory
with the supplied input
* stream.
*
* @param is InputStream
*/
public DefaultLdapFactory(final InputStream is)
{
this.config = LdapConfig.createFromProperties(is);
this.config.makeImmutable();
}
/**
* This creates a new DefaultLdapFactory
with the supplied ldap
* configuration. The ldap configuration will be marked as immutable by this
* factory.
*
* @param lc ldap config
*/
public DefaultLdapFactory(final LdapConfig lc)
{
this.config = lc;
this.config.makeImmutable();
}
/**
* Returns whether ldap objects will attempt to connect after creation.
* Default is true.
*
* @return boolean
*/
public boolean getConnectOnCreate()
{
return this.connectOnCreate;
}
/**
* This sets whether newly created ldap objects will attempt to connect.
* Default is true.
*
* @param b connect on create
*/
public void setConnectOnCreate(final boolean b)
{
this.connectOnCreate = b;
}
/** {@inheritDoc} */
public Ldap create()
{
Ldap l = new Ldap(this.config);
if (this.connectOnCreate) {
try {
l.connect();
} catch (NamingException e) {
if (this.logger.isErrorEnabled()) {
this.logger.error("unabled to connect to the ldap", e);
}
l = null;
}
}
return l;
}
/** {@inheritDoc} */
public void destroy(final Ldap l)
{
l.close();
if (this.logger.isTraceEnabled()) {
this.logger.trace("destroyed ldap object: " + l);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy