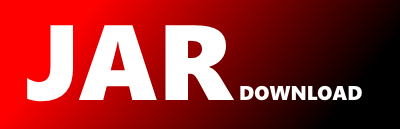
edu.vt.middleware.ldap.servlets.session.SessionManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: SessionManager.java 1330 2010-05-23 22:10:53Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1330 $
Updated: $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
package edu.vt.middleware.ldap.servlets.session;
import javax.servlet.ServletException;
import javax.servlet.http.HttpSession;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* SessionManager
provides a parent class for initializing a
* HttpSession
after a successful authentication and destroying a
* HttpSession
after logout.
*
* @author Middleware Services
* @version $Revision: 1330 $ $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
public abstract class SessionManager
{
/** Log for this class. */
protected final Log logger = LogFactory.getLog(this.getClass());
/** Identifier to set in the session after valid authentication. */
protected String sessionId;
/** Whether to invalidate session on logout. */
protected boolean invalidateSession = true;
/**
* This sets a session id that can be used in {@link #login} or {@link
* #logout}.
*
* @param id String
*/
public void setSessionId(final String id)
{
this.sessionId = id;
if (this.logger.isDebugEnabled()) {
this.logger.debug("Set session attribute to " + this.sessionId);
}
}
/**
* This sets whether to invalidate a session on logout. Default value is true.
*
* @param invalidate boolean
*/
public void setInvalidateSession(final boolean invalidate)
{
this.invalidateSession = invalidate;
if (this.logger.isDebugEnabled()) {
this.logger.debug("Set invalidateSession to " + this.invalidateSession);
}
}
/**
* This performs any actions necessary to login the suppled user.
*
* @param session HttpSession
* @param user String
*
* @throws ServletException if an error occurs initializing the session
*/
public abstract void login(HttpSession session, String user)
throws ServletException;
/**
* This performs any actions necessary to logout the suppled session.
*
* @param session HttpSession
*
* @throws ServletException if an error occurs cleaning up the session
*/
public abstract void logout(HttpSession session)
throws ServletException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy