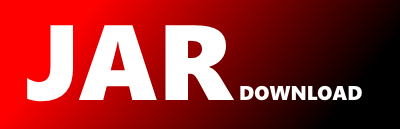
edu.vt.middleware.password.RegexRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vt-password Show documentation
Show all versions of vt-password Show documentation
Library for checking that a password complies with a custom set of rules
The newest version!
/*
$Id: RegexRule.java 2704 2013-04-24 21:30:32Z dfisher $
Copyright (C) 2003-2013 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 2704 $
Updated: $Date: 2013-04-24 17:30:32 -0400 (Wed, 24 Apr 2013) $
*/
package edu.vt.middleware.password;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Rule for determining if a password matches a regular expression.
*
* @author Middleware Services
* @version $Revision: 2704 $ $Date: 2013-04-24 17:30:32 -0400 (Wed, 24 Apr 2013) $
*/
public class RegexRule implements Rule
{
/** Error code for regex validation failures. */
public static final String ERROR_CODE = "ILLEGAL_MATCH";
/** Regex pattern. */
protected final Pattern pattern;
/**
* Creates a new regex rule.
*
* @param regex regular expression
*/
public RegexRule(final String regex)
{
pattern = Pattern.compile(regex);
}
/** {@inheritDoc} */
@Override
public RuleResult validate(final PasswordData passwordData)
{
final RuleResult result = new RuleResult(true);
final Matcher m = pattern.matcher(passwordData.getPassword().getText());
if (m.find()) {
result.setValid(false);
result.getDetails().add(
new RuleResultDetail(
ERROR_CODE,
createRuleResultDetailParameters(m.group())));
}
return result;
}
/**
* Creates the parameter data for the rule result detail.
*
* @param match matching regex
*
* @return map of parameter name to value
*/
protected Map createRuleResultDetailParameters(final String match)
{
final Map m = new LinkedHashMap();
m.put("match", match);
m.put("pattern", pattern);
return m;
}
/** {@inheritDoc} */
@Override
public String toString()
{
return
String.format(
"%s@%h::pattern=%s",
getClass().getName(),
hashCode(),
pattern);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy