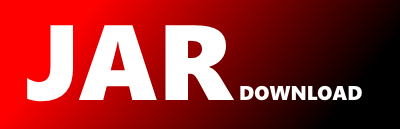
ee.xtee6.ads.normal.ADSnormalResponse Maven / Gradle / Ivy
Show all versions of xtee6-client-ads Show documentation
package ee.xtee6.ads.normal;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import ee.datel.client.utils.AdapterForDouble;
import ee.datel.client.utils.AdapterForLong;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for ADSnormalVastusType complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ADSnormalVastusType", namespace = "http://www.maaamet.ee", propOrder = {
"normalTulem",
"teade",
"taisAadress",
"optiAadress",
"lahiAadress",
"aadressid"
})
@XmlRootElement(name = "ADSnormalResponse", namespace = "http://www.maaamet.ee")
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:11+02:00")
public class ADSnormalResponse
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(required = true)
protected String normalTulem;
protected String teade;
protected String taisAadress;
protected String optiAadress;
@XmlElement(required = true)
protected String lahiAadress;
protected ADSnormalResponse.Aadressid aadressid;
/**
* Gets the value of the normalTulem property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNormalTulem() {
return normalTulem;
}
/**
* Sets the value of the normalTulem property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNormalTulem(String value) {
this.normalTulem = value;
}
/**
* Gets the value of the teade property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTeade() {
return teade;
}
/**
* Sets the value of the teade property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTeade(String value) {
this.teade = value;
}
/**
* Gets the value of the taisAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTaisAadress() {
return taisAadress;
}
/**
* Sets the value of the taisAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTaisAadress(String value) {
this.taisAadress = value;
}
/**
* Gets the value of the optiAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOptiAadress() {
return optiAadress;
}
/**
* Sets the value of the optiAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOptiAadress(String value) {
this.optiAadress = value;
}
/**
* Gets the value of the lahiAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLahiAadress() {
return lahiAadress;
}
/**
* Sets the value of the lahiAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLahiAadress(String value) {
this.lahiAadress = value;
}
/**
* Gets the value of the aadressid property.
*
* @return
* possible object is
* {@link ADSnormalResponse.Aadressid }
*
*/
public ADSnormalResponse.Aadressid getAadressid() {
return aadressid;
}
/**
* Sets the value of the aadressid property.
*
* @param value
* allowed object is
* {@link ADSnormalResponse.Aadressid }
*
*/
public void setAadressid(ADSnormalResponse.Aadressid value) {
this.aadressid = value;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"aadresses"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:11+02:00")
public static class Aadressid
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "aadress")
protected List aadresses;
/**
* Gets the value of the aadresses property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the aadresses property.
*
*
* For example, to add a new item, do as follows:
*
*
* getAadresses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ADSnormalResponse.Aadressid.Aadress }
*
*
*
* @return
* The value of the aadresses property.
*/
public List getAadresses() {
if (aadresses == null) {
aadresses = new ArrayList<>();
}
return this.aadresses;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"adsTase1",
"adsTase2",
"adsTase3",
"adsTase4",
"adsTase5",
"adsTase6",
"adsTase7",
"adsTase8",
"tekst",
"adrId",
"koodaadress",
"punktX",
"punktY"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:11+02:00")
public static class Aadress
implements Serializable
{
private static final long serialVersionUID = -1L;
protected AdsTaseType adsTase1;
protected AdsTaseType adsTase2;
protected AdsTaseType adsTase3;
protected AdsTaseType adsTase4;
protected AdsTaseType adsTase5;
protected AdsTaseType adsTase6;
protected AdsTaseType adsTase7;
protected AdsTaseType adsTase8;
protected String tekst;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long adrId;
protected String koodaadress;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double punktX;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double punktY;
/**
* Gets the value of the adsTase1 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase1() {
return adsTase1;
}
/**
* Sets the value of the adsTase1 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase1(AdsTaseType value) {
this.adsTase1 = value;
}
/**
* Gets the value of the adsTase2 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase2() {
return adsTase2;
}
/**
* Sets the value of the adsTase2 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase2(AdsTaseType value) {
this.adsTase2 = value;
}
/**
* Gets the value of the adsTase3 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase3() {
return adsTase3;
}
/**
* Sets the value of the adsTase3 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase3(AdsTaseType value) {
this.adsTase3 = value;
}
/**
* Gets the value of the adsTase4 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase4() {
return adsTase4;
}
/**
* Sets the value of the adsTase4 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase4(AdsTaseType value) {
this.adsTase4 = value;
}
/**
* Gets the value of the adsTase5 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase5() {
return adsTase5;
}
/**
* Sets the value of the adsTase5 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase5(AdsTaseType value) {
this.adsTase5 = value;
}
/**
* Gets the value of the adsTase6 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase6() {
return adsTase6;
}
/**
* Sets the value of the adsTase6 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase6(AdsTaseType value) {
this.adsTase6 = value;
}
/**
* Gets the value of the adsTase7 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase7() {
return adsTase7;
}
/**
* Sets the value of the adsTase7 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase7(AdsTaseType value) {
this.adsTase7 = value;
}
/**
* Gets the value of the adsTase8 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase8() {
return adsTase8;
}
/**
* Sets the value of the adsTase8 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase8(AdsTaseType value) {
this.adsTase8 = value;
}
/**
* Gets the value of the tekst property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTekst() {
return tekst;
}
/**
* Sets the value of the tekst property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTekst(String value) {
this.tekst = value;
}
/**
* Gets the value of the adrId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getAdrId() {
return adrId;
}
/**
* Sets the value of the adrId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdrId(Long value) {
this.adrId = value;
}
/**
* Gets the value of the koodaadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKoodaadress() {
return koodaadress;
}
/**
* Sets the value of the koodaadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKoodaadress(String value) {
this.koodaadress = value;
}
/**
* Gets the value of the punktX property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getPunktX() {
return punktX;
}
/**
* Sets the value of the punktX property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPunktX(Double value) {
this.punktX = value;
}
/**
* Gets the value of the punktY property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getPunktY() {
return punktY;
}
/**
* Sets the value of the punktY property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPunktY(Double value) {
this.punktY = value;
}
}
}
}