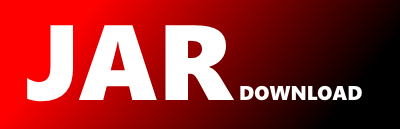
edu.vt.middleware.ldap.props.SimplePropertyInvoker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: SimplePropertyInvoker.java 1441 2010-07-01 16:55:43Z dfisher $
Copyright (C) 2003-2010 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 1441 $
Updated: $Date: 2010-07-01 12:55:43 -0400 (Thu, 01 Jul 2010) $
*/
package edu.vt.middleware.ldap.props;
import java.lang.reflect.Array;
/**
* SimplePropertyInvoker
stores setter methods for a class to make
* method invocation of simple properties easier.
*
* @author Middleware Services
* @version $Revision: 1441 $ $Date: 2010-07-01 12:55:43 -0400 (Thu, 01 Jul 2010) $
*/
public class SimplePropertyInvoker extends AbstractPropertyInvoker
{
/**
* Creates a new SimplePropertyInvoker
for the supplied class.
*
* @param c Class
that has setter methods
*/
public SimplePropertyInvoker(final Class> c)
{
this.initialize(c, "");
}
/** {@inheritDoc} */
protected Object convertValue(final Class> type, final String value)
{
Object newValue = value;
if (type != String.class) {
if (Class.class.isAssignableFrom(type)) {
if ("null".equals(value)) {
newValue = null;
} else {
newValue = createClass(value);
}
} else if (Class[].class.isAssignableFrom(type)) {
if ("null".equals(value)) {
newValue = null;
} else {
final String[] classes = value.split(",");
newValue = Array.newInstance(Class.class, classes.length);
for (int i = 0; i < classes.length; i++) {
Array.set(newValue, i, createClass(classes[i]));
}
}
} else if (type.isEnum()) {
for (Object o : type.getEnumConstants()) {
final Enum> e = (Enum>) o;
if (e.name().equals(value)) {
newValue = o;
}
}
} else if (String[].class == type) {
newValue = value.split(",");
} else if (Object[].class == type) {
newValue = value.split(",");
} else if (float.class == type) {
newValue = Float.parseFloat(value);
} else if (int.class == type) {
newValue = Integer.parseInt(value);
} else if (long.class == type) {
newValue = Long.parseLong(value);
} else if (short.class == type) {
newValue = Short.parseShort(value);
} else if (double.class == type) {
newValue = Double.parseDouble(value);
} else if (boolean.class == type) {
newValue = Boolean.valueOf(value);
}
}
return newValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy