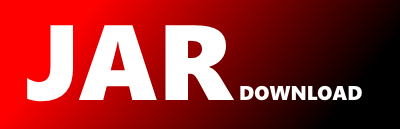
edu.vt.middleware.ldap.handler.SearchCriteria Maven / Gradle / Ivy
/*
$Id: SearchCriteria.java 1330 2010-05-23 22:10:53Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1330 $
Updated: $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
package edu.vt.middleware.ldap.handler;
import javax.naming.directory.Attributes;
/**
* SearchCriteria
contains the attributes used to perform ldap
* searches.
*
* @author Middleware Services
* @version $Revision: 1330 $ $Date: 2010-05-23 18:10:53 -0400 (Sun, 23 May 2010) $
*/
public class SearchCriteria
{
/** dn. */
private String dn;
/** filter. */
private String filter;
/** filter arguments. */
private Object[] filterArgs;
/** return attributes. */
private String[] returnAttrs;
/** match attributes. */
private Attributes matchAttrs;
/** Default constructor. */
public SearchCriteria() {}
/**
* Creates a new search criteria with the supplied dn.
*
* @param s to set dn
*/
public SearchCriteria(final String s)
{
this.dn = s;
}
/**
* Gets the dn.
*
* @return dn
*/
public String getDn()
{
return this.dn;
}
/**
* Sets the dn.
*
* @param s to set dn
*/
public void setDn(final String s)
{
this.dn = s;
}
/**
* Gets the filter.
*
* @return filter
*/
public String getFilter()
{
return this.filter;
}
/**
* Sets the filter.
*
* @param s to set filter
*/
public void setFilter(final String s)
{
this.filter = s;
}
/**
* Gets the filter arguments.
*
* @return filter args
*/
public Object[] getFilterArgs()
{
return this.filterArgs;
}
/**
* Sets the filter arguments.
*
* @param o to set filter argumets
*/
public void setFilterArgs(final Object[] o)
{
this.filterArgs = o;
}
/**
* Gets the return attributes.
*
* @return return attributes
*/
public String[] getReturnAttrs()
{
return this.returnAttrs;
}
/**
* Sets the return attributes.
*
* @param s to set return attributes
*/
public void setReturnAttrs(final String[] s)
{
this.returnAttrs = s;
}
/**
* Gets the match attributes.
*
* @return match attributes
*/
public Attributes getMatchAttrs()
{
return this.matchAttrs;
}
/**
* Sets the match attributes.
*
* @param a to set match attributes
*/
public void setMatchAttrs(final Attributes a)
{
this.matchAttrs = a;
}
/**
* This returns a string representation of this search criteria.
*
* @return String
*/
@Override
public String toString()
{
return
String.format(
"dn=%s,filter=%s,filterArgs=%s,returnAttrs=%s,matchAttrs=%s",
this.dn,
this.filter,
this.filterArgs,
this.returnAttrs,
this.matchAttrs);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy