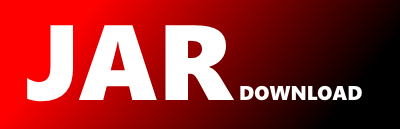
ee.xtee6.kr.dm.Jagu0 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtee6-client-kr Show documentation
Show all versions of xtee6-client-kr Show documentation
Library for XROAD KR service clints
The newest version!
package ee.xtee6.kr.dm;
import java.io.Serializable;
import java.time.LocalDateTime;
import ee.datel.client.utils.AdapterForInteger;
import ee.datel.client.utils.AdapterForLocalDateTime;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for Jagu0 complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Jagu0", propOrder = {
"avaldused",
"jaoskondVoiOsakond",
"kandeAlguskuupaev",
"kandeLiik",
"kandeLiikTekst",
"kandeLoppkuupaev",
"kandeTekst",
"nimi",
"nimiAlgusKp",
"nimiLoppKp",
"registriosaNr"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:10:52+02:00")
public class Jagu0
implements Serializable
{
private static final long serialVersionUID = -1L;
protected ArrayOfKinnistamisavaldus avaldused;
@XmlElement(name = "jaoskond_voi_osakond")
protected String jaoskondVoiOsakond;
@XmlElement(name = "kande_alguskuupaev", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime kandeAlguskuupaev;
@XmlElement(name = "kande_liik", type = String.class)
@XmlJavaTypeAdapter(AdapterForInteger.class)
@XmlSchemaType(name = "int")
protected Integer kandeLiik;
@XmlElement(name = "kande_liik_tekst")
protected String kandeLiikTekst;
@XmlElement(name = "kande_loppkuupaev", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime kandeLoppkuupaev;
@XmlElement(name = "kande_tekst")
protected String kandeTekst;
protected String nimi;
@XmlElement(name = "nimi_algus_kp", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime nimiAlgusKp;
@XmlElement(name = "nimi_lopp_kp", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime nimiLoppKp;
@XmlElement(name = "registriosa_nr")
protected String registriosaNr;
/**
* Gets the value of the avaldused property.
*
* @return
* possible object is
* {@link ArrayOfKinnistamisavaldus }
*
*/
public ArrayOfKinnistamisavaldus getAvaldused() {
return avaldused;
}
/**
* Sets the value of the avaldused property.
*
* @param value
* allowed object is
* {@link ArrayOfKinnistamisavaldus }
*
*/
public void setAvaldused(ArrayOfKinnistamisavaldus value) {
this.avaldused = value;
}
/**
* Gets the value of the jaoskondVoiOsakond property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getJaoskondVoiOsakond() {
return jaoskondVoiOsakond;
}
/**
* Sets the value of the jaoskondVoiOsakond property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJaoskondVoiOsakond(String value) {
this.jaoskondVoiOsakond = value;
}
/**
* Gets the value of the kandeAlguskuupaev property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getKandeAlguskuupaev() {
return kandeAlguskuupaev;
}
/**
* Sets the value of the kandeAlguskuupaev property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeAlguskuupaev(LocalDateTime value) {
this.kandeAlguskuupaev = value;
}
/**
* Gets the value of the kandeLiik property.
*
* @return
* possible object is
* {@link String }
*
*/
public Integer getKandeLiik() {
return kandeLiik;
}
/**
* Sets the value of the kandeLiik property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeLiik(Integer value) {
this.kandeLiik = value;
}
/**
* Gets the value of the kandeLiikTekst property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKandeLiikTekst() {
return kandeLiikTekst;
}
/**
* Sets the value of the kandeLiikTekst property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeLiikTekst(String value) {
this.kandeLiikTekst = value;
}
/**
* Gets the value of the kandeLoppkuupaev property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getKandeLoppkuupaev() {
return kandeLoppkuupaev;
}
/**
* Sets the value of the kandeLoppkuupaev property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeLoppkuupaev(LocalDateTime value) {
this.kandeLoppkuupaev = value;
}
/**
* Gets the value of the kandeTekst property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKandeTekst() {
return kandeTekst;
}
/**
* Sets the value of the kandeTekst property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeTekst(String value) {
this.kandeTekst = value;
}
/**
* Gets the value of the nimi property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNimi() {
return nimi;
}
/**
* Sets the value of the nimi property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNimi(String value) {
this.nimi = value;
}
/**
* Gets the value of the nimiAlgusKp property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getNimiAlgusKp() {
return nimiAlgusKp;
}
/**
* Sets the value of the nimiAlgusKp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNimiAlgusKp(LocalDateTime value) {
this.nimiAlgusKp = value;
}
/**
* Gets the value of the nimiLoppKp property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getNimiLoppKp() {
return nimiLoppKp;
}
/**
* Sets the value of the nimiLoppKp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNimiLoppKp(LocalDateTime value) {
this.nimiLoppKp = value;
}
/**
* Gets the value of the registriosaNr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRegistriosaNr() {
return registriosaNr;
}
/**
* Sets the value of the registriosaNr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRegistriosaNr(String value) {
this.registriosaNr = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy