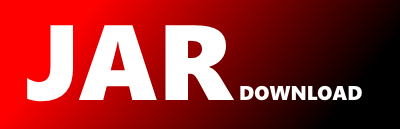
ee.xtee6.kr.dm.Jagu3 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtee6-client-kr Show documentation
Show all versions of xtee6-client-kr Show documentation
Library for XROAD KR service clints
The newest version!
package ee.xtee6.kr.dm;
import java.io.Serializable;
import java.time.LocalDateTime;
import ee.datel.client.utils.AdapterForInteger;
import ee.datel.client.utils.AdapterForLocalDateTime;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for Jagu3 complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Jagu3", propOrder = {
"hoonestusoiguseRegistriosaNr",
"jaoNr",
"kandeAlguskuupaev",
"kandeAlus",
"kandeAlusdokumendid",
"kandeKehtivus",
"kandeLiik",
"kandeLiikTekst",
"kandeLoppkuupaev",
"kandeNr",
"kandeTekst",
"koormatiseRahalineVaartus",
"koormatiseRahaliseVaartuseValuuta",
"koormatiseTahtaegAastates",
"koormatiseTahtaegKuudes",
"koormatiseTahtajaKuupaev",
"oiguseAlamliik",
"oiguseAlamliikTekst",
"oiguseLiik",
"oiguseLiikTekst",
"oiguseSeisund",
"oiguseSeisundTekst",
"oigustatudIsikud",
"registriosaNr",
"valitsevRegistriosaNr"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:10:52+02:00")
public class Jagu3
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "hoonestusoiguse_registriosa_nr")
protected String hoonestusoiguseRegistriosaNr;
@XmlElement(name = "jao_nr")
protected String jaoNr;
@XmlElement(name = "kande_alguskuupaev", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime kandeAlguskuupaev;
@XmlElement(name = "kande_alus")
protected String kandeAlus;
@XmlElement(name = "kande_alusdokumendid")
protected ArrayOfKinnistamisavaldus kandeAlusdokumendid;
@XmlElement(name = "kande_kehtivus")
protected String kandeKehtivus;
@XmlElement(name = "kande_liik", type = String.class)
@XmlJavaTypeAdapter(AdapterForInteger.class)
@XmlSchemaType(name = "int")
protected Integer kandeLiik;
@XmlElement(name = "kande_liik_tekst")
protected String kandeLiikTekst;
@XmlElement(name = "kande_loppkuupaev", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime kandeLoppkuupaev;
@XmlElement(name = "kande_nr")
protected String kandeNr;
@XmlElement(name = "kande_tekst")
protected String kandeTekst;
@XmlElement(name = "koormatise_rahaline_vaartus")
protected String koormatiseRahalineVaartus;
@XmlElement(name = "koormatise_rahalise_vaartuse_valuuta")
protected String koormatiseRahaliseVaartuseValuuta;
@XmlElement(name = "koormatise_tahtaeg_aastates", type = String.class)
@XmlJavaTypeAdapter(AdapterForInteger.class)
@XmlSchemaType(name = "int")
protected Integer koormatiseTahtaegAastates;
@XmlElement(name = "koormatise_tahtaeg_kuudes", type = String.class)
@XmlJavaTypeAdapter(AdapterForInteger.class)
@XmlSchemaType(name = "int")
protected Integer koormatiseTahtaegKuudes;
@XmlElement(name = "koormatise_tahtaja_kuupaev", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime koormatiseTahtajaKuupaev;
@XmlElement(name = "oiguse_alamliik")
protected String oiguseAlamliik;
@XmlElement(name = "oiguse_alamliik_tekst")
protected String oiguseAlamliikTekst;
@XmlElement(name = "oiguse_liik")
protected String oiguseLiik;
@XmlElement(name = "oiguse_liik_tekst")
protected String oiguseLiikTekst;
@XmlElement(name = "oiguse_seisund")
protected String oiguseSeisund;
@XmlElement(name = "oiguse_seisund_tekst")
protected String oiguseSeisundTekst;
@XmlElement(name = "oigustatud_isikud")
protected ArrayOfKinnistuIsik oigustatudIsikud;
@XmlElement(name = "registriosa_nr")
protected String registriosaNr;
@XmlElement(name = "valitsev_registriosa_nr")
protected String valitsevRegistriosaNr;
/**
* Gets the value of the hoonestusoiguseRegistriosaNr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHoonestusoiguseRegistriosaNr() {
return hoonestusoiguseRegistriosaNr;
}
/**
* Sets the value of the hoonestusoiguseRegistriosaNr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHoonestusoiguseRegistriosaNr(String value) {
this.hoonestusoiguseRegistriosaNr = value;
}
/**
* Gets the value of the jaoNr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getJaoNr() {
return jaoNr;
}
/**
* Sets the value of the jaoNr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJaoNr(String value) {
this.jaoNr = value;
}
/**
* Gets the value of the kandeAlguskuupaev property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getKandeAlguskuupaev() {
return kandeAlguskuupaev;
}
/**
* Sets the value of the kandeAlguskuupaev property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeAlguskuupaev(LocalDateTime value) {
this.kandeAlguskuupaev = value;
}
/**
* Gets the value of the kandeAlus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKandeAlus() {
return kandeAlus;
}
/**
* Sets the value of the kandeAlus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeAlus(String value) {
this.kandeAlus = value;
}
/**
* Gets the value of the kandeAlusdokumendid property.
*
* @return
* possible object is
* {@link ArrayOfKinnistamisavaldus }
*
*/
public ArrayOfKinnistamisavaldus getKandeAlusdokumendid() {
return kandeAlusdokumendid;
}
/**
* Sets the value of the kandeAlusdokumendid property.
*
* @param value
* allowed object is
* {@link ArrayOfKinnistamisavaldus }
*
*/
public void setKandeAlusdokumendid(ArrayOfKinnistamisavaldus value) {
this.kandeAlusdokumendid = value;
}
/**
* Gets the value of the kandeKehtivus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKandeKehtivus() {
return kandeKehtivus;
}
/**
* Sets the value of the kandeKehtivus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeKehtivus(String value) {
this.kandeKehtivus = value;
}
/**
* Gets the value of the kandeLiik property.
*
* @return
* possible object is
* {@link String }
*
*/
public Integer getKandeLiik() {
return kandeLiik;
}
/**
* Sets the value of the kandeLiik property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeLiik(Integer value) {
this.kandeLiik = value;
}
/**
* Gets the value of the kandeLiikTekst property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKandeLiikTekst() {
return kandeLiikTekst;
}
/**
* Sets the value of the kandeLiikTekst property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeLiikTekst(String value) {
this.kandeLiikTekst = value;
}
/**
* Gets the value of the kandeLoppkuupaev property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getKandeLoppkuupaev() {
return kandeLoppkuupaev;
}
/**
* Sets the value of the kandeLoppkuupaev property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeLoppkuupaev(LocalDateTime value) {
this.kandeLoppkuupaev = value;
}
/**
* Gets the value of the kandeNr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKandeNr() {
return kandeNr;
}
/**
* Sets the value of the kandeNr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeNr(String value) {
this.kandeNr = value;
}
/**
* Gets the value of the kandeTekst property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKandeTekst() {
return kandeTekst;
}
/**
* Sets the value of the kandeTekst property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKandeTekst(String value) {
this.kandeTekst = value;
}
/**
* Gets the value of the koormatiseRahalineVaartus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKoormatiseRahalineVaartus() {
return koormatiseRahalineVaartus;
}
/**
* Sets the value of the koormatiseRahalineVaartus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKoormatiseRahalineVaartus(String value) {
this.koormatiseRahalineVaartus = value;
}
/**
* Gets the value of the koormatiseRahaliseVaartuseValuuta property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKoormatiseRahaliseVaartuseValuuta() {
return koormatiseRahaliseVaartuseValuuta;
}
/**
* Sets the value of the koormatiseRahaliseVaartuseValuuta property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKoormatiseRahaliseVaartuseValuuta(String value) {
this.koormatiseRahaliseVaartuseValuuta = value;
}
/**
* Gets the value of the koormatiseTahtaegAastates property.
*
* @return
* possible object is
* {@link String }
*
*/
public Integer getKoormatiseTahtaegAastates() {
return koormatiseTahtaegAastates;
}
/**
* Sets the value of the koormatiseTahtaegAastates property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKoormatiseTahtaegAastates(Integer value) {
this.koormatiseTahtaegAastates = value;
}
/**
* Gets the value of the koormatiseTahtaegKuudes property.
*
* @return
* possible object is
* {@link String }
*
*/
public Integer getKoormatiseTahtaegKuudes() {
return koormatiseTahtaegKuudes;
}
/**
* Sets the value of the koormatiseTahtaegKuudes property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKoormatiseTahtaegKuudes(Integer value) {
this.koormatiseTahtaegKuudes = value;
}
/**
* Gets the value of the koormatiseTahtajaKuupaev property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getKoormatiseTahtajaKuupaev() {
return koormatiseTahtajaKuupaev;
}
/**
* Sets the value of the koormatiseTahtajaKuupaev property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKoormatiseTahtajaKuupaev(LocalDateTime value) {
this.koormatiseTahtajaKuupaev = value;
}
/**
* Gets the value of the oiguseAlamliik property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOiguseAlamliik() {
return oiguseAlamliik;
}
/**
* Sets the value of the oiguseAlamliik property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOiguseAlamliik(String value) {
this.oiguseAlamliik = value;
}
/**
* Gets the value of the oiguseAlamliikTekst property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOiguseAlamliikTekst() {
return oiguseAlamliikTekst;
}
/**
* Sets the value of the oiguseAlamliikTekst property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOiguseAlamliikTekst(String value) {
this.oiguseAlamliikTekst = value;
}
/**
* Gets the value of the oiguseLiik property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOiguseLiik() {
return oiguseLiik;
}
/**
* Sets the value of the oiguseLiik property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOiguseLiik(String value) {
this.oiguseLiik = value;
}
/**
* Gets the value of the oiguseLiikTekst property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOiguseLiikTekst() {
return oiguseLiikTekst;
}
/**
* Sets the value of the oiguseLiikTekst property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOiguseLiikTekst(String value) {
this.oiguseLiikTekst = value;
}
/**
* Gets the value of the oiguseSeisund property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOiguseSeisund() {
return oiguseSeisund;
}
/**
* Sets the value of the oiguseSeisund property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOiguseSeisund(String value) {
this.oiguseSeisund = value;
}
/**
* Gets the value of the oiguseSeisundTekst property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOiguseSeisundTekst() {
return oiguseSeisundTekst;
}
/**
* Sets the value of the oiguseSeisundTekst property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOiguseSeisundTekst(String value) {
this.oiguseSeisundTekst = value;
}
/**
* Gets the value of the oigustatudIsikud property.
*
* @return
* possible object is
* {@link ArrayOfKinnistuIsik }
*
*/
public ArrayOfKinnistuIsik getOigustatudIsikud() {
return oigustatudIsikud;
}
/**
* Sets the value of the oigustatudIsikud property.
*
* @param value
* allowed object is
* {@link ArrayOfKinnistuIsik }
*
*/
public void setOigustatudIsikud(ArrayOfKinnistuIsik value) {
this.oigustatudIsikud = value;
}
/**
* Gets the value of the registriosaNr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRegistriosaNr() {
return registriosaNr;
}
/**
* Sets the value of the registriosaNr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRegistriosaNr(String value) {
this.registriosaNr = value;
}
/**
* Gets the value of the valitsevRegistriosaNr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getValitsevRegistriosaNr() {
return valitsevRegistriosaNr;
}
/**
* Sets the value of the valitsevRegistriosaNr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setValitsevRegistriosaNr(String value) {
this.valitsevRegistriosaNr = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy