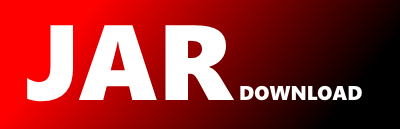
ee.xtee6.mis.logi.KylogiResponse Maven / Gradle / Ivy
Show all versions of xtee6-client-mis Show documentation
package ee.xtee6.mis.logi;
import java.io.Serializable;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
import ee.datel.client.utils.AdapterForInteger;
import ee.datel.client.utils.AdapterForLocalDate;
import ee.datel.client.utils.AdapterForLocalDateTime;
import jakarta.annotation.Generated;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for kylogiVastusType complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "kylogiVastusType", propOrder = {
"tulemid",
"fault"
})
@XmlRootElement(name = "kylogiResponse")
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:11:24+02:00")
public class KylogiResponse
implements Serializable
{
private static final long serialVersionUID = -1L;
protected KylogiResponse.Tulemid tulemid;
protected KylogiResponse.Fault fault;
/**
* Gets the value of the tulemid property.
*
* @return
* possible object is
* {@link KylogiResponse.Tulemid }
*
*/
public KylogiResponse.Tulemid getTulemid() {
return tulemid;
}
/**
* Sets the value of the tulemid property.
*
* @param value
* allowed object is
* {@link KylogiResponse.Tulemid }
*
*/
public void setTulemid(KylogiResponse.Tulemid value) {
this.tulemid = value;
}
/**
* Gets the value of the fault property.
*
* @return
* possible object is
* {@link KylogiResponse.Fault }
*
*/
public KylogiResponse.Fault getFault() {
return fault;
}
/**
* Sets the value of the fault property.
*
* @param value
* allowed object is
* {@link KylogiResponse.Fault }
*
*/
public void setFault(KylogiResponse.Fault value) {
this.fault = value;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"faultCode",
"faultString"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:11:24+02:00")
public static class Fault
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(required = true)
protected String faultCode;
@XmlElement(required = true)
protected String faultString;
/**
* Gets the value of the faultCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFaultCode() {
return faultCode;
}
/**
* Sets the value of the faultCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFaultCode(String value) {
this.faultCode = value;
}
/**
* Gets the value of the faultString property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFaultString() {
return faultString;
}
/**
* Sets the value of the faultString property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFaultString(String value) {
this.faultString = value;
}
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"tulems"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:11:24+02:00")
public static class Tulemid
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "tulem", required = true)
protected List tulems;
/**
* Gets the value of the tulems property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the tulems property.
*
*
* For example, to add a new item, do as follows:
*
*
* getTulems().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link KylogiResponse.Tulemid.Tulem }
*
*
*
* @return
* The value of the tulems property.
*/
public List getTulems() {
if (tulems == null) {
tulems = new ArrayList<>();
}
return this.tulems;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"logi",
"kyandmed"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:11:24+02:00")
public static class Tulem
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(required = true)
protected KylogiResponse.Tulemid.Tulem.Logi logi;
protected KylogiResponse.Tulemid.Tulem.Kyandmed kyandmed;
/**
* Gets the value of the logi property.
*
* @return
* possible object is
* {@link KylogiResponse.Tulemid.Tulem.Logi }
*
*/
public KylogiResponse.Tulemid.Tulem.Logi getLogi() {
return logi;
}
/**
* Sets the value of the logi property.
*
* @param value
* allowed object is
* {@link KylogiResponse.Tulemid.Tulem.Logi }
*
*/
public void setLogi(KylogiResponse.Tulemid.Tulem.Logi value) {
this.logi = value;
}
/**
* Gets the value of the kyandmed property.
*
* @return
* possible object is
* {@link KylogiResponse.Tulemid.Tulem.Kyandmed }
*
*/
public KylogiResponse.Tulemid.Tulem.Kyandmed getKyandmed() {
return kyandmed;
}
/**
* Sets the value of the kyandmed property.
*
* @param value
* allowed object is
* {@link KylogiResponse.Tulemid.Tulem.Kyandmed }
*
*/
public void setKyandmed(KylogiResponse.Tulemid.Tulem.Kyandmed value) {
this.kyandmed = value;
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"uued",
"olid"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:11:24+02:00")
public static class Kyandmed
implements Serializable
{
private static final long serialVersionUID = -1L;
protected KatastriyksusType uued;
protected KatastriyksusType olid;
/**
* Gets the value of the uued property.
*
* @return
* possible object is
* {@link KatastriyksusType }
*
*/
public KatastriyksusType getUued() {
return uued;
}
/**
* Sets the value of the uued property.
*
* @param value
* allowed object is
* {@link KatastriyksusType }
*
*/
public void setUued(KatastriyksusType value) {
this.uued = value;
}
/**
* Gets the value of the olid property.
*
* @return
* possible object is
* {@link KatastriyksusType }
*
*/
public KatastriyksusType getOlid() {
return olid;
}
/**
* Sets the value of the olid property.
*
* @param value
* allowed object is
* {@link KatastriyksusType }
*
*/
public void setOlid(KatastriyksusType value) {
this.olid = value;
}
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"kYtunnus",
"toiming",
"toimingid",
"suund",
"registreerimisaeg",
"logStamp",
"logId",
"muutLiik",
"kyOlek",
"tsentroid",
"muudatusvektor",
"tagasiAndmevektor",
"parinebMillest"
})
@Generated(value = "com.sun.tools.ws.wscompile.WsimportTool", comments = "XML-WS Tools 4.0.2", date = "2024-11-22T14:11:24+02:00")
public static class Logi
implements Serializable
{
private static final long serialVersionUID = -1L;
@XmlElement(name = "KYtunnus", required = true)
protected String kYtunnus;
@XmlElement(required = true)
protected String toiming;
@XmlElement(required = true)
protected String toimingid;
@XmlElement(required = true)
protected String suund;
@XmlElement(required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDate.class)
@XmlSchemaType(name = "date")
protected LocalDate registreerimisaeg;
@XmlElement(name = "log_stamp", required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForLocalDateTime.class)
@XmlSchemaType(name = "dateTime")
protected LocalDateTime logStamp;
@XmlElement(name = "log_id", required = true, type = String.class)
@XmlJavaTypeAdapter(AdapterForInteger.class)
@XmlSchemaType(name = "int")
protected Integer logId;
@XmlElement(name = "muut_liik", required = true)
@XmlSchemaType(name = "string")
protected MuutliikType muutLiik;
@XmlElement(name = "ky_olek", required = true)
@XmlSchemaType(name = "string")
protected OlekType kyOlek;
protected String tsentroid;
@XmlElement(required = true)
protected String muudatusvektor;
@XmlElement(required = true)
protected String tagasiAndmevektor;
@XmlElement(name = "parineb_millest")
protected String parinebMillest;
/**
* Gets the value of the kYtunnus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKYtunnus() {
return kYtunnus;
}
/**
* Sets the value of the kYtunnus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKYtunnus(String value) {
this.kYtunnus = value;
}
/**
* Gets the value of the toiming property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getToiming() {
return toiming;
}
/**
* Sets the value of the toiming property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setToiming(String value) {
this.toiming = value;
}
/**
* Gets the value of the toimingid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getToimingid() {
return toimingid;
}
/**
* Sets the value of the toimingid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setToimingid(String value) {
this.toimingid = value;
}
/**
* Gets the value of the suund property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSuund() {
return suund;
}
/**
* Sets the value of the suund property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSuund(String value) {
this.suund = value;
}
/**
* Gets the value of the registreerimisaeg property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getRegistreerimisaeg() {
return registreerimisaeg;
}
/**
* Sets the value of the registreerimisaeg property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRegistreerimisaeg(LocalDate value) {
this.registreerimisaeg = value;
}
/**
* Gets the value of the logStamp property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDateTime getLogStamp() {
return logStamp;
}
/**
* Sets the value of the logStamp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLogStamp(LocalDateTime value) {
this.logStamp = value;
}
/**
* Gets the value of the logId property.
*
* @return
* possible object is
* {@link String }
*
*/
public Integer getLogId() {
return logId;
}
/**
* Sets the value of the logId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLogId(Integer value) {
this.logId = value;
}
/**
* Gets the value of the muutLiik property.
*
* @return
* possible object is
* {@link MuutliikType }
*
*/
public MuutliikType getMuutLiik() {
return muutLiik;
}
/**
* Sets the value of the muutLiik property.
*
* @param value
* allowed object is
* {@link MuutliikType }
*
*/
public void setMuutLiik(MuutliikType value) {
this.muutLiik = value;
}
/**
* Gets the value of the kyOlek property.
*
* @return
* possible object is
* {@link OlekType }
*
*/
public OlekType getKyOlek() {
return kyOlek;
}
/**
* Sets the value of the kyOlek property.
*
* @param value
* allowed object is
* {@link OlekType }
*
*/
public void setKyOlek(OlekType value) {
this.kyOlek = value;
}
/**
* Gets the value of the tsentroid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTsentroid() {
return tsentroid;
}
/**
* Sets the value of the tsentroid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTsentroid(String value) {
this.tsentroid = value;
}
/**
* Gets the value of the muudatusvektor property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMuudatusvektor() {
return muudatusvektor;
}
/**
* Sets the value of the muudatusvektor property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMuudatusvektor(String value) {
this.muudatusvektor = value;
}
/**
* Gets the value of the tagasiAndmevektor property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTagasiAndmevektor() {
return tagasiAndmevektor;
}
/**
* Sets the value of the tagasiAndmevektor property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTagasiAndmevektor(String value) {
this.tagasiAndmevektor = value;
}
/**
* Gets the value of the parinebMillest property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getParinebMillest() {
return parinebMillest;
}
/**
* Sets the value of the parinebMillest property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setParinebMillest(String value) {
this.parinebMillest = value;
}
}
}
}
}