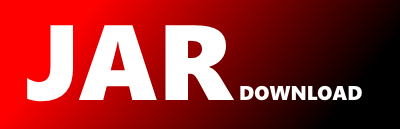
ee.xtee6.ads.aadrmuud.ADSaadrmuudatused Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtee6-client-ads Show documentation
Show all versions of xtee6-client-ads Show documentation
Library for XROAD ADS service clints
package ee.xtee6.ads.aadrmuud;
import java.io.Serializable;
import java.time.LocalDate;
import ee.datel.client.utils.AdapterForBoolean;
import ee.datel.client.utils.AdapterForInteger;
import ee.datel.client.utils.AdapterForLocalDate;
import ee.datel.client.utils.AdapterForLong;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"muudetudAlates",
"muudetudPaevad",
"logId",
"maxarv",
"pSyndmused",
"sSyndmused",
"nSyndmused",
"aSyndmused",
"oSyndmused",
"tSyndmused",
"objekt",
"seosed",
"aadressKomp",
"aadressJarglased",
"liidestujaObjektid"
})
@XmlRootElement(name = "ADSaadrmuudatused", namespace = "http://www.maaamet.ee")
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:08+02:00")
public class ADSaadrmuudatused
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDate.class)
@XmlSchemaType(name = "date")
protected LocalDate muudetudAlates;
@XmlElement(type = String.class, defaultValue = "1")
@XmlJavaTypeAdapter(AdapterForInteger.class)
protected Integer muudetudPaevad;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long logId;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
protected Long maxarv;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean pSyndmused;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean sSyndmused;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean nSyndmused;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean aSyndmused;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean oSyndmused;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean tSyndmused;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean objekt;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean seosed;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean aadressKomp;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean aadressJarglased;
@XmlElement(type = String.class, defaultValue = "false")
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean liidestujaObjektid;
/**
* Gets the value of the muudetudAlates property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getMuudetudAlates() {
return muudetudAlates;
}
/**
* Sets the value of the muudetudAlates property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMuudetudAlates(LocalDate value) {
this.muudetudAlates = value;
}
/**
* Gets the value of the muudetudPaevad property.
*
* @return
* possible object is
* {@link String }
*
*/
public Integer getMuudetudPaevad() {
return muudetudPaevad;
}
/**
* Sets the value of the muudetudPaevad property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMuudetudPaevad(Integer value) {
this.muudetudPaevad = value;
}
/**
* Gets the value of the logId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getLogId() {
return logId;
}
/**
* Sets the value of the logId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLogId(Long value) {
this.logId = value;
}
/**
* Gets the value of the maxarv property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getMaxarv() {
return maxarv;
}
/**
* Sets the value of the maxarv property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMaxarv(Long value) {
this.maxarv = value;
}
/**
* Gets the value of the pSyndmused property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isPSyndmused() {
return pSyndmused;
}
/**
* Sets the value of the pSyndmused property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPSyndmused(Boolean value) {
this.pSyndmused = value;
}
/**
* Gets the value of the sSyndmused property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isSSyndmused() {
return sSyndmused;
}
/**
* Sets the value of the sSyndmused property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSSyndmused(Boolean value) {
this.sSyndmused = value;
}
/**
* Gets the value of the nSyndmused property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isNSyndmused() {
return nSyndmused;
}
/**
* Sets the value of the nSyndmused property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNSyndmused(Boolean value) {
this.nSyndmused = value;
}
/**
* Gets the value of the aSyndmused property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isASyndmused() {
return aSyndmused;
}
/**
* Sets the value of the aSyndmused property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setASyndmused(Boolean value) {
this.aSyndmused = value;
}
/**
* Gets the value of the oSyndmused property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isOSyndmused() {
return oSyndmused;
}
/**
* Sets the value of the oSyndmused property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOSyndmused(Boolean value) {
this.oSyndmused = value;
}
/**
* Gets the value of the tSyndmused property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isTSyndmused() {
return tSyndmused;
}
/**
* Sets the value of the tSyndmused property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTSyndmused(Boolean value) {
this.tSyndmused = value;
}
/**
* Gets the value of the objekt property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isObjekt() {
return objekt;
}
/**
* Sets the value of the objekt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setObjekt(Boolean value) {
this.objekt = value;
}
/**
* Gets the value of the seosed property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isSeosed() {
return seosed;
}
/**
* Sets the value of the seosed property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSeosed(Boolean value) {
this.seosed = value;
}
/**
* Gets the value of the aadressKomp property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isAadressKomp() {
return aadressKomp;
}
/**
* Sets the value of the aadressKomp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadressKomp(Boolean value) {
this.aadressKomp = value;
}
/**
* Gets the value of the aadressJarglased property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isAadressJarglased() {
return aadressJarglased;
}
/**
* Sets the value of the aadressJarglased property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadressJarglased(Boolean value) {
this.aadressJarglased = value;
}
/**
* Gets the value of the liidestujaObjektid property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isLiidestujaObjektid() {
return liidestujaObjektid;
}
/**
* Sets the value of the liidestujaObjektid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLiidestujaObjektid(Boolean value) {
this.liidestujaObjektid = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy