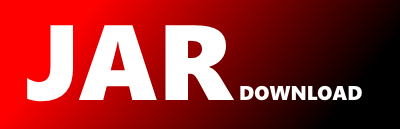
edu.vt.middleware.ldap.auth.ConstructDnResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: ConstructDnResolver.java 1632 2010-09-28 22:42:24Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1632 $
Updated: $Date: 2010-09-28 18:42:24 -0400 (Tue, 28 Sep 2010) $
*/
package edu.vt.middleware.ldap.auth;
import java.io.Serializable;
import javax.naming.NamingException;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* ConstructDnResolver
creates an LDAP DN using known information
* about the LDAP. Specifically it concatenates the first user field with the
* base DN.
*
* @author Middleware Services
* @version $Revision: 1632 $ $Date: 2010-09-28 18:42:24 -0400 (Tue, 28 Sep 2010) $
*/
public class ConstructDnResolver implements DnResolver, Serializable
{
/** serial version uid. */
private static final long serialVersionUID = -6508789359608064771L;
/** Log for this class. */
protected final Log logger = LogFactory.getLog(this.getClass());
/** Authentication configuration. */
protected AuthenticatorConfig config;
/** Default constructor. */
public ConstructDnResolver() {}
/**
* This will create a new ConstructDnResolver
with the supplied
* AuthenticatorConfig
.
*
* @param authConfig AuthenticatorConfig
*/
public ConstructDnResolver(final AuthenticatorConfig authConfig)
{
this.setAuthenticatorConfig(authConfig);
}
/**
* This will set the config parameters of this Authenticator
.
*
* @param authConfig AuthenticatorConfig
*/
public void setAuthenticatorConfig(final AuthenticatorConfig authConfig)
{
this.config = authConfig;
}
/**
* This returns the AuthenticatorConfig
of the
* Authenticator
.
*
* @return AuthenticatorConfig
*/
public AuthenticatorConfig getAuthenticatorConfig()
{
return this.config;
}
/**
* Creates a LDAP DN by combining the userField and the base dn.
*
* @param user String
to find dn for
*
* @return String
- user's dn
*
* @throws NamingException if the LDAP search fails
*/
public String resolve(final String user)
throws NamingException
{
String dn = null;
if (user != null && !"".equals(user)) {
if (this.logger.isDebugEnabled()) {
this.logger.debug("Constructing DN from first userfield and base");
}
dn = String.format(
"%s=%s,%s",
this.config.getUserField()[0],
user,
this.config.getBaseDn());
} else {
if (this.logger.isDebugEnabled()) {
this.logger.debug("User input was empty or null");
}
}
return dn;
}
/** {@inheritDoc} */
public void close() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy