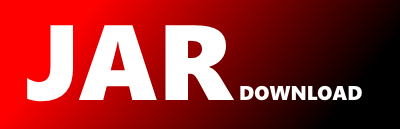
edu.vt.middleware.ldap.ssl.AggregateTrustManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: AggregateTrustManager.java 2231 2012-02-02 15:46:27Z dfisher $
Copyright (C) 2003-2012 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 2231 $
Updated: $Date: 2012-02-02 10:46:27 -0500 (Thu, 02 Feb 2012) $
*/
package edu.vt.middleware.ldap.ssl;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import java.util.ArrayList;
import java.util.List;
import javax.net.ssl.X509TrustManager;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* Trust manager that delegates to multiple trust managers.
*
* @author Middleware Services
* @version $Revision: 2231 $
*/
public class AggregateTrustManager implements X509TrustManager
{
/** Log for this class. */
protected final Log logger = LogFactory.getLog(this.getClass());
/** Trust managers to invoke. */
private X509TrustManager[] trustManagers;
/**
* Creates a new aggregate trust manager.
*
* @param managers to aggregate
*/
public AggregateTrustManager(final X509TrustManager... managers)
{
trustManagers = managers;
}
/** {@inheritDoc} */
public void checkClientTrusted(
final X509Certificate[] chain, final String authType)
throws CertificateException
{
if (trustManagers != null) {
for (X509TrustManager tm : trustManagers) {
if (this.logger.isDebugEnabled()) {
this.logger.debug("invoking checkClientTrusted for " + tm);
}
tm.checkClientTrusted(chain, authType);
}
}
}
/** {@inheritDoc} */
public void checkServerTrusted(
final X509Certificate[] chain, final String authType)
throws CertificateException
{
if (trustManagers != null) {
for (X509TrustManager tm : trustManagers) {
if (this.logger.isDebugEnabled()) {
this.logger.debug("invoking checkServerTrusted for " + tm);
}
tm.checkServerTrusted(chain, authType);
}
}
}
/** {@inheritDoc} */
public X509Certificate[] getAcceptedIssuers()
{
final List issuers = new ArrayList();
if (trustManagers != null) {
for (X509TrustManager tm : trustManagers) {
if (this.logger.isDebugEnabled()) {
this.logger.debug("invoking getAcceptedIssuers invoked for " + tm);
}
for (X509Certificate cert : tm.getAcceptedIssuers()) {
issuers.add(cert);
}
}
}
return issuers.toArray(new X509Certificate[issuers.size()]);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy