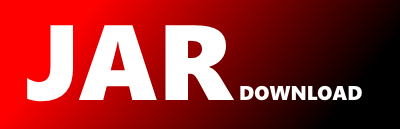
ee.xtee6.ads.objmuud.ADSobjmuudatusedV7Response Maven / Gradle / Ivy
Show all versions of xtee6-client-ads Show documentation
package ee.xtee6.ads.objmuud;
import java.io.Serializable;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
import ee.datel.client.utils.AdapterForBoolean;
import ee.datel.client.utils.AdapterForDouble;
import ee.datel.client.utils.AdapterForLocalDate;
import ee.datel.client.utils.AdapterForLocalDateTime;
import ee.datel.client.utils.AdapterForLong;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"objmuudatusedTulem",
"fault"
})
@XmlRootElement(name = "ADSobjmuudatusedV7Response", namespace = "http://www.maaamet.ee")
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public class ADSobjmuudatusedV7Response
implements Serializable
{
private static final long serialVersionUID = -1L;
protected ADSobjmuudatusedV7Response.ObjmuudatusedTulem objmuudatusedTulem;
protected ADSobjmuudatusedV7Response.Fault fault;
/**
* Gets the value of the objmuudatusedTulem property.
*
* @return
* possible object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem }
*
*/
public ADSobjmuudatusedV7Response.ObjmuudatusedTulem getObjmuudatusedTulem() {
return objmuudatusedTulem;
}
/**
* Sets the value of the objmuudatusedTulem property.
*
* @param value
* allowed object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem }
*
*/
public void setObjmuudatusedTulem(ADSobjmuudatusedV7Response.ObjmuudatusedTulem value) {
this.objmuudatusedTulem = value;
}
/**
* Gets the value of the fault property.
*
* @return
* possible object is
* {@link ADSobjmuudatusedV7Response.Fault }
*
*/
public ADSobjmuudatusedV7Response.Fault getFault() {
return fault;
}
/**
* Sets the value of the fault property.
*
* @param value
* allowed object is
* {@link ADSobjmuudatusedV7Response.Fault }
*
*/
public void setFault(ADSobjmuudatusedV7Response.Fault value) {
this.fault = value;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"faultCode",
"faultString"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class Fault
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(required = true)
protected String faultCode;
@XmlElement(required = true)
protected String faultString;
/**
* Gets the value of the faultCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFaultCode() {
return faultCode;
}
/**
* Sets the value of the faultCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFaultCode(String value) {
this.faultCode = value;
}
/**
* Gets the value of the faultString property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFaultString() {
return faultString;
}
/**
* Sets the value of the faultString property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFaultString(String value) {
this.faultString = value;
}
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"muudatuses"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class ObjmuudatusedTulem
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "muudatus", required = true)
protected List muudatuses;
/**
* Gets the value of the muudatuses property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the muudatuses property.
*
*
* For example, to add a new item, do as follows:
*
*
* getMuudatuses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus }
*
*
*
* @return
* The value of the muudatuses property.
*/
public List getMuudatuses() {
if (muudatuses == null) {
muudatuses = new ArrayList<>();
}
return this.muudatuses;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"logId",
"logStamp",
"syndmus",
"muutvektor",
"objektiLiik",
"adsOid",
"adobId",
"origTunnus",
"taisAadress",
"lahiAadress",
"olek",
"vanaAdobId",
"vanaTaisAadress",
"vanaLahiAadress",
"unikaalne",
"eellased",
"jarglased",
"hooneOID",
"kehtiv",
"kehtetu",
"hooneKujuPindala",
"hooneKorgusR",
"hooneKorgusM",
"muudatuseAlgataja",
"ruumikuju",
"kujuMoodustusviis",
"objektiPunktX",
"objektiPunktY",
"etakId",
"aadressid",
"ehRlisaandmed",
"objseosed",
"hooneosad",
"huvipunktid",
"liidestujaObjektid"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class Muudatus
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long logId;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime logStamp;
@XmlSchemaType(name = "string")
protected ObjSyndmusType syndmus;
protected String muutvektor;
@XmlElement(required = true)
@XmlSchemaType(name = "string")
protected AdsobjliikKlassifikaator objektiLiik;
@XmlElement(required = true)
protected String adsOid;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long adobId;
protected String origTunnus;
@XmlElement(required = true)
protected String taisAadress;
protected String lahiAadress;
@XmlElement(required = true)
@XmlSchemaType(name = "string")
protected OlekType olek;
protected String vanaAdobId;
protected String vanaTaisAadress;
protected String vanaLahiAadress;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean unikaalne;
protected String eellased;
protected String jarglased;
protected String hooneOID;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime kehtiv;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime kehtetu;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double hooneKujuPindala;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double hooneKorgusR;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double hooneKorgusM;
protected String muudatuseAlgataja;
protected String ruumikuju;
@XmlSchemaType(name = "string")
protected AdsObjmviisType kujuMoodustusviis;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double objektiPunktX;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double objektiPunktY;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long etakId;
protected ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Aadressid aadressid;
@XmlElement(name = "EHRlisaandmed")
protected ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.EHRlisaandmed ehRlisaandmed;
protected ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Objseosed objseosed;
protected ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Hooneosad hooneosad;
protected ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Huvipunktid huvipunktid;
protected ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.LiidestujaObjektid liidestujaObjektid;
/**
* Gets the value of the logId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getLogId() {
return logId;
}
/**
* Sets the value of the logId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLogId(Long value) {
this.logId = value;
}
/**
* Gets the value of the logStamp property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getLogStamp() {
return logStamp;
}
/**
* Sets the value of the logStamp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLogStamp(LocalDateTime value) {
this.logStamp = value;
}
/**
* Gets the value of the syndmus property.
*
* @return
* possible object is
* {@link ObjSyndmusType }
*
*/
public ObjSyndmusType getSyndmus() {
return syndmus;
}
/**
* Sets the value of the syndmus property.
*
* @param value
* allowed object is
* {@link ObjSyndmusType }
*
*/
public void setSyndmus(ObjSyndmusType value) {
this.syndmus = value;
}
/**
* Gets the value of the muutvektor property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMuutvektor() {
return muutvektor;
}
/**
* Sets the value of the muutvektor property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMuutvektor(String value) {
this.muutvektor = value;
}
/**
* Gets the value of the objektiLiik property.
*
* @return
* possible object is
* {@link AdsobjliikKlassifikaator }
*
*/
public AdsobjliikKlassifikaator getObjektiLiik() {
return objektiLiik;
}
/**
* Sets the value of the objektiLiik property.
*
* @param value
* allowed object is
* {@link AdsobjliikKlassifikaator }
*
*/
public void setObjektiLiik(AdsobjliikKlassifikaator value) {
this.objektiLiik = value;
}
/**
* Gets the value of the adsOid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAdsOid() {
return adsOid;
}
/**
* Sets the value of the adsOid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdsOid(String value) {
this.adsOid = value;
}
/**
* Gets the value of the adobId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getAdobId() {
return adobId;
}
/**
* Sets the value of the adobId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdobId(Long value) {
this.adobId = value;
}
/**
* Gets the value of the origTunnus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrigTunnus() {
return origTunnus;
}
/**
* Sets the value of the origTunnus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrigTunnus(String value) {
this.origTunnus = value;
}
/**
* Gets the value of the taisAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTaisAadress() {
return taisAadress;
}
/**
* Sets the value of the taisAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTaisAadress(String value) {
this.taisAadress = value;
}
/**
* Gets the value of the lahiAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLahiAadress() {
return lahiAadress;
}
/**
* Sets the value of the lahiAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLahiAadress(String value) {
this.lahiAadress = value;
}
/**
* Gets the value of the olek property.
*
* @return
* possible object is
* {@link OlekType }
*
*/
public OlekType getOlek() {
return olek;
}
/**
* Sets the value of the olek property.
*
* @param value
* allowed object is
* {@link OlekType }
*
*/
public void setOlek(OlekType value) {
this.olek = value;
}
/**
* Gets the value of the vanaAdobId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVanaAdobId() {
return vanaAdobId;
}
/**
* Sets the value of the vanaAdobId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVanaAdobId(String value) {
this.vanaAdobId = value;
}
/**
* Gets the value of the vanaTaisAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVanaTaisAadress() {
return vanaTaisAadress;
}
/**
* Sets the value of the vanaTaisAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVanaTaisAadress(String value) {
this.vanaTaisAadress = value;
}
/**
* Gets the value of the vanaLahiAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVanaLahiAadress() {
return vanaLahiAadress;
}
/**
* Sets the value of the vanaLahiAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVanaLahiAadress(String value) {
this.vanaLahiAadress = value;
}
/**
* Gets the value of the unikaalne property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isUnikaalne() {
return unikaalne;
}
/**
* Sets the value of the unikaalne property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUnikaalne(Boolean value) {
this.unikaalne = value;
}
/**
* Gets the value of the eellased property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEellased() {
return eellased;
}
/**
* Sets the value of the eellased property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEellased(String value) {
this.eellased = value;
}
/**
* Gets the value of the jarglased property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getJarglased() {
return jarglased;
}
/**
* Sets the value of the jarglased property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJarglased(String value) {
this.jarglased = value;
}
/**
* Gets the value of the hooneOID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHooneOID() {
return hooneOID;
}
/**
* Sets the value of the hooneOID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHooneOID(String value) {
this.hooneOID = value;
}
/**
* Gets the value of the kehtiv property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getKehtiv() {
return kehtiv;
}
/**
* Sets the value of the kehtiv property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKehtiv(LocalDateTime value) {
this.kehtiv = value;
}
/**
* Gets the value of the kehtetu property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getKehtetu() {
return kehtetu;
}
/**
* Sets the value of the kehtetu property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKehtetu(LocalDateTime value) {
this.kehtetu = value;
}
/**
* Gets the value of the hooneKujuPindala property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getHooneKujuPindala() {
return hooneKujuPindala;
}
/**
* Sets the value of the hooneKujuPindala property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHooneKujuPindala(Double value) {
this.hooneKujuPindala = value;
}
/**
* Gets the value of the hooneKorgusR property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getHooneKorgusR() {
return hooneKorgusR;
}
/**
* Sets the value of the hooneKorgusR property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHooneKorgusR(Double value) {
this.hooneKorgusR = value;
}
/**
* Gets the value of the hooneKorgusM property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getHooneKorgusM() {
return hooneKorgusM;
}
/**
* Sets the value of the hooneKorgusM property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHooneKorgusM(Double value) {
this.hooneKorgusM = value;
}
/**
* Gets the value of the muudatuseAlgataja property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMuudatuseAlgataja() {
return muudatuseAlgataja;
}
/**
* Sets the value of the muudatuseAlgataja property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMuudatuseAlgataja(String value) {
this.muudatuseAlgataja = value;
}
/**
* Gets the value of the ruumikuju property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRuumikuju() {
return ruumikuju;
}
/**
* Sets the value of the ruumikuju property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRuumikuju(String value) {
this.ruumikuju = value;
}
/**
* Gets the value of the kujuMoodustusviis property.
*
* @return
* possible object is
* {@link AdsObjmviisType }
*
*/
public AdsObjmviisType getKujuMoodustusviis() {
return kujuMoodustusviis;
}
/**
* Sets the value of the kujuMoodustusviis property.
*
* @param value
* allowed object is
* {@link AdsObjmviisType }
*
*/
public void setKujuMoodustusviis(AdsObjmviisType value) {
this.kujuMoodustusviis = value;
}
/**
* Gets the value of the objektiPunktX property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getObjektiPunktX() {
return objektiPunktX;
}
/**
* Sets the value of the objektiPunktX property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setObjektiPunktX(Double value) {
this.objektiPunktX = value;
}
/**
* Gets the value of the objektiPunktY property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getObjektiPunktY() {
return objektiPunktY;
}
/**
* Sets the value of the objektiPunktY property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setObjektiPunktY(Double value) {
this.objektiPunktY = value;
}
/**
* Gets the value of the etakId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getEtakId() {
return etakId;
}
/**
* Sets the value of the etakId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEtakId(Long value) {
this.etakId = value;
}
/**
* Gets the value of the aadressid property.
*
* @return
* possible object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Aadressid }
*
*/
public ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Aadressid getAadressid() {
return aadressid;
}
/**
* Sets the value of the aadressid property.
*
* @param value
* allowed object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Aadressid }
*
*/
public void setAadressid(ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Aadressid value) {
this.aadressid = value;
}
/**
* Gets the value of the ehRlisaandmed property.
*
* @return
* possible object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.EHRlisaandmed }
*
*/
public ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.EHRlisaandmed getEHRlisaandmed() {
return ehRlisaandmed;
}
/**
* Sets the value of the ehRlisaandmed property.
*
* @param value
* allowed object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.EHRlisaandmed }
*
*/
public void setEHRlisaandmed(ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.EHRlisaandmed value) {
this.ehRlisaandmed = value;
}
/**
* Gets the value of the objseosed property.
*
* @return
* possible object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Objseosed }
*
*/
public ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Objseosed getObjseosed() {
return objseosed;
}
/**
* Sets the value of the objseosed property.
*
* @param value
* allowed object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Objseosed }
*
*/
public void setObjseosed(ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Objseosed value) {
this.objseosed = value;
}
/**
* Gets the value of the hooneosad property.
*
* @return
* possible object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Hooneosad }
*
*/
public ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Hooneosad getHooneosad() {
return hooneosad;
}
/**
* Sets the value of the hooneosad property.
*
* @param value
* allowed object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Hooneosad }
*
*/
public void setHooneosad(ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Hooneosad value) {
this.hooneosad = value;
}
/**
* Gets the value of the huvipunktid property.
*
* @return
* possible object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Huvipunktid }
*
*/
public ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Huvipunktid getHuvipunktid() {
return huvipunktid;
}
/**
* Sets the value of the huvipunktid property.
*
* @param value
* allowed object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Huvipunktid }
*
*/
public void setHuvipunktid(ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Huvipunktid value) {
this.huvipunktid = value;
}
/**
* Gets the value of the liidestujaObjektid property.
*
* @return
* possible object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.LiidestujaObjektid }
*
*/
public ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.LiidestujaObjektid getLiidestujaObjektid() {
return liidestujaObjektid;
}
/**
* Sets the value of the liidestujaObjektid property.
*
* @param value
* allowed object is
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.LiidestujaObjektid }
*
*/
public void setLiidestujaObjektid(ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.LiidestujaObjektid value) {
this.liidestujaObjektid = value;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"aadresses"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class Aadressid
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "aadress")
protected List aadresses;
/**
* Gets the value of the aadresses property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the aadresses property.
*
*
* For example, to add a new item, do as follows:
*
*
* getAadresses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Aadressid.Aadress }
*
*
*
* @return
* The value of the aadresses property.
*/
public List getAadresses() {
if (aadresses == null) {
aadresses = new ArrayList<>();
}
return this.aadresses;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"adrId",
"koodAadress",
"taisAadress",
"lahiAadress",
"sihtnumber",
"maPiirkond",
"maPiirkondAlias",
"aadressiPunktX",
"aadressiPunktY",
"tehniline",
"primaarseimObjekt",
"adsTase1",
"adsTase2",
"adsTase3",
"adsTase4",
"adsTase5",
"adsTase6",
"adsTase7",
"adsTase8"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class Aadress
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long adrId;
protected String koodAadress;
protected String taisAadress;
protected String lahiAadress;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long sihtnumber;
protected String maPiirkond;
protected String maPiirkondAlias;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double aadressiPunktX;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double aadressiPunktY;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean tehniline;
protected String primaarseimObjekt;
protected AdsTaseType adsTase1;
protected AdsTaseType adsTase2;
protected AdsTaseType adsTase3;
protected AdsTaseType adsTase4;
protected AdsTaseType adsTase5;
protected AdsTaseType adsTase6;
protected AdsTaseType adsTase7;
protected AdsTaseType adsTase8;
/**
* Gets the value of the adrId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getAdrId() {
return adrId;
}
/**
* Sets the value of the adrId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdrId(Long value) {
this.adrId = value;
}
/**
* Gets the value of the koodAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKoodAadress() {
return koodAadress;
}
/**
* Sets the value of the koodAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKoodAadress(String value) {
this.koodAadress = value;
}
/**
* Gets the value of the taisAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTaisAadress() {
return taisAadress;
}
/**
* Sets the value of the taisAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTaisAadress(String value) {
this.taisAadress = value;
}
/**
* Gets the value of the lahiAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLahiAadress() {
return lahiAadress;
}
/**
* Sets the value of the lahiAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLahiAadress(String value) {
this.lahiAadress = value;
}
/**
* Gets the value of the sihtnumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getSihtnumber() {
return sihtnumber;
}
/**
* Sets the value of the sihtnumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSihtnumber(Long value) {
this.sihtnumber = value;
}
/**
* Gets the value of the maPiirkond property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMaPiirkond() {
return maPiirkond;
}
/**
* Sets the value of the maPiirkond property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMaPiirkond(String value) {
this.maPiirkond = value;
}
/**
* Gets the value of the maPiirkondAlias property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMaPiirkondAlias() {
return maPiirkondAlias;
}
/**
* Sets the value of the maPiirkondAlias property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMaPiirkondAlias(String value) {
this.maPiirkondAlias = value;
}
/**
* Gets the value of the aadressiPunktX property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getAadressiPunktX() {
return aadressiPunktX;
}
/**
* Sets the value of the aadressiPunktX property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadressiPunktX(Double value) {
this.aadressiPunktX = value;
}
/**
* Gets the value of the aadressiPunktY property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getAadressiPunktY() {
return aadressiPunktY;
}
/**
* Sets the value of the aadressiPunktY property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadressiPunktY(Double value) {
this.aadressiPunktY = value;
}
/**
* Gets the value of the tehniline property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isTehniline() {
return tehniline;
}
/**
* Sets the value of the tehniline property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTehniline(Boolean value) {
this.tehniline = value;
}
/**
* Gets the value of the primaarseimObjekt property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrimaarseimObjekt() {
return primaarseimObjekt;
}
/**
* Sets the value of the primaarseimObjekt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPrimaarseimObjekt(String value) {
this.primaarseimObjekt = value;
}
/**
* Gets the value of the adsTase1 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase1() {
return adsTase1;
}
/**
* Sets the value of the adsTase1 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase1(AdsTaseType value) {
this.adsTase1 = value;
}
/**
* Gets the value of the adsTase2 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase2() {
return adsTase2;
}
/**
* Sets the value of the adsTase2 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase2(AdsTaseType value) {
this.adsTase2 = value;
}
/**
* Gets the value of the adsTase3 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase3() {
return adsTase3;
}
/**
* Sets the value of the adsTase3 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase3(AdsTaseType value) {
this.adsTase3 = value;
}
/**
* Gets the value of the adsTase4 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase4() {
return adsTase4;
}
/**
* Sets the value of the adsTase4 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase4(AdsTaseType value) {
this.adsTase4 = value;
}
/**
* Gets the value of the adsTase5 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase5() {
return adsTase5;
}
/**
* Sets the value of the adsTase5 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase5(AdsTaseType value) {
this.adsTase5 = value;
}
/**
* Gets the value of the adsTase6 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase6() {
return adsTase6;
}
/**
* Sets the value of the adsTase6 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase6(AdsTaseType value) {
this.adsTase6 = value;
}
/**
* Gets the value of the adsTase7 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase7() {
return adsTase7;
}
/**
* Sets the value of the adsTase7 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase7(AdsTaseType value) {
this.adsTase7 = value;
}
/**
* Gets the value of the adsTase8 property.
*
* @return
* possible object is
* {@link AdsTaseType }
*
*/
public AdsTaseType getAdsTase8() {
return adsTase8;
}
/**
* Sets the value of the adsTase8 property.
*
* @param value
* allowed object is
* {@link AdsTaseType }
*
*/
public void setAdsTase8(AdsTaseType value) {
this.adsTase8 = value;
}
}
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"nimetus",
"pind",
"kasutusotstarbed",
"korrus",
"staatus",
"andmedSeisuga"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class EHRlisaandmed
implements Serializable
{
private static final long serialVersionUID = -1L;
protected String nimetus;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double pind;
protected String kasutusotstarbed;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long korrus;
protected String staatus;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDate.class)
@XmlSchemaType(name = "date")
protected LocalDate andmedSeisuga;
/**
* Gets the value of the nimetus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNimetus() {
return nimetus;
}
/**
* Sets the value of the nimetus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNimetus(String value) {
this.nimetus = value;
}
/**
* Gets the value of the pind property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getPind() {
return pind;
}
/**
* Sets the value of the pind property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPind(Double value) {
this.pind = value;
}
/**
* Gets the value of the kasutusotstarbed property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKasutusotstarbed() {
return kasutusotstarbed;
}
/**
* Sets the value of the kasutusotstarbed property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKasutusotstarbed(String value) {
this.kasutusotstarbed = value;
}
/**
* Gets the value of the korrus property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getKorrus() {
return korrus;
}
/**
* Sets the value of the korrus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKorrus(Long value) {
this.korrus = value;
}
/**
* Gets the value of the staatus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStaatus() {
return staatus;
}
/**
* Sets the value of the staatus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStaatus(String value) {
this.staatus = value;
}
/**
* Gets the value of the andmedSeisuga property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getAndmedSeisuga() {
return andmedSeisuga;
}
/**
* Sets the value of the andmedSeisuga property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAndmedSeisuga(LocalDate value) {
this.andmedSeisuga = value;
}
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"hooneosas"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class Hooneosad
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "hooneosa", required = true)
protected List hooneosas;
/**
* Gets the value of the hooneosas property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the hooneosas property.
*
*
* For example, to add a new item, do as follows:
*
*
* getHooneosas().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Hooneosad.Hooneosa }
*
*
*
* @return
* The value of the hooneosas property.
*/
public List getHooneosas() {
if (hooneosas == null) {
hooneosas = new ArrayList<>();
}
return this.hooneosas;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"adsOid",
"adobId",
"objektiLiik",
"origTunnus",
"aadress",
"hooneOID"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class Hooneosa
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(required = true)
protected String adsOid;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long adobId;
@XmlSchemaType(name = "string")
protected AdsobjliikKlassifikaator objektiLiik;
protected String origTunnus;
@XmlElement(required = true)
protected String aadress;
protected String hooneOID;
/**
* Gets the value of the adsOid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAdsOid() {
return adsOid;
}
/**
* Sets the value of the adsOid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdsOid(String value) {
this.adsOid = value;
}
/**
* Gets the value of the adobId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getAdobId() {
return adobId;
}
/**
* Sets the value of the adobId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdobId(Long value) {
this.adobId = value;
}
/**
* Gets the value of the objektiLiik property.
*
* @return
* possible object is
* {@link AdsobjliikKlassifikaator }
*
*/
public AdsobjliikKlassifikaator getObjektiLiik() {
return objektiLiik;
}
/**
* Sets the value of the objektiLiik property.
*
* @param value
* allowed object is
* {@link AdsobjliikKlassifikaator }
*
*/
public void setObjektiLiik(AdsobjliikKlassifikaator value) {
this.objektiLiik = value;
}
/**
* Gets the value of the origTunnus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrigTunnus() {
return origTunnus;
}
/**
* Sets the value of the origTunnus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrigTunnus(String value) {
this.origTunnus = value;
}
/**
* Gets the value of the aadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAadress() {
return aadress;
}
/**
* Sets the value of the aadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadress(String value) {
this.aadress = value;
}
/**
* Gets the value of the hooneOID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHooneOID() {
return hooneOID;
}
/**
* Sets the value of the hooneOID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHooneOID(String value) {
this.hooneOID = value;
}
}
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"huvipunkts"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class Huvipunktid
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "huvipunkt", required = true)
protected List huvipunkts;
/**
* Gets the value of the huvipunkts property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the huvipunkts property.
*
*
* For example, to add a new item, do as follows:
*
*
* getHuvipunkts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Huvipunktid.Huvipunkt }
*
*
*
* @return
* The value of the huvipunkts property.
*/
public List getHuvipunkts() {
if (huvipunkts == null) {
huvipunkts = new ArrayList<>();
}
return this.huvipunkts;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"huviPunktiNimi"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class Huvipunkt
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(required = true)
protected String huviPunktiNimi;
/**
* Gets the value of the huviPunktiNimi property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHuviPunktiNimi() {
return huviPunktiNimi;
}
/**
* Sets the value of the huviPunktiNimi property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHuviPunktiNimi(String value) {
this.huviPunktiNimi = value;
}
}
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"liidestujaObjekts"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class LiidestujaObjektid
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "liidestujaObjekt", required = true)
protected List liidestujaObjekts;
/**
* Gets the value of the liidestujaObjekts property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the liidestujaObjekts property.
*
*
* For example, to add a new item, do as follows:
*
*
* getLiidestujaObjekts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.LiidestujaObjektid.LiidestujaObjekt }
*
*
*
* @return
* The value of the liidestujaObjekts property.
*/
public List getLiidestujaObjekts() {
if (liidestujaObjekts == null) {
liidestujaObjekts = new ArrayList<>();
}
return this.liidestujaObjekts;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"register",
"idRegistris",
"lisainfo"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class LiidestujaObjekt
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlSchemaType(name = "string")
protected AdsRegisterType register;
protected String idRegistris;
protected String lisainfo;
/**
* Gets the value of the register property.
*
* @return
* possible object is
* {@link AdsRegisterType }
*
*/
public AdsRegisterType getRegister() {
return register;
}
/**
* Sets the value of the register property.
*
* @param value
* allowed object is
* {@link AdsRegisterType }
*
*/
public void setRegister(AdsRegisterType value) {
this.register = value;
}
/**
* Gets the value of the idRegistris property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIdRegistris() {
return idRegistris;
}
/**
* Sets the value of the idRegistris property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIdRegistris(String value) {
this.idRegistris = value;
}
/**
* Gets the value of the lisainfo property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLisainfo() {
return lisainfo;
}
/**
* Sets the value of the lisainfo property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLisainfo(String value) {
this.lisainfo = value;
}
}
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"objseos"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class Objseosed
implements Serializable
{
private static final long serialVersionUID = -1L;
protected List objseos;
/**
* Gets the value of the objseos property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the objseos property.
*
*
* For example, to add a new item, do as follows:
*
*
* getObjseos().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ADSobjmuudatusedV7Response.ObjmuudatusedTulem.Muudatus.Objseosed.Objseos }
*
*
*
* @return
* The value of the objseos property.
*/
public List getObjseos() {
if (objseos == null) {
objseos = new ArrayList<>();
}
return this.objseos;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"adsOid",
"adobId",
"objektiLiik",
"origTunnus",
"aadress",
"unikaalne"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:09:07+02:00")
public static class Objseos
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(required = true)
protected String adsOid;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long adobId;
@XmlSchemaType(name = "string")
protected AdsobjliikKlassifikaator objektiLiik;
protected String origTunnus;
@XmlElement(required = true)
protected String aadress;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForBoolean.class)
@XmlSchemaType(name = "boolean")
protected Boolean unikaalne;
/**
* Gets the value of the adsOid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAdsOid() {
return adsOid;
}
/**
* Sets the value of the adsOid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdsOid(String value) {
this.adsOid = value;
}
/**
* Gets the value of the adobId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getAdobId() {
return adobId;
}
/**
* Sets the value of the adobId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdobId(Long value) {
this.adobId = value;
}
/**
* Gets the value of the objektiLiik property.
*
* @return
* possible object is
* {@link AdsobjliikKlassifikaator }
*
*/
public AdsobjliikKlassifikaator getObjektiLiik() {
return objektiLiik;
}
/**
* Sets the value of the objektiLiik property.
*
* @param value
* allowed object is
* {@link AdsobjliikKlassifikaator }
*
*/
public void setObjektiLiik(AdsobjliikKlassifikaator value) {
this.objektiLiik = value;
}
/**
* Gets the value of the origTunnus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrigTunnus() {
return origTunnus;
}
/**
* Sets the value of the origTunnus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrigTunnus(String value) {
this.origTunnus = value;
}
/**
* Gets the value of the aadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAadress() {
return aadress;
}
/**
* Sets the value of the aadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadress(String value) {
this.aadress = value;
}
/**
* Gets the value of the unikaalne property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean isUnikaalne() {
return unikaalne;
}
/**
* Sets the value of the unikaalne property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUnikaalne(Boolean value) {
this.unikaalne = value;
}
}
}
}
}
}