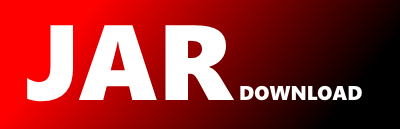
ee.datel.client.rr.RR67MuutusV1Service Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xtee6-client-rr Show documentation
Show all versions of xtee6-client-rr Show documentation
Library for XROAD RR service clints
The newest version!
package ee.datel.client.rr;
/*-
* ==========================LICENSE_START===================================
* ee.datel.xtee6:xtee6-client-rr
*
* Copyright (C) 2018 - 2024 AS Datel
*
* Redistribution and use in binary form, without modification, are permitted
* provided that the following conditions are met:
*
* 1. Redistributions must reproduce the above copyright notice, this list of
* conditions and the following disclaimer in the documentation and/or other
* materials provided with the distribution.
*
* 2. Neither the name of the copyright holder nor the names of its
* contributors may be used to endorse or promote products derived from this
* software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED
* TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
* LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
* ===========================LICENSE_END====================================
*/
import ee.datel.client.utils.ServiceUtils;
import ee.datel.xtee.fault.ServiceFaultException;
import ee.xtee6.rr.rr67v1.Client;
import ee.xtee6.rr.rr67v1.RR67MuutusRequestType;
import ee.xtee6.rr.rr67v1.RR67MuutusResponseType;
import ee.xtee6.rr.rr67v1.RR67MuutusResponseType.TtKood.TtKoodid;
import ee.xtee6.rr.rr67v1.Service;
import ee.xtee6.rr.rr67v1.XRoadAdapterPortType;
import ee.xtee6.rr.rr67v1.XRoadService;
import java.time.LocalDateTime;
import java.util.Collections;
import java.util.List;
import jakarta.xml.ws.WebServiceException;
/**
*
Muudatuste koodid: (komaga eraldatud koodid, võivad puududa)
1-nimemuutus (ees või perenimi)
2-aadressi muutus (ELUKOHT)
3-isikukoodi muutus (vana väärtus olemas)
4-teovõime muutus
5-uus isikukood:sünd(vana väärtus puudub)
6-uus isikukood muul põhjusel(vana väärtus puudub)
7-surm
8-isikuttõendava dokumendi muutus (uus EV pass, EV diplomaatiline pass,välismaalase EV pass, ID-kaart,juhiluba,nende dok.väljaandmise aeg, kehtiv kuni, staatus)
9-isiku staatuse muutus
10-elamisloa andmete muutus(uus elamisluba, väljaandmise aeg, kehtiv kuni, staatus)
11-kodakondsus
12-isiku kirje staatus
22- Eesti kodakondsuse olemasolu (vana väärtus on Eesti või uus väärtus on Eesti)
23- Tähtajalise elamisõigus (lisandumine, kehtivusaja muutus)
24- Isiku staatuseks surnud (ainult need muudatused, kus isiku oleku uueks väärtuseks on SURNUD)
*
*
* @author aldoa
*
*/
public class RR67MuutusV1Service extends RrCommon {
private static XRoadService srv = new XRoadService();
private RR67MuutusV1Service() {}
public static List getNimeMuutused(RR67MuutusV1XRoadParams params, String userid, LocalDateTime alates,
LocalDateTime kuni, String issue) throws ServiceFaultException {
return getMuutused(params.getPort(), params.getClient(), params.getService(), userid, alates, kuni, issue, "1");
}
public static List getIsikMuutused(RR67MuutusV1XRoadParams params, String userid, LocalDateTime alates,
LocalDateTime kuni, String issue) throws ServiceFaultException {
return getMuutused(params.getPort(), params.getClient(), params.getService(), userid, alates, kuni, issue,
"1,2,3,7,9");
}
public static List getMuutused(XRoadAdapterPortType port, // NOSONAR
Client client, Service service, String userid, LocalDateTime alates, LocalDateTime kuni, String issue,
String muutus) throws ServiceFaultException {
RR67MuutusRequestType request = new RR67MuutusRequestType();
request.setCAlgKpv(alates.toLocalDate());
request.setCAlgKell(alates.toLocalTime());
request.setCLoppKpv(kuni.toLocalDate());
request.setCLoppKell(kuni.toLocalTime());
request.setCMuutused(muutus);
var id = ServiceUtils.getRequestId();
try {
RR67MuutusResponseType result =
port.rr67Muutus(request, client, service, userid, id, issue, ServiceUtils.getProtocolVersion());
if (result.getFaultCode() != null) {
if ("10010".equals(result.getFaultCode())) {
// 'Päring ei saanud vastust. Andmed puuduvad. (10010)'
return null; // NOSONAR
}
throw new ServiceFaultException(result.getFaultCode(), result.getFaultString(), id);
}
return result.getTtKood() == null ? Collections.emptyList() : result.getTtKood().getTtKoodids();
} catch (WebServiceException ex) {
throw new ServiceFaultException(ex.getClass().getSimpleName(), ex.getMessage(), id);
}
}
protected static Service getServiceIdentifier(String xroadInstance) {
return getServiceIdentifier(xroadInstance, memberClass, serviceCode, subsystemCode, "RR67_muutus", "v1");
}
protected static XRoadAdapterPortType getServicePort(String serverUrl) {
XRoadAdapterPortType port = srv.getMainPort();
ServiceUtils.connectPort(port, serverUrl);
return port;
}
protected static Client getClientIdentifier(String xroadInstance, String memberClass, String memberCode,
String subsystemCode) {
var client = new Client();
ServiceUtils.getXRoadIdentifierType(client, xroadInstance, memberClass, memberCode, subsystemCode);
return client;
}
protected static Service getServiceIdentifier(String xroadInstance, String memberClass, String memberCode,
String subsystemCode, String serviceCode, String serviceVersion) {
return ServiceUtils.getXRoadIdentifierType(new Service(), xroadInstance, memberClass, memberCode, subsystemCode,
serviceCode, serviceVersion);
}
public static class RR67MuutusV1XRoadParams {
private XRoadAdapterPortType port;
private Client client;
private Service service;
public RR67MuutusV1XRoadParams(String serverUrl, String xRoadInstance, String memberClass, String memberCode,
String subsystemCode) {
port = getServicePort(serverUrl);
client = getClientIdentifier(xRoadInstance, memberClass, memberCode, subsystemCode);
service = getServiceIdentifier(xRoadInstance);
}
public Service getService() {
return service;
}
public Client getClient() {
return client;
}
public XRoadAdapterPortType getPort() {
return port;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy