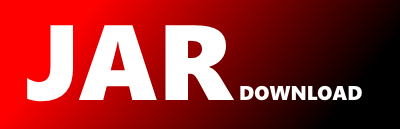
ee.datel.client.kpois.KpoisTrykisService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtee6-client-kpois Show documentation
Show all versions of xtee6-client-kpois Show documentation
Library for XROAD KPOIS service clints
The newest version!
package ee.datel.client.kpois;
/*-
* ==========================LICENSE_START===================================
* ee.datel.xtee6:xtee6-client-kpois
*
* Copyright (C) 2018 - 2024 AS Datel
*
* Redistribution and use in binary form, without modification, are permitted
* provided that the following conditions are met:
*
* 1. Redistributions must reproduce the above copyright notice, this list of
* conditions and the following disclaimer in the documentation and/or other
* materials provided with the distribution.
*
* 2. Neither the name of the copyright holder nor the names of its
* contributors may be used to endorse or promote products derived from this
* software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED
* TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
* LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
* ===========================LICENSE_END====================================
*/
import ee.datel.client.utils.ServiceUtils;
import ee.datel.xtee.fault.ServiceFaultException;
import ee.xtee6.kpois.trykis.AluskaartKlassifikaatorType;
import ee.xtee6.kpois.trykis.Client;
import ee.xtee6.kpois.trykis.FormaatKlassifikaatorType;
import ee.xtee6.kpois.trykis.KpoisPortService;
import ee.xtee6.kpois.trykis.KpoisServicePort;
import ee.xtee6.kpois.trykis.KyKitsendusteTrykisRequestType;
import ee.xtee6.kpois.trykis.KyKitsendusteTrykisResponseType;
import ee.xtee6.kpois.trykis.Service;
import java.time.LocalDate;
import jakarta.xml.ws.Holder;
import jakarta.xml.ws.WebServiceException;
public class KpoisTrykisService extends KpoisCommon {
private static KpoisPortService srv = new KpoisPortService();
private KpoisTrykisService() {}
public static byte[] getPdf(KpoisTrykisXRoadParams xroad, String katastritunnus, FormaatKlassifikaatorType formaat,
AluskaartKlassifikaatorType aluskaart, LocalDate kuupaev, String userid) throws ServiceFaultException {
KyKitsendusteTrykisRequestType request = new KyKitsendusteTrykisRequestType();
request.setKatastritunnus(katastritunnus);
request.setFormaat(formaat == null ? FormaatKlassifikaatorType.A_4 : formaat);
request.setAluskaart(aluskaart == null ? AluskaartKlassifikaatorType.PUUDUB : aluskaart);
Holder xroadRequest = new Holder<>(request);
if (kuupaev != null) {
request.setKuupaev(kuupaev);
}
Holder response = new Holder<>();
var id = ServiceUtils.getRequestId();
try {
xroad.port.kyKitsendusteTrykis(xroadRequest, response, xroad.getClient(), xroad.getService(), id, userid,
null, ServiceUtils.getProtocolVersion());
return response.value == null ? null : response.value.getTrykis();
} catch (WebServiceException ex) {
throw new ServiceFaultException(ex.getClass().getSimpleName(), ex.getMessage(), id);
}
}
public static class KpoisTrykisXRoadParams {
private KpoisServicePort port;
private Client client;
private Service service;
public KpoisTrykisXRoadParams(String serverUrl, String xRoadInstance, String memberClass, String memberCode,
String subsystemCode) {
port = srv.getKpoisServicePortSoap11();
ServiceUtils.connectPort(port, serverUrl);
client = getClientIdentifier(xRoadInstance, memberClass, memberCode, subsystemCode);
service = getServiceIdentifier(xRoadInstance);
}
public Service getService() {
return service;
}
public Client getClient() {
return client;
}
public KpoisServicePort getPort() {
return port;
}
private static Client getClientIdentifier(String xroadInstance, String memberClass, String memberCode,
String subsystemCode) {
var client = new Client();
ServiceUtils.getXRoadIdentifierType(client, xroadInstance, memberClass, memberCode, subsystemCode);
return client;
}
private static Service getServiceIdentifier(String xroadInstance) {
var server = new Service();
ServiceUtils.getXRoadIdentifierType(server, xroadInstance, memberClass, memberCode, subsystemCode,
"kyKitsendusteTrykis", "v2");
return server;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy