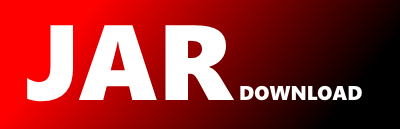
ee.xtee6.kr.dm.AadressobjektObjektiAadress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtee6-client-kr Show documentation
Show all versions of xtee6-client-kr Show documentation
Library for XROAD KR service clints
The newest version!
package ee.xtee6.kr.dm;
import java.io.Serializable;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java class for Aadressobjekt.ObjektiAadress complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Aadressobjekt.ObjektiAadress", propOrder = {
"aadressinumber",
"aadressinumberKood",
"adrId",
"asustusYksus",
"asustusYksusKood",
"koodaadress",
"korteriNumber",
"korteriNumberKood",
"liikluspind",
"liikluspindKood",
"maakond",
"maakondKood",
"nimetus",
"nimetusKood",
"omavalitsus",
"omavalitsusKood",
"taisaadress",
"vaikekoht",
"vaikekohtKood"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:10:52+02:00")
public class AadressobjektObjektiAadress
implements Serializable
{
private static final long serialVersionUID = -1L;
protected String aadressinumber;
@XmlElement(name = "aadressinumber_kood")
protected String aadressinumberKood;
@XmlElement(name = "adr_id")
protected String adrId;
@XmlElement(name = "asustus_yksus")
protected String asustusYksus;
@XmlElement(name = "asustus_yksus_kood")
protected String asustusYksusKood;
protected String koodaadress;
@XmlElement(name = "korteri_number")
protected String korteriNumber;
@XmlElement(name = "korteri_number_kood")
protected String korteriNumberKood;
protected String liikluspind;
@XmlElement(name = "liikluspind_kood")
protected String liikluspindKood;
protected String maakond;
@XmlElement(name = "maakond_kood")
protected String maakondKood;
protected String nimetus;
@XmlElement(name = "nimetus_kood")
protected String nimetusKood;
protected String omavalitsus;
@XmlElement(name = "omavalitsus_kood")
protected String omavalitsusKood;
protected String taisaadress;
protected String vaikekoht;
@XmlElement(name = "vaikekoht_kood")
protected String vaikekohtKood;
/**
* Gets the value of the aadressinumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAadressinumber() {
return aadressinumber;
}
/**
* Sets the value of the aadressinumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadressinumber(String value) {
this.aadressinumber = value;
}
/**
* Gets the value of the aadressinumberKood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAadressinumberKood() {
return aadressinumberKood;
}
/**
* Sets the value of the aadressinumberKood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAadressinumberKood(String value) {
this.aadressinumberKood = value;
}
/**
* Gets the value of the adrId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAdrId() {
return adrId;
}
/**
* Sets the value of the adrId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdrId(String value) {
this.adrId = value;
}
/**
* Gets the value of the asustusYksus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAsustusYksus() {
return asustusYksus;
}
/**
* Sets the value of the asustusYksus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAsustusYksus(String value) {
this.asustusYksus = value;
}
/**
* Gets the value of the asustusYksusKood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAsustusYksusKood() {
return asustusYksusKood;
}
/**
* Sets the value of the asustusYksusKood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAsustusYksusKood(String value) {
this.asustusYksusKood = value;
}
/**
* Gets the value of the koodaadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKoodaadress() {
return koodaadress;
}
/**
* Sets the value of the koodaadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKoodaadress(String value) {
this.koodaadress = value;
}
/**
* Gets the value of the korteriNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKorteriNumber() {
return korteriNumber;
}
/**
* Sets the value of the korteriNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKorteriNumber(String value) {
this.korteriNumber = value;
}
/**
* Gets the value of the korteriNumberKood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKorteriNumberKood() {
return korteriNumberKood;
}
/**
* Sets the value of the korteriNumberKood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKorteriNumberKood(String value) {
this.korteriNumberKood = value;
}
/**
* Gets the value of the liikluspind property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLiikluspind() {
return liikluspind;
}
/**
* Sets the value of the liikluspind property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLiikluspind(String value) {
this.liikluspind = value;
}
/**
* Gets the value of the liikluspindKood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLiikluspindKood() {
return liikluspindKood;
}
/**
* Sets the value of the liikluspindKood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLiikluspindKood(String value) {
this.liikluspindKood = value;
}
/**
* Gets the value of the maakond property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMaakond() {
return maakond;
}
/**
* Sets the value of the maakond property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMaakond(String value) {
this.maakond = value;
}
/**
* Gets the value of the maakondKood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMaakondKood() {
return maakondKood;
}
/**
* Sets the value of the maakondKood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMaakondKood(String value) {
this.maakondKood = value;
}
/**
* Gets the value of the nimetus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNimetus() {
return nimetus;
}
/**
* Sets the value of the nimetus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNimetus(String value) {
this.nimetus = value;
}
/**
* Gets the value of the nimetusKood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNimetusKood() {
return nimetusKood;
}
/**
* Sets the value of the nimetusKood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNimetusKood(String value) {
this.nimetusKood = value;
}
/**
* Gets the value of the omavalitsus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOmavalitsus() {
return omavalitsus;
}
/**
* Sets the value of the omavalitsus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOmavalitsus(String value) {
this.omavalitsus = value;
}
/**
* Gets the value of the omavalitsusKood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOmavalitsusKood() {
return omavalitsusKood;
}
/**
* Sets the value of the omavalitsusKood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOmavalitsusKood(String value) {
this.omavalitsusKood = value;
}
/**
* Gets the value of the taisaadress property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTaisaadress() {
return taisaadress;
}
/**
* Sets the value of the taisaadress property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTaisaadress(String value) {
this.taisaadress = value;
}
/**
* Gets the value of the vaikekoht property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVaikekoht() {
return vaikekoht;
}
/**
* Sets the value of the vaikekoht property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVaikekoht(String value) {
this.vaikekoht = value;
}
/**
* Gets the value of the vaikekohtKood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVaikekohtKood() {
return vaikekohtKood;
}
/**
* Sets the value of the vaikekohtKood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVaikekohtKood(String value) {
this.vaikekohtKood = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy