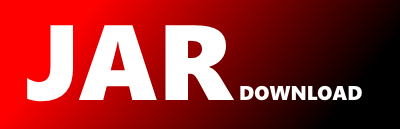
edu.vt.middleware.ldap.auth.SearchDnResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: SearchDnResolver.java 1634 2010-09-29 20:03:09Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1634 $
Updated: $Date: 2010-09-29 16:03:09 -0400 (Wed, 29 Sep 2010) $
*/
package edu.vt.middleware.ldap.auth;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import javax.naming.NamingException;
import javax.naming.directory.SearchResult;
import edu.vt.middleware.ldap.AbstractLdap;
import edu.vt.middleware.ldap.SearchFilter;
/**
* SearchDnResolver
looks up a user's DN using an LDAP search.
*
* @author Middleware Services
* @version $Revision: 1634 $ $Date: 2010-09-29 16:03:09 -0400 (Wed, 29 Sep 2010) $
*/
public class SearchDnResolver extends AbstractLdap
implements DnResolver, Serializable
{
/** serial version uid. */
private static final long serialVersionUID = -7615995272176088807L;
/** Default constructor. */
public SearchDnResolver() {}
/**
* This will create a new SearchDnResolver
with the supplied
* AuthenticatorConfig
.
*
* @param authConfig AuthenticatorConfig
*/
public SearchDnResolver(final AuthenticatorConfig authConfig)
{
this.setAuthenticatorConfig(authConfig);
}
/**
* This will set the config parameters of this Authenticator
.
*
* @param authConfig AuthenticatorConfig
*/
public void setAuthenticatorConfig(final AuthenticatorConfig authConfig)
{
super.setLdapConfig(authConfig);
}
/**
* This returns the AuthenticatorConfig
of the
* Authenticator
.
*
* @return AuthenticatorConfig
*/
public AuthenticatorConfig getAuthenticatorConfig()
{
return this.config;
}
/**
* This will attempt to find the dn for the supplied user. {@link
* AuthenticatorConfig#getUserFilter()} or {@link
* AuthenticatorConfig#getUserField()} is used to look up the dn. If a filter
* is used, the user is provided as the {0} variable filter argument. If a
* field is used, the filter is built by ORing the fields together. If more
* than one entry matches the search, the result is controlled by {@link
* AuthenticatorConfig#setAllowMultipleDns(boolean)}.
*
* @param user String
to find dn for
*
* @return String
- user's dn
*
* @throws NamingException if the LDAP search fails
*/
public String resolve(final String user)
throws NamingException
{
String dn = null;
if (user != null && !"".equals(user)) {
// create the search filter
final SearchFilter filter = new SearchFilter();
if (this.config.getUserFilter() != null) {
if (this.logger.isDebugEnabled()) {
this.logger.debug("Looking up DN using userFilter");
}
filter.setFilter(this.config.getUserFilter());
filter.setFilterArgs(this.config.getUserFilterArgs());
} else {
if (this.logger.isDebugEnabled()) {
this.logger.debug("Looking up DN using userField");
}
if (
this.config.getUserField() == null ||
this.config.getUserField().length == 0) {
if (this.logger.isErrorEnabled()) {
this.logger.error("Invalid userField, cannot be null or empty.");
}
} else {
final StringBuffer searchFilter = new StringBuffer();
if (this.config.getUserField().length > 1) {
searchFilter.append("(|");
for (int i = 0; i < this.config.getUserField().length; i++) {
searchFilter.append("(").append(this.config.getUserField()[i])
.append("={0})");
}
searchFilter.append(")");
} else {
searchFilter.append("(").append(this.config.getUserField()[0])
.append("={0})");
}
filter.setFilter(searchFilter.toString());
}
}
if (filter.getFilter() != null) {
// make user the first filter arg
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy