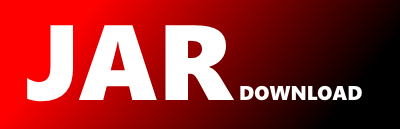
edu.vt.middleware.ldap.ssl.HostnameVerifyingTrustManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-ldap Show documentation
Show all versions of vt-ldap Show documentation
Library for performing common LDAP operations
/*
$Id: HostnameVerifyingTrustManager.java 2231 2012-02-02 15:46:27Z dfisher $
Copyright (C) 2003-2012 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 2231 $
Updated: $Date: 2012-02-02 10:46:27 -0500 (Thu, 02 Feb 2012) $
*/
package edu.vt.middleware.ldap.ssl;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import java.util.Arrays;
import javax.net.ssl.X509TrustManager;
/**
* Trust manager that delegates to {@link CertificateHostnameVerifier}. Any name
* that verifies passes this trust manager check.
*
* @author Middleware Services
* @version $Revision: 2231 $
*/
public class HostnameVerifyingTrustManager implements X509TrustManager
{
/** Hostnames to allow. */
private String[] hostnames;
/** Hostname verifier to use for trust. */
private CertificateHostnameVerifier hostnameVerifier;
/**
* Creates a new hostname verifying trust manager.
*
* @param verifier that establishes trust
* @param names to match against a certificate
*/
public HostnameVerifyingTrustManager(
final CertificateHostnameVerifier verifier,
final String... names)
{
hostnameVerifier = verifier;
hostnames = names;
}
/** {@inheritDoc} */
public void checkClientTrusted(
final X509Certificate[] chain, final String authType)
throws CertificateException
{
checkCertificateTrusted(chain[0]);
}
/** {@inheritDoc} */
public void checkServerTrusted(
final X509Certificate[] chain, final String authType)
throws CertificateException
{
checkCertificateTrusted(chain[0]);
}
/**
* Verifies the supplied certificate using the hostname verifier with each
* hostname.
*
* @param cert to verify
*
* @throws CertificateException if none of the hostnames verify
*/
private void checkCertificateTrusted(final X509Certificate cert)
throws CertificateException
{
for (String name : hostnames) {
if (hostnameVerifier.verify(name, cert)) {
return;
}
}
throw new CertificateException(
String.format(
"Hostname '%s' does not match the hostname in the server's " +
"certificate", Arrays.toString(hostnames)));
}
/** {@inheritDoc} */
public X509Certificate[] getAcceptedIssuers()
{
return new X509Certificate[0];
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy