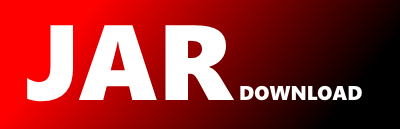
ee.xtee6.kr.dm.KinnistusraamatuMuudatusedRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtee6-client-kr Show documentation
Show all versions of xtee6-client-kr Show documentation
Library for XROAD KR service clints
The newest version!
package ee.xtee6.kr.dm;
import java.io.Serializable;
import java.time.LocalDateTime;
import ee.datel.client.utils.AdapterForInteger;
import ee.datel.client.utils.AdapterForLocalDateTime;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for Kinnistusraamatu_MuudatusedRequest complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Kinnistusraamatu_MuudatusedRequest", propOrder = {
"isikuLiikID",
"jaoNumber",
"kasutajanimi",
"katastritunnus",
"kinnistuLiik",
"kinnistuteArvLehel",
"korteriyhistuRegistrikood",
"kuupaevAlgus",
"kuupaevLopp",
"lehekyljeNr",
"muudatusteValik",
"omavalitsus",
"parool",
"registriosaNr"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:10:52+02:00")
public class KinnistusraamatuMuudatusedRequest
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "isiku_liik_ID", type = String.class)
@XmlJavaTypeAdapter(AdapterForInteger.class)
@XmlSchemaType(name = "int")
protected Integer isikuLiikID;
@XmlElement(name = "jao_number")
protected String jaoNumber;
protected String kasutajanimi;
protected String katastritunnus;
@XmlElement(name = "kinnistu_liik")
protected String kinnistuLiik;
@XmlElement(name = "kinnistute_arv_lehel", type = String.class)
@XmlJavaTypeAdapter(AdapterForInteger.class)
@XmlSchemaType(name = "int")
protected Integer kinnistuteArvLehel;
@XmlElement(name = "korteriyhistu_registrikood")
protected String korteriyhistuRegistrikood;
@XmlElement(name = "kuupaev_algus", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime kuupaevAlgus;
@XmlElement(name = "kuupaev_lopp", type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime kuupaevLopp;
@XmlElement(name = "lehekylje_nr", type = String.class)
@XmlJavaTypeAdapter(AdapterForInteger.class)
@XmlSchemaType(name = "int")
protected Integer lehekyljeNr;
@XmlElement(name = "muudatuste_valik")
protected String muudatusteValik;
protected String omavalitsus;
protected String parool;
@XmlElement(name = "registriosa_nr")
protected String registriosaNr;
/**
* Gets the value of the isikuLiikID property.
*
* @return
* possible object is
* {@link String }
*
*/
public Integer getIsikuLiikID() {
return isikuLiikID;
}
/**
* Sets the value of the isikuLiikID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIsikuLiikID(Integer value) {
this.isikuLiikID = value;
}
/**
* Gets the value of the jaoNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getJaoNumber() {
return jaoNumber;
}
/**
* Sets the value of the jaoNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJaoNumber(String value) {
this.jaoNumber = value;
}
/**
* Gets the value of the kasutajanimi property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKasutajanimi() {
return kasutajanimi;
}
/**
* Sets the value of the kasutajanimi property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKasutajanimi(String value) {
this.kasutajanimi = value;
}
/**
* Gets the value of the katastritunnus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKatastritunnus() {
return katastritunnus;
}
/**
* Sets the value of the katastritunnus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKatastritunnus(String value) {
this.katastritunnus = value;
}
/**
* Gets the value of the kinnistuLiik property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKinnistuLiik() {
return kinnistuLiik;
}
/**
* Sets the value of the kinnistuLiik property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKinnistuLiik(String value) {
this.kinnistuLiik = value;
}
/**
* Gets the value of the kinnistuteArvLehel property.
*
* @return
* possible object is
* {@link String }
*
*/
public Integer getKinnistuteArvLehel() {
return kinnistuteArvLehel;
}
/**
* Sets the value of the kinnistuteArvLehel property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKinnistuteArvLehel(Integer value) {
this.kinnistuteArvLehel = value;
}
/**
* Gets the value of the korteriyhistuRegistrikood property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKorteriyhistuRegistrikood() {
return korteriyhistuRegistrikood;
}
/**
* Sets the value of the korteriyhistuRegistrikood property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKorteriyhistuRegistrikood(String value) {
this.korteriyhistuRegistrikood = value;
}
/**
* Gets the value of the kuupaevAlgus property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getKuupaevAlgus() {
return kuupaevAlgus;
}
/**
* Sets the value of the kuupaevAlgus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKuupaevAlgus(LocalDateTime value) {
this.kuupaevAlgus = value;
}
/**
* Gets the value of the kuupaevLopp property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getKuupaevLopp() {
return kuupaevLopp;
}
/**
* Sets the value of the kuupaevLopp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKuupaevLopp(LocalDateTime value) {
this.kuupaevLopp = value;
}
/**
* Gets the value of the lehekyljeNr property.
*
* @return
* possible object is
* {@link String }
*
*/
public Integer getLehekyljeNr() {
return lehekyljeNr;
}
/**
* Sets the value of the lehekyljeNr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLehekyljeNr(Integer value) {
this.lehekyljeNr = value;
}
/**
* Gets the value of the muudatusteValik property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMuudatusteValik() {
return muudatusteValik;
}
/**
* Sets the value of the muudatusteValik property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMuudatusteValik(String value) {
this.muudatusteValik = value;
}
/**
* Gets the value of the omavalitsus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOmavalitsus() {
return omavalitsus;
}
/**
* Sets the value of the omavalitsus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOmavalitsus(String value) {
this.omavalitsus = value;
}
/**
* Gets the value of the parool property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getParool() {
return parool;
}
/**
* Sets the value of the parool property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setParool(String value) {
this.parool = value;
}
/**
* Gets the value of the registriosaNr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRegistriosaNr() {
return registriosaNr;
}
/**
* Sets the value of the registriosaNr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRegistriosaNr(String value) {
this.registriosaNr = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy