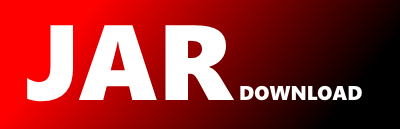
edu.vt.middleware.ldap.handler.ConnectionHandler Maven / Gradle / Ivy
/*
$Id: ConnectionHandler.java 1616 2010-09-21 17:22:27Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1616 $
Updated: $Date: 2010-09-21 13:22:27 -0400 (Tue, 21 Sep 2010) $
*/
package edu.vt.middleware.ldap.handler;
import javax.naming.NamingException;
import javax.naming.ldap.LdapContext;
import edu.vt.middleware.ldap.LdapConfig;
/**
* ConnectionHandler provides an interface for creating and closing LDAP
* connections.
*
* @author Middleware Services
* @version $Revision: 1616 $
*/
public interface ConnectionHandler
{
/** Enum to define the type of connection strategy. */
public enum ConnectionStrategy {
/** default strategy. */
DEFAULT,
/** active-passive strategy. */
ACTIVE_PASSIVE,
/** round robin strategy. */
ROUND_ROBIN,
/** random strategy. */
RANDOM,
}
/**
* Returns the connection strategy.
*
* @return strategy for making connections
*/
ConnectionStrategy getConnectionStrategy();
/**
* Sets the connection strategy.
*
* @param strategy for making connections
*/
void setConnectionStrategy(ConnectionStrategy strategy);
/**
* This returns the exception types to retry connections on.
*
* @return Class[]
*/
Class>[] getConnectionRetryExceptions();
/**
* This sets the exception types to retry connections on.
*
* @param exceptions Class[]
*/
void setConnectionRetryExceptions(Class>[] exceptions);
/**
* Returns the ldap configuration.
*
* @return ldap config
*/
LdapConfig getLdapConfig();
/**
* Sets the ldap configuration.
*
* @param lc ldap config
*/
void setLdapConfig(LdapConfig lc);
/**
* Open a connection to an LDAP.
*
* @param dn to attempt bind with
* @param credential to attempt bind with
*
* @throws NamingException if an LDAP error occurs
*/
void connect(String dn, Object credential)
throws NamingException;
/**
* Returns whether the underlying context has been established.
*
* @return whether a connection has been made
*/
boolean isConnected();
/**
* Returns an ldap context to use for ldap operations. {@link #connect(String,
* Object)} must be called prior to invoking this.
*
* @return ldap context
*
* @throws NamingException if an LDAP error occurs
*/
LdapContext getLdapContext()
throws NamingException;
/**
* Close a connection to an LDAP.
*
* @throws NamingException if an LDAP error occurs
*/
void close()
throws NamingException;
/**
* Returns a separate instance of this connection handler with the same
* underlying ldap configuration.
*
* @return connection handler
*/
ConnectionHandler newInstance();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy