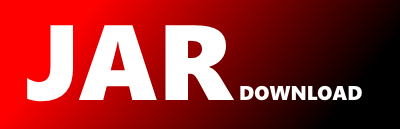
edu.vt.middleware.ldap.bean.AbstractLdapAttributes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: AbstractLdapAttributes.java 1330 2010-05-23 22:10:53Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1330 $
Updated: $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
package edu.vt.middleware.ldap.bean;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import javax.naming.NamingEnumeration;
import javax.naming.NamingException;
import javax.naming.directory.Attribute;
import javax.naming.directory.Attributes;
import javax.naming.directory.BasicAttributes;
/**
* AbstractLdapAttributes
provides a base implementation of
* LdapAttributes
where the underlying attributes are backed by a
* Map
.
*
* @param type of backing map
*
* @author Middleware Services
* @version $Revision: 1330 $ $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
public abstract class
AbstractLdapAttributes>
extends AbstractLdapBean implements LdapAttributes
{
/** Whether to ignore case when creating BasicAttributes
. */
public static final boolean DEFAULT_IGNORE_CASE = true;
/** hash code seed. */
protected static final int HASH_CODE_SEED = 42;
/** Attributes contained in this bean. */
protected T attributes;
/**
* Creates a new AbstractLdapAttributes
with the supplied ldap
* bean factory.
*
* @param lbf LdapBeanFactory
*/
public AbstractLdapAttributes(final LdapBeanFactory lbf)
{
super(lbf);
}
/** {@inheritDoc} */
public Collection getAttributes()
{
return this.attributes.values();
}
/** {@inheritDoc} */
public LdapAttribute getAttribute(final String name)
{
return this.attributes.get(name);
}
/** {@inheritDoc} */
public String[] getAttributeNames()
{
return this.attributes.keySet().toArray(new String[0]);
}
/** {@inheritDoc} */
public void addAttribute(final LdapAttribute a)
{
this.attributes.put(a.getName(), a);
}
/** {@inheritDoc} */
public void addAttribute(final String name, final Object value)
{
final LdapAttribute la = this.beanFactory.newLdapAttribute();
la.setName(name);
la.getValues().add(value);
this.addAttribute(la);
}
/** {@inheritDoc} */
public void addAttribute(final String name, final List> values)
{
final LdapAttribute la = this.beanFactory.newLdapAttribute();
la.setName(name);
la.getValues().addAll(values);
this.addAttribute(la);
}
/** {@inheritDoc} */
public void addAttributes(final Collection c)
{
for (LdapAttribute la : c) {
this.addAttribute(la);
}
}
/** {@inheritDoc} */
public void addAttributes(final Attributes a)
throws NamingException
{
final NamingEnumeration extends Attribute> ne = a.getAll();
while (ne.hasMore()) {
final LdapAttribute la = this.beanFactory.newLdapAttribute();
la.setAttribute(ne.next());
this.addAttribute(la);
}
}
/** {@inheritDoc} */
public void removeAttribute(final LdapAttribute a)
{
this.attributes.remove(a.getName());
}
/** {@inheritDoc} */
public void removeAttribute(final String name)
{
this.attributes.remove(name);
}
/** {@inheritDoc} */
public void removeAttributes(final Collection c)
{
for (LdapAttribute la : c) {
this.removeAttribute(la);
}
}
/** {@inheritDoc} */
public void removeAttributes(final Attributes a)
throws NamingException
{
final NamingEnumeration extends Attribute> ne = a.getAll();
while (ne.hasMore()) {
final LdapAttribute la = this.beanFactory.newLdapAttribute();
la.setAttribute(ne.next());
this.removeAttribute(la);
}
}
/** {@inheritDoc} */
public int size()
{
return this.attributes.size();
}
/** {@inheritDoc} */
public void clear()
{
this.attributes.clear();
}
/** {@inheritDoc} */
public int hashCode()
{
int hc = HASH_CODE_SEED;
for (LdapAttribute a : this.attributes.values()) {
if (a != null) {
hc += a.hashCode();
}
}
return hc;
}
/**
* This returns a string representation of this object.
*
* @return String
*/
@Override
public String toString()
{
return String.format("%s", this.attributes.values());
}
/** {@inheritDoc} */
public Attributes toAttributes()
{
final Attributes attributes = new BasicAttributes(DEFAULT_IGNORE_CASE);
for (LdapAttribute a : this.attributes.values()) {
attributes.put(a.toAttribute());
}
return attributes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy