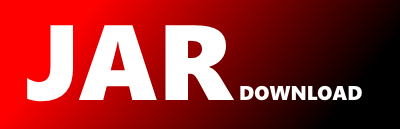
ee.xtee6.mis.ehlogi.ObjectFactory Maven / Gradle / Ivy
Show all versions of xtee6-client-mis Show documentation
package ee.xtee6.mis.ehlogi;
import javax.xml.namespace.QName;
import jakarta.annotation.Generated;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlElementDecl;
import jakarta.xml.bind.annotation.XmlRegistry;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the ee.xtee6.mis.ehlogi package.
* An ObjectFactory allows you to programmatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:11:25+02:00")
public class ObjectFactory {
private static final QName _XRoadInstance_QNAME = new QName("http://x-road.eu/xsd/identifiers", "xRoadInstance");
private static final QName _MemberClass_QNAME = new QName("http://x-road.eu/xsd/identifiers", "memberClass");
private static final QName _MemberCode_QNAME = new QName("http://x-road.eu/xsd/identifiers", "memberCode");
private static final QName _SubsystemCode_QNAME = new QName("http://x-road.eu/xsd/identifiers", "subsystemCode");
private static final QName _GroupCode_QNAME = new QName("http://x-road.eu/xsd/identifiers", "groupCode");
private static final QName _ServiceCode_QNAME = new QName("http://x-road.eu/xsd/identifiers", "serviceCode");
private static final QName _ServiceVersion_QNAME = new QName("http://x-road.eu/xsd/identifiers", "serviceVersion");
private static final QName _SecurityCategoryCode_QNAME = new QName("http://x-road.eu/xsd/identifiers", "securityCategoryCode");
private static final QName _ServerCode_QNAME = new QName("http://x-road.eu/xsd/identifiers", "serverCode");
private static final QName _Id_QNAME = new QName("http://x-road.eu/xsd/xroad.xsd", "id");
private static final QName _UserId_QNAME = new QName("http://x-road.eu/xsd/xroad.xsd", "userId");
private static final QName _Issue_QNAME = new QName("http://x-road.eu/xsd/xroad.xsd", "issue");
private static final QName _ProtocolVersion_QNAME = new QName("http://x-road.eu/xsd/xroad.xsd", "protocolVersion");
private static final QName _Version_QNAME = new QName("http://x-road.eu/xsd/xroad.xsd", "version");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: ee.xtee6.mis.ehlogi
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Ehaklogi }
*
* @return
* the new instance of {@link Ehaklogi }
*/
public Ehaklogi createEhaklogi() {
return new Ehaklogi();
}
/**
* Create an instance of {@link EhaklogiResponse }
*
* @return
* the new instance of {@link EhaklogiResponse }
*/
public EhaklogiResponse createEhaklogiResponse() {
return new EhaklogiResponse();
}
/**
* Create an instance of {@link EhaklogiResponse.Tulem }
*
* @return
* the new instance of {@link EhaklogiResponse.Tulem }
*/
public EhaklogiResponse.Tulem createEhaklogiResponseTulem() {
return new EhaklogiResponse.Tulem();
}
/**
* Create an instance of {@link Ehaklogi.Ajavahemik }
*
* @return
* the new instance of {@link Ehaklogi.Ajavahemik }
*/
public Ehaklogi.Ajavahemik createEhaklogiAjavahemik() {
return new Ehaklogi.Ajavahemik();
}
/**
* Create an instance of {@link EhaklogiResponse.Fault }
*
* @return
* the new instance of {@link EhaklogiResponse.Fault }
*/
public EhaklogiResponse.Fault createEhaklogiResponseFault() {
return new EhaklogiResponse.Fault();
}
/**
* Create an instance of {@link AjavahemikType }
*
* @return
* the new instance of {@link AjavahemikType }
*/
public AjavahemikType createAjavahemikType() {
return new AjavahemikType();
}
/**
* Create an instance of {@link AadressType }
*
* @return
* the new instance of {@link AadressType }
*/
public AadressType createAadressType() {
return new AadressType();
}
/**
* Create an instance of {@link EHAKlogiType }
*
* @return
* the new instance of {@link EHAKlogiType }
*/
public EHAKlogiType createEHAKlogiType() {
return new EHAKlogiType();
}
/**
* Create an instance of {@link GenRuumiandmedType }
*
* @return
* the new instance of {@link GenRuumiandmedType }
*/
public GenRuumiandmedType createGenRuumiandmedType() {
return new GenRuumiandmedType();
}
/**
* Create an instance of {@link AdsTaseType }
*
* @return
* the new instance of {@link AdsTaseType }
*/
public AdsTaseType createAdsTaseType() {
return new AdsTaseType();
}
/**
* Create an instance of {@link AdsTaseSimpleType }
*
* @return
* the new instance of {@link AdsTaseSimpleType }
*/
public AdsTaseSimpleType createAdsTaseSimpleType() {
return new AdsTaseSimpleType();
}
/**
* Create an instance of {@link EHAKType }
*
* @return
* the new instance of {@link EHAKType }
*/
public EHAKType createEHAKType() {
return new EHAKType();
}
/**
* Create an instance of {@link EHAKtekstAndmedType }
*
* @return
* the new instance of {@link EHAKtekstAndmedType }
*/
public EHAKtekstAndmedType createEHAKtekstAndmedType() {
return new EHAKtekstAndmedType();
}
/**
* Create an instance of {@link Client }
*
* @return
* the new instance of {@link Client }
*/
public Client createClient() {
return new Client();
}
/**
* Create an instance of {@link XRoadIdentifierType }
*
* @return
* the new instance of {@link XRoadIdentifierType }
*/
public XRoadIdentifierType createXRoadIdentifierType() {
return new XRoadIdentifierType();
}
/**
* Create an instance of {@link Service }
*
* @return
* the new instance of {@link Service }
*/
public Service createService() {
return new Service();
}
/**
* Create an instance of {@link CentralService }
*
* @return
* the new instance of {@link CentralService }
*/
public CentralService createCentralService() {
return new CentralService();
}
/**
* Create an instance of {@link RequestHash }
*
* @return
* the new instance of {@link RequestHash }
*/
public RequestHash createRequestHash() {
return new RequestHash();
}
/**
* Create an instance of {@link Title }
*
* @return
* the new instance of {@link Title }
*/
public Title createTitle() {
return new Title();
}
/**
* Create an instance of {@link Notes }
*
* @return
* the new instance of {@link Notes }
*/
public Notes createNotes() {
return new Notes();
}
/**
* Create an instance of {@link TechNotes }
*
* @return
* the new instance of {@link TechNotes }
*/
public TechNotes createTechNotes() {
return new TechNotes();
}
/**
* Create an instance of {@link XRoadSecurityCategoryIdentifierType }
*
* @return
* the new instance of {@link XRoadSecurityCategoryIdentifierType }
*/
public XRoadSecurityCategoryIdentifierType createXRoadSecurityCategoryIdentifierType() {
return new XRoadSecurityCategoryIdentifierType();
}
/**
* Create an instance of {@link XRoadSecurityServerIdentifierType }
*
* @return
* the new instance of {@link XRoadSecurityServerIdentifierType }
*/
public XRoadSecurityServerIdentifierType createXRoadSecurityServerIdentifierType() {
return new XRoadSecurityServerIdentifierType();
}
/**
* Create an instance of {@link XRoadGlobalGroupIdentifierType }
*
* @return
* the new instance of {@link XRoadGlobalGroupIdentifierType }
*/
public XRoadGlobalGroupIdentifierType createXRoadGlobalGroupIdentifierType() {
return new XRoadGlobalGroupIdentifierType();
}
/**
* Create an instance of {@link XRoadLocalGroupIdentifierType }
*
* @return
* the new instance of {@link XRoadLocalGroupIdentifierType }
*/
public XRoadLocalGroupIdentifierType createXRoadLocalGroupIdentifierType() {
return new XRoadLocalGroupIdentifierType();
}
/**
* Create an instance of {@link EhaklogiResponse.Tulem.Ehakandmed }
*
* @return
* the new instance of {@link EhaklogiResponse.Tulem.Ehakandmed }
*/
public EhaklogiResponse.Tulem.Ehakandmed createEhaklogiResponseTulemEhakandmed() {
return new EhaklogiResponse.Tulem.Ehakandmed();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/identifiers", name = "xRoadInstance")
public JAXBElement createXRoadInstance(String value) {
return new JAXBElement<>(_XRoadInstance_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/identifiers", name = "memberClass")
public JAXBElement createMemberClass(String value) {
return new JAXBElement<>(_MemberClass_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/identifiers", name = "memberCode")
public JAXBElement createMemberCode(String value) {
return new JAXBElement<>(_MemberCode_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/identifiers", name = "subsystemCode")
public JAXBElement createSubsystemCode(String value) {
return new JAXBElement<>(_SubsystemCode_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/identifiers", name = "groupCode")
public JAXBElement createGroupCode(String value) {
return new JAXBElement<>(_GroupCode_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/identifiers", name = "serviceCode")
public JAXBElement createServiceCode(String value) {
return new JAXBElement<>(_ServiceCode_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/identifiers", name = "serviceVersion")
public JAXBElement createServiceVersion(String value) {
return new JAXBElement<>(_ServiceVersion_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/identifiers", name = "securityCategoryCode")
public JAXBElement createSecurityCategoryCode(String value) {
return new JAXBElement<>(_SecurityCategoryCode_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/identifiers", name = "serverCode")
public JAXBElement createServerCode(String value) {
return new JAXBElement<>(_ServerCode_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/xroad.xsd", name = "id")
public JAXBElement createId(String value) {
return new JAXBElement<>(_Id_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/xroad.xsd", name = "userId")
public JAXBElement createUserId(String value) {
return new JAXBElement<>(_UserId_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/xroad.xsd", name = "issue")
public JAXBElement createIssue(String value) {
return new JAXBElement<>(_Issue_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/xroad.xsd", name = "protocolVersion")
public JAXBElement createProtocolVersion(String value) {
return new JAXBElement<>(_ProtocolVersion_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://x-road.eu/xsd/xroad.xsd", name = "version")
public JAXBElement createVersion(String value) {
return new JAXBElement<>(_Version_QNAME, String.class, null, value);
}
}