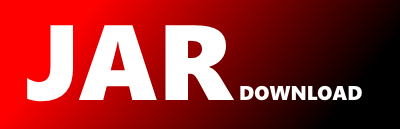
edu.vt.middleware.password.PasswordCli Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-password Show documentation
Show all versions of vt-password Show documentation
Library for checking that a password complies with a custom set of rules
The newest version!
/*
$Id: PasswordCli.java 2704 2013-04-24 21:30:32Z dfisher $
Copyright (C) 2003-2013 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 2704 $
Updated: $Date: 2013-04-24 17:30:32 -0400 (Wed, 24 Apr 2013) $
*/
package edu.vt.middleware.password;
import java.io.RandomAccessFile;
import java.util.ArrayList;
import java.util.List;
import edu.vt.middleware.dictionary.FileWordList;
import edu.vt.middleware.dictionary.TernaryTreeDictionary;
/**
* Provides a simple command line interface to password validation.
*
* @author Middleware Services
* @version $Revision: 2704 $ $Date: 2013-04-24 17:30:32 -0400 (Wed, 24 Apr 2013) $
*/
public final class PasswordCli
{
/** Default constructor. */
private PasswordCli() {}
/**
* Provides command line access to password rules.
*
* @param args from command line
*
* @throws Exception if an error occurs
*/
public static void main(final String[] args)
throws Exception
{
final List rules = new ArrayList();
String username = null;
String password = null;
try {
if (args.length < 2) {
throw new ArrayIndexOutOfBoundsException();
}
for (int i = 0; i < args.length; i++) {
if ("-l".equals(args[i])) {
final int min = Integer.parseInt(args[++i]);
final int max = Integer.parseInt(args[++i]);
final LengthRule rule = new LengthRule(min, max);
rules.add(rule);
} else if ("-c".equals(args[i])) {
final CharacterCharacteristicsRule rule =
new CharacterCharacteristicsRule();
rule.getRules().add(
new DigitCharacterRule(Integer.parseInt(args[++i])));
rule.getRules().add(
new AlphabeticalCharacterRule(Integer.parseInt(args[++i])));
rule.getRules().add(
new NonAlphanumericCharacterRule(Integer.parseInt(args[++i])));
rule.getRules().add(
new UppercaseCharacterRule(Integer.parseInt(args[++i])));
rule.getRules().add(
new LowercaseCharacterRule(Integer.parseInt(args[++i])));
rule.setNumberOfCharacteristics(Integer.parseInt(args[++i]));
rules.add(rule);
} else if ("-d".equals(args[i])) {
final TernaryTreeDictionary dict = new TernaryTreeDictionary(
new FileWordList(new RandomAccessFile(args[++i], "r"), false));
final DictionarySubstringRule rule = new DictionarySubstringRule(
dict);
rule.setMatchBackwards(true);
rule.setWordLength(Integer.parseInt(args[++i]));
rules.add(rule);
} else if ("-u".equals(args[i])) {
rules.add(new UsernameRule(true, true));
username = args[++i];
} else if ("-s".equals(args[i])) {
rules.add(new QwertySequenceRule());
rules.add(new AlphabeticalSequenceRule());
rules.add(new NumericalSequenceRule());
rules.add(new RepeatCharacterRegexRule());
} else if ("-h".equals(args[i])) {
throw new ArrayIndexOutOfBoundsException();
} else {
password = args[i];
}
}
if (password == null) {
throw new ArrayIndexOutOfBoundsException();
} else {
RuleResult result;
final PasswordData pd = new PasswordData(new Password(password));
final PasswordValidator validator = new PasswordValidator(rules);
if (username != null) {
pd.setUsername(username);
}
result = validator.validate(pd);
if (result.isValid()) {
System.out.println("Valid password");
} else {
for (String s : validator.getMessages(result)) {
System.out.println(s);
}
}
}
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println(
"Usage: java " + PasswordCli.class.getName() +
" \\");
System.out.println("");
System.out.println("where includes:");
System.out.println(" -l (Set the min & max password length) \\");
System.out.println(" \\");
System.out.println(" \\");
System.out.println(
" -c (Set the characters which must be present" +
" in the password) \\");
System.out.println(" (Each of the following must be >= 0) \\");
System.out.println(" \\");
System.out.println(" \\");
System.out.println(" \\");
System.out.println(" \\");
System.out.println(" \\");
System.out.println(
" (Number of these rules to" +
" enforce) \\");
System.out.println(" -d (Test password against a dictionary) \\");
System.out.println(" (dictionary files) \\");
System.out.println(
" (number of characters in matching" +
" words) \\");
System.out.println(" -u (Test for a user id) \\");
System.out.println(" \\");
System.out.println(" -s (Test for sequences) \\");
System.out.println(" -h (Print this message) \\");
System.exit(1);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy