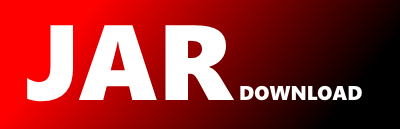
es.iti.wakamiti.api.util.Pair Maven / Gradle / Ivy
The newest version!
/*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at https://mozilla.org/MPL/2.0/.
*/
package es.iti.wakamiti.api.util;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.stream.Collector;
import java.util.stream.Collectors;
import static es.iti.wakamiti.api.util.MapUtils.entry;
/**
* A simple utility class representing a pair of values.
*
* @param The type of the key.
* @param The type of the value.
* @author Luis Iñesta Gelabert - [email protected]
*/
public class Pair {
private final T key;
private final U value;
public Pair(T key, U value) {
this.key = key;
this.value = value;
}
/**
* Computes the value of the Pair using the provided function.
*
* @param The type of the key.
* @param The type of the value.
* @param function The function to compute the value.
* @return A Function that computes the value for a given key.
*/
public static Function> computeValue(Function function) {
return key -> new Pair<>(key, function.apply(key));
}
/**
* Returns a Collector that accumulates elements into a LinkedHashMap.
*
* @param The type of the key.
* @param The type of the value.
* @return A Collector that accumulates elements into a LinkedHashMap.
*/
public static Collector, ?, Map> toMap() {
return Collector.of(
LinkedHashMap::new,
(m, e) -> m.put(e.key(), e.value()),
(m, r) -> m
);
}
/**
* Computes a Pair using the provided function.
*
* @param The type of the key.
* @param The type of the value.
* @param function The function to compute the value.
* @return A Function that computes a Pair for a given key.
*/
public static Function> compute(Function function) {
return first -> new Pair<>(first, function.apply(first));
}
/**
* Gets the key of the pair.
*
* @return The key.
*/
public T key() {
return key;
}
/**
* Gets the value of the pair.
*
* @return The value.
*/
public U value() {
return value;
}
/**
* Maps the Pair using the provided BiFunction.
*
* @param The type of the new key.
* @param The type of the new value.
* @param map The mapping function.
* @return A new Pair with mapped key and value.
*/
public Pair map(BiFunction> map) {
return map.apply(key, value);
}
public Map.Entry asEntry() {
return entry(key, value);
}
/**
* Maps each element of the Pair using the provided Function.
*
* @param The type of the new key and value.
* @param map The mapping function.
* @return A new Pair with mapped key and value.
*/
public Pair mapEach(Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy