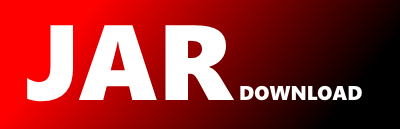
ee.xtee6.arireg.detail.DetailandmedV5VaAsukoht Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtee6-client-arireg Show documentation
Show all versions of xtee6-client-arireg Show documentation
Library for XROAD ARIREG service clints
package ee.xtee6.arireg.detail;
import java.io.Serializable;
import java.time.LocalDate;
import ee.datel.client.utils.AdapterForLocalDate;
import ee.datel.client.utils.AdapterForLong;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for detailandmed_v5_va_asukoht complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "detailandmed_v5_va_asukoht", propOrder = {
"kirjeId",
"kaardiPiirkond",
"kaardiNr",
"kaardiTyyp",
"kandeNr",
"aadressRiik",
"aadressRiikTekstina",
"aadress",
"postiindeks",
"algusKpv",
"loppKpv"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-10-29T13:15:31+02:00")
public class DetailandmedV5VaAsukoht
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "kirje_id", type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long kirjeId;
@XmlElement(name = "kaardi_piirkond", type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long kaardiPiirkond;
@XmlElement(name = "kaardi_nr", type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long kaardiNr;
@XmlElement(name = "kaardi_tyyp")
protected String kaardiTyyp;
@XmlElement(name = "kande_nr", type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "integer")
protected Long kandeNr;
@XmlElement(name = "aadress_riik")
protected String aadressRiik;
@XmlElement(name = "aadress_riik_tekstina")
protected String aadressRiikTekstina;
protected String aadress;
protected String postiindeks;
@XmlElement(name = "algus_kpv", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDate.class)
@XmlSchemaType(name = "date")
protected LocalDate algusKpv;
@XmlElement(name = "lopp_kpv", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDate.class)
@XmlSchemaType(name = "date")
protected LocalDate loppKpv;
/**
* Gets the value of the kirjeId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getKirjeId() {
return kirjeId;
}
/**
* Sets the value of the kirjeId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKirjeId(Long value) {
this.kirjeId = value;
}
/**
* Gets the value of the kaardiPiirkond property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getKaardiPiirkond() {
return kaardiPiirkond;
}
/**
* Sets the value of the kaardiPiirkond property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKaardiPiirkond(Long value) {
this.kaardiPiirkond = value;
}
/**
* Gets the value of the kaardiNr property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getKaardiNr() {
return kaardiNr;
}
/**
* Sets the value of the kaardiNr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKaardiNr(Long value) {
this.kaardiNr = value;
}
/**
* Gets the value of the kaardiTyyp property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKaardiTyyp() {
return kaardiTyyp;
}
/**
* Sets the value of the kaardiTyyp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKaardiTyyp(String value) {
this.kaardiTyyp = value;
}
/**
* Gets the value of the kandeNr property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getKandeNr() {
return kandeNr;
}
/**
* Sets the value of the kandeNr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeNr(Long value) {
this.kandeNr = value;
}
/**
* Gets the value of the aadressRiik property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAadressRiik() {
return aadressRiik;
}
/**
* Sets the value of the aadressRiik property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadressRiik(String value) {
this.aadressRiik = value;
}
/**
* Gets the value of the aadressRiikTekstina property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAadressRiikTekstina() {
return aadressRiikTekstina;
}
/**
* Sets the value of the aadressRiikTekstina property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadressRiikTekstina(String value) {
this.aadressRiikTekstina = value;
}
/**
* Gets the value of the aadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAadress() {
return aadress;
}
/**
* Sets the value of the aadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadress(String value) {
this.aadress = value;
}
/**
* Gets the value of the postiindeks property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPostiindeks() {
return postiindeks;
}
/**
* Sets the value of the postiindeks property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPostiindeks(String value) {
this.postiindeks = value;
}
/**
* Gets the value of the algusKpv property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getAlgusKpv() {
return algusKpv;
}
/**
* Sets the value of the algusKpv property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAlgusKpv(LocalDate value) {
this.algusKpv = value;
}
/**
* Gets the value of the loppKpv property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getLoppKpv() {
return loppKpv;
}
/**
* Sets the value of the loppKpv property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLoppKpv(LocalDate value) {
this.loppKpv = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy