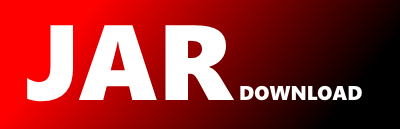
ee.xtee6.knr.nime1.NimeobjektiLogiResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtee6-client-knr Show documentation
Show all versions of xtee6-client-knr Show documentation
Library for XROAD MUINAS service clints
The newest version!
package ee.xtee6.knr.nime1;
import java.io.Serializable;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import ee.datel.client.utils.AdapterForDouble;
import ee.datel.client.utils.AdapterForLocalDate;
import ee.datel.client.utils.AdapterForLong;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for nimeobjektiLogiResponseType complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "nimeobjektiLogiResponseType", namespace = "http://www.maaamet.ee", propOrder = {
"tulems"
})
@XmlRootElement(name = "nimeobjektiLogiResponse", namespace = "http://www.maaamet.ee")
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:10:36+02:00")
public class NimeobjektiLogiResponse
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "tulem")
protected List tulems;
/**
* Gets the value of the tulems property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the tulems property.
*
*
* For example, to add a new item, do as follows:
*
*
* getTulems().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NimeobjektiLogiResponse.Tulem }
*
*
*
* @return
* The value of the tulems property.
*/
public List getTulems() {
if (tulems == null) {
tulems = new ArrayList<>();
}
return this.tulems;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"logiId",
"logiKuupaev",
"nimeobjektId",
"nimeobjektiLiigiKood",
"nimeobjektiLiigiNimetus",
"tunnuspunktX",
"tunnuspunktY",
"ruumiKuju",
"register",
"tunnusRegistris",
"adsOid",
"olek",
"ylemobjektId",
"ryhmanimeKuva",
"ametlikNimi",
"maEsikohanimi",
"maMuunimi",
"esindusAadress",
"esindusAadressKood",
"esindusAadressId",
"maakond",
"omavalitsus",
"asustusyksus",
"vaikekoht"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:10:36+02:00")
public static class Tulem
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "long")
protected Long logiId;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDate.class)
@XmlSchemaType(name = "date")
protected LocalDate logiKuupaev;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "long")
protected Long nimeobjektId;
@XmlElement(required = true)
protected String nimeobjektiLiigiKood;
@XmlElement(required = true)
protected String nimeobjektiLiigiNimetus;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double tunnuspunktX;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForDouble.class)
@XmlSchemaType(name = "double")
protected Double tunnuspunktY;
protected String ruumiKuju;
protected String register;
protected String tunnusRegistris;
protected String adsOid;
@XmlElement(required = true)
protected String olek;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "long")
protected Long ylemobjektId;
protected String ryhmanimeKuva;
protected String ametlikNimi;
protected String maEsikohanimi;
protected String maMuunimi;
protected String esindusAadress;
protected String esindusAadressKood;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(AdapterForLong.class)
@XmlSchemaType(name = "long")
protected Long esindusAadressId;
@XmlElement(required = true)
protected EhakType maakond;
@XmlElement(required = true)
protected EhakType omavalitsus;
@XmlElement(required = true)
protected EhakType asustusyksus;
@XmlElement(required = true)
protected EhakType vaikekoht;
/**
* Gets the value of the logiId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getLogiId() {
return logiId;
}
/**
* Sets the value of the logiId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLogiId(Long value) {
this.logiId = value;
}
/**
* Gets the value of the logiKuupaev property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getLogiKuupaev() {
return logiKuupaev;
}
/**
* Sets the value of the logiKuupaev property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLogiKuupaev(LocalDate value) {
this.logiKuupaev = value;
}
/**
* Gets the value of the nimeobjektId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getNimeobjektId() {
return nimeobjektId;
}
/**
* Sets the value of the nimeobjektId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNimeobjektId(Long value) {
this.nimeobjektId = value;
}
/**
* Gets the value of the nimeobjektiLiigiKood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNimeobjektiLiigiKood() {
return nimeobjektiLiigiKood;
}
/**
* Sets the value of the nimeobjektiLiigiKood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNimeobjektiLiigiKood(String value) {
this.nimeobjektiLiigiKood = value;
}
/**
* Gets the value of the nimeobjektiLiigiNimetus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNimeobjektiLiigiNimetus() {
return nimeobjektiLiigiNimetus;
}
/**
* Sets the value of the nimeobjektiLiigiNimetus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNimeobjektiLiigiNimetus(String value) {
this.nimeobjektiLiigiNimetus = value;
}
/**
* Gets the value of the tunnuspunktX property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getTunnuspunktX() {
return tunnuspunktX;
}
/**
* Sets the value of the tunnuspunktX property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTunnuspunktX(Double value) {
this.tunnuspunktX = value;
}
/**
* Gets the value of the tunnuspunktY property.
*
* @return
* possible object is
* {@link String }
*
*/
public Double getTunnuspunktY() {
return tunnuspunktY;
}
/**
* Sets the value of the tunnuspunktY property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTunnuspunktY(Double value) {
this.tunnuspunktY = value;
}
/**
* Gets the value of the ruumiKuju property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRuumiKuju() {
return ruumiKuju;
}
/**
* Sets the value of the ruumiKuju property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRuumiKuju(String value) {
this.ruumiKuju = value;
}
/**
* Gets the value of the register property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRegister() {
return register;
}
/**
* Sets the value of the register property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRegister(String value) {
this.register = value;
}
/**
* Gets the value of the tunnusRegistris property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTunnusRegistris() {
return tunnusRegistris;
}
/**
* Sets the value of the tunnusRegistris property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTunnusRegistris(String value) {
this.tunnusRegistris = value;
}
/**
* Gets the value of the adsOid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAdsOid() {
return adsOid;
}
/**
* Sets the value of the adsOid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdsOid(String value) {
this.adsOid = value;
}
/**
* Gets the value of the olek property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOlek() {
return olek;
}
/**
* Sets the value of the olek property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOlek(String value) {
this.olek = value;
}
/**
* Gets the value of the ylemobjektId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getYlemobjektId() {
return ylemobjektId;
}
/**
* Sets the value of the ylemobjektId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setYlemobjektId(Long value) {
this.ylemobjektId = value;
}
/**
* Gets the value of the ryhmanimeKuva property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRyhmanimeKuva() {
return ryhmanimeKuva;
}
/**
* Sets the value of the ryhmanimeKuva property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRyhmanimeKuva(String value) {
this.ryhmanimeKuva = value;
}
/**
* Gets the value of the ametlikNimi property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAmetlikNimi() {
return ametlikNimi;
}
/**
* Sets the value of the ametlikNimi property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAmetlikNimi(String value) {
this.ametlikNimi = value;
}
/**
* Gets the value of the maEsikohanimi property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMaEsikohanimi() {
return maEsikohanimi;
}
/**
* Sets the value of the maEsikohanimi property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMaEsikohanimi(String value) {
this.maEsikohanimi = value;
}
/**
* Gets the value of the maMuunimi property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMaMuunimi() {
return maMuunimi;
}
/**
* Sets the value of the maMuunimi property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMaMuunimi(String value) {
this.maMuunimi = value;
}
/**
* Gets the value of the esindusAadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEsindusAadress() {
return esindusAadress;
}
/**
* Sets the value of the esindusAadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEsindusAadress(String value) {
this.esindusAadress = value;
}
/**
* Gets the value of the esindusAadressKood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEsindusAadressKood() {
return esindusAadressKood;
}
/**
* Sets the value of the esindusAadressKood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEsindusAadressKood(String value) {
this.esindusAadressKood = value;
}
/**
* Gets the value of the esindusAadressId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Long getEsindusAadressId() {
return esindusAadressId;
}
/**
* Sets the value of the esindusAadressId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEsindusAadressId(Long value) {
this.esindusAadressId = value;
}
/**
* Gets the value of the maakond property.
*
* @return
* possible object is
* {@link EhakType }
*
*/
public EhakType getMaakond() {
return maakond;
}
/**
* Sets the value of the maakond property.
*
* @param value
* allowed object is
* {@link EhakType }
*
*/
public void setMaakond(EhakType value) {
this.maakond = value;
}
/**
* Gets the value of the omavalitsus property.
*
* @return
* possible object is
* {@link EhakType }
*
*/
public EhakType getOmavalitsus() {
return omavalitsus;
}
/**
* Sets the value of the omavalitsus property.
*
* @param value
* allowed object is
* {@link EhakType }
*
*/
public void setOmavalitsus(EhakType value) {
this.omavalitsus = value;
}
/**
* Gets the value of the asustusyksus property.
*
* @return
* possible object is
* {@link EhakType }
*
*/
public EhakType getAsustusyksus() {
return asustusyksus;
}
/**
* Sets the value of the asustusyksus property.
*
* @param value
* allowed object is
* {@link EhakType }
*
*/
public void setAsustusyksus(EhakType value) {
this.asustusyksus = value;
}
/**
* Gets the value of the vaikekoht property.
*
* @return
* possible object is
* {@link EhakType }
*
*/
public EhakType getVaikekoht() {
return vaikekoht;
}
/**
* Sets the value of the vaikekoht property.
*
* @param value
* allowed object is
* {@link EhakType }
*
*/
public void setVaikekoht(EhakType value) {
this.vaikekoht = value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy