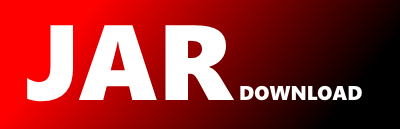
edu.vt.middleware.ldap.LdapSearch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: LdapSearch.java 1330 2010-05-23 22:10:53Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1330 $
Updated: $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
package edu.vt.middleware.ldap;
import java.io.IOException;
import java.io.Writer;
import java.util.Iterator;
import javax.naming.NamingException;
import javax.naming.directory.SearchResult;
import edu.vt.middleware.ldap.bean.LdapBeanFactory;
import edu.vt.middleware.ldap.bean.LdapBeanProvider;
import edu.vt.middleware.ldap.bean.LdapResult;
import edu.vt.middleware.ldap.pool.LdapPool;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* LdapSearch
queries an LDAP and returns the result. Each instance
* of LdapSearch
maintains it's own pool of LDAP connections.
*
* @author Middleware Services
* @version $Revision: 1330 $ $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
public class LdapSearch
{
/** Log for this class. */
protected final Log logger = LogFactory.getLog(this.getClass());
/** Ldap object to use for searching. */
protected LdapPool pool;
/** Ldap bean factory. */
protected LdapBeanFactory beanFactory = LdapBeanProvider.getLdapBeanFactory();
/**
* This creates a new LdapSearch
with the supplied pool.
*
* @param pool LdapPool
*/
public LdapSearch(final LdapPool pool)
{
this.pool = pool;
}
/**
* Returns the factory for creating ldap beans.
*
* @return LdapBeanFactory
*/
public LdapBeanFactory getLdapBeanFactory()
{
return this.beanFactory;
}
/**
* Sets the factory for creating ldap beans.
*
* @param lbf LdapBeanFactory
*/
public void setLdapBeanFactory(final LdapBeanFactory lbf)
{
if (lbf != null) {
this.beanFactory = lbf;
}
}
/**
* This will perform an LDAP search with the supplied query and return
* attributes.
*
* @param query String
to search for
* @param attrs String[]
to return
*
* @return Iterator
of search results
*
* @throws NamingException if an error occurs while searching
*/
public Iterator search(final String query, final String[] attrs)
throws NamingException
{
Iterator queryResults = null;
if (query != null) {
try {
Ldap ldap = null;
try {
ldap = this.pool.checkOut();
queryResults = ldap.search(new SearchFilter(query), attrs);
} catch (NamingException e) {
if (this.logger.isErrorEnabled()) {
this.logger.error("Error attempting LDAP search", e);
}
throw e;
} finally {
this.pool.checkIn(ldap);
}
} catch (Exception e) {
if (this.logger.isErrorEnabled()) {
this.logger.error("Error using LDAP pool", e);
}
}
}
return queryResults;
}
/**
* This will perform an LDAP search with the supplied query and return
* attributes. The results will be written to the supplied
* Writer
.
*
* @param query String
to search for
* @param attrs String[]
to return
* @param writer Writer
to write to
*
* @throws NamingException if an error occurs while searching
* @throws IOException if an error occurs while writing search results
*/
public void search(
final String query,
final String[] attrs,
final Writer writer)
throws NamingException, IOException
{
final LdapResult lr = this.beanFactory.newLdapResult();
lr.addEntries(this.search(query, attrs));
writer.write(lr.toString());
writer.flush();
}
/**
* Empties the underlying ldap pool, closing all connections. See {@link
* LdapPool#close()}.
*/
public void close()
{
this.pool.close();
}
/**
* Called by the garbage collector on an object when garbage collection
* determines that there are no more references to the object.
*
* @throws Throwable if an exception is thrown by this method
*/
protected void finalize()
throws Throwable
{
try {
this.close();
} finally {
super.finalize();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy