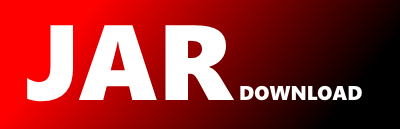
es.iti.wakamiti.api.plan.PlanNodeBuilder Maven / Gradle / Ivy
The newest version!
/*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at https://mozilla.org/MPL/2.0/.
*/
package es.iti.wakamiti.api.plan;
import es.iti.wakamiti.api.model.TreeNodeBuilder;
import java.util.*;
import java.util.stream.Collectors;
/**
* Builder class for creating instances of {@link PlanNode}.
*
* @author Luis Iñesta Gelabert - [email protected]
*/
public class PlanNodeBuilder extends TreeNodeBuilder {
private final List description = new ArrayList<>();
private final Set tags = new LinkedHashSet<>();
private final Map properties = new HashMap<>();
private NodeType nodeType;
private String language;
private String id;
private String source;
private String keyword;
private String name;
private String displayNamePattern = "[{id}] {keyword} {name}";
private PlanNodeData data;
private Object underlyingModel;
private boolean filtered;
public PlanNodeBuilder(NodeType nodeType) {
this.nodeType = nodeType;
}
public PlanNodeBuilder(NodeType nodeType, Collection children) {
super(children);
this.nodeType = nodeType;
}
public String name() {
return name;
}
public String keyword() {
return keyword;
}
public String id() {
return id;
}
public String language() {
return language;
}
public NodeType nodeType() {
return nodeType;
}
public List description() {
return description;
}
public Set tags() {
return tags;
}
public String source() {
return source;
}
public Map properties() {
return properties;
}
public String displayNamePattern() {
return displayNamePattern;
}
public boolean filtered() {
return filtered;
}
public String displayName() {
String displayName = displayNamePattern;
displayName = displayName.replace("{id}", id == null ? "" : id);
displayName = displayName.replace("{keyword}", keyword == null ? "" : keyword);
displayName = displayName.replace("{name}", name == null ? "" : name);
return displayName;
}
public Optional data() {
return Optional.ofNullable(data);
}
public Object getUnderlyingModel() {
return underlyingModel;
}
public PlanNodeBuilder setUnderlyingModel(Object gherkinModel) {
this.underlyingModel = gherkinModel;
return this;
}
public PlanNodeBuilder setId(String id) {
this.id = id;
return this;
}
public PlanNodeBuilder setKeyword(String keyword) {
this.keyword = keyword;
return this;
}
public PlanNodeBuilder setLanguage(String language) {
this.language = language;
return this;
}
public PlanNodeBuilder setName(String name) {
this.name = name;
return this;
}
public PlanNodeBuilder setNodeType(NodeType nodeType) {
this.nodeType = nodeType;
return this;
}
public PlanNodeBuilder setDisplayNamePattern(String displayNamePattern) {
this.displayNamePattern = displayNamePattern;
return this;
}
public PlanNodeBuilder setSource(String source) {
this.source = source;
return this;
}
public PlanNodeBuilder addTags(Collection tags) {
this.tags.addAll(tags);
return this;
}
public PlanNodeBuilder addDescription(Collection description) {
this.description.addAll(description);
return this;
}
public PlanNodeBuilder addProperties(Map properties) {
this.properties.putAll(properties);
return this;
}
public PlanNodeBuilder addProperty(String key, String value) {
this.properties.put(key, value);
return this;
}
public PlanNodeBuilder setData(PlanNodeData data) {
this.data = data;
return this;
}
public void filtered(boolean filtered) {
this.filtered = filtered;
}
@Override
public PlanNodeBuilder copy() {
return copy(new PlanNodeBuilder(nodeType));
}
@Override
protected PlanNodeBuilder copy(PlanNodeBuilder copy) {
copy.setLanguage(this.language);
copy.setId(this.id);
copy.setKeyword(this.keyword);
copy.setName(this.name);
copy.setDisplayNamePattern(this.displayNamePattern);
copy.addTags(this.tags);
copy.setSource(this.source());
copy.addDescription(this.description);
copy.properties.putAll(this.properties);
copy.setData(this.data().map(PlanNodeData::copy).orElse(null));
copy.filtered = this.filtered;
super.copy(copy);
return copy;
}
public PlanNode build() {
PlanNode node = new PlanNode(
this.nodeType(),
this.children().map(PlanNodeBuilder::build).collect(Collectors.toList()));
node.description = Collections.unmodifiableList(this.description());
node.tags = Collections.unmodifiableSet(this.tags());
node.properties = Collections.unmodifiableMap(this.properties());
node.language = this.language();
node.id = this.id();
node.source = this.source();
node.keyword = this.keyword();
node.name = this.name();
node.displayName = this.displayName();
node.data = this.data();
node.filtered = this.filtered;
return node;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy