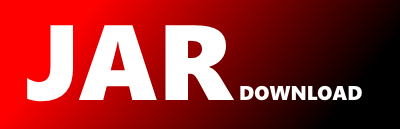
es.iti.wakamiti.api.plan.PlanNode Maven / Gradle / Ivy
The newest version!
/*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at https://mozilla.org/MPL/2.0/.
*/
package es.iti.wakamiti.api.plan;
import es.iti.wakamiti.api.model.ExecutableTreeNode;
import es.iti.wakamiti.api.util.Argument;
import java.util.*;
import java.util.function.Predicate;
/**
* Represents a node in a test plan.
*
* @author Luis Iñesta Gelabert - [email protected]
*/
public class PlanNode extends ExecutableTreeNode {
List description;
Set tags;
Map properties;
NodeType nodeType;
String language;
String id;
String source;
String keyword;
String name;
String displayName;
Optional data;
List arguments;
boolean filtered;
public PlanNode(NodeType nodeType, List children) {
super(children);
description = null;
tags = new LinkedHashSet<>();
properties = new LinkedHashMap<>();
this.nodeType = nodeType;
language = null;
id = null;
source = null;
keyword = null;
name = null;
displayName = null;
data = Optional.empty();
arguments = new LinkedList<>();
}
public String name() {
return name;
}
public String keyword() {
return keyword;
}
public String id() {
return id;
}
public String language() {
return language;
}
public NodeType nodeType() {
return nodeType;
}
public List description() {
return description;
}
public Set tags() {
return tags;
}
public String source() {
return source;
}
public Map properties() {
return properties;
}
public Optional data() {
return data;
}
public List arguments() {
return arguments;
}
public String displayName() {
return displayName;
}
public boolean filtered() {
return filtered;
}
public int numDescendants(NodeType nodeType) {
return numDescendants(descendant -> descendant.nodeType() == nodeType);
}
public int numDescendants(NodeType nodeType, Result result) {
return numDescendants(
descendant -> descendant.nodeType() == nodeType &&
descendant.executionState().isPresent() &&
descendant.executionState().get().hasResult(result)
);
}
public void resolveProperties(Predicate> filter) {
children().forEach(c -> c.resolveProperties(filter));
arguments.stream().map(Argument::evaluations)
.reduce((firstMap, secondMap) -> {
secondMap.forEach(firstMap::putIfAbsent);
return firstMap;
}).orElse(new HashMap<>())
.entrySet().stream()
.filter(filter)
.forEach(e -> {
data = data.map(d -> d.copyReplacingVariables(v -> v.replace(e.getKey(), e.getValue())));
name = name.replace(e.getKey(), e.getValue());
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy