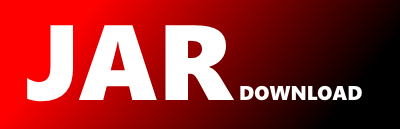
es.nitaur.bitbucket.rest.client.http.BitBucketWinHttpExecutor Maven / Gradle / Ivy
package es.nitaur.bitbucket.rest.client.http;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Maps;
import org.apache.http.Header;
import org.apache.http.StatusLine;
import org.apache.http.client.methods.*;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.WinHttpClients;
import org.apache.http.impl.conn.PoolingHttpClientConnectionManager;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.net.URI;
import java.util.Map;
public class BitBucketWinHttpExecutor implements BitBucketExecutor {
private static Logger LOGGER = LoggerFactory.getLogger(BitBucketHttpExecutor.class);
private CloseableHttpClient httpClient;
private String baseUrl;
public BitBucketWinHttpExecutor(String baseUrl) {
this.baseUrl = baseUrl;
PoolingHttpClientConnectionManager connectionManager = new PoolingHttpClientConnectionManager();
connectionManager.setMaxTotal(5);
connectionManager.setDefaultMaxPerRoute(4);
httpClient = WinHttpClients.createDefault();
}
public HttpResponse execute(HttpRequest httpRequest) throws BitBucketException {
HttpRequestBase request;
try {
request = createRequest(httpRequest);
org.apache.http.HttpResponse response = httpClient.execute(request);
StatusLine status = response.getStatusLine();
Map headers = toHeaderMultimap(response.getAllHeaders());
String body = null;
if (response.getEntity() != null) {
ByteArrayOutputStream out = new ByteArrayOutputStream(2048);
response.getEntity().writeTo(out);
body = new String(out.toByteArray(), "UTF-8");
}
return new HttpResponse(status.getStatusCode(), status.getReasonPhrase(), headers, body);
} catch (IOException e) {
throw new BitBucketException(e);
}
}
private Map toHeaderMultimap(Header[] headers) {
Map headerMap = Maps.newLinkedHashMap();
for (Header header : headers) {
String name = header.getName().toLowerCase();
String existingValue = headerMap.get(name);
headerMap.put(name, existingValue == null ? header.getValue() : existingValue + "," + header.getValue());
}
return ImmutableMap.copyOf(headerMap);
}
private HttpRequestBase createRequest(HttpRequest httpRequest) {
URI fullUri = URI.create(baseUrl + "/" + httpRequest.getUrl()).normalize();
String payload;
switch (httpRequest.getMethod()) {
case GET:
return new HttpGet(fullUri);
case POST:
HttpPost request = new HttpPost(fullUri);
payload = httpRequest.getPayload();
if (payload != null) {
request.setEntity(new StringEntity(payload, ContentType.APPLICATION_JSON));
}
return request;
case PUT:
HttpPut putRequest = new HttpPut(fullUri);
payload = httpRequest.getPayload();
if (payload != null) {
putRequest.setEntity(new StringEntity(payload, ContentType.APPLICATION_JSON));
}
return putRequest;
case DELETE:
return new HttpDelete(fullUri);
default:
throw new UnsupportedOperationException(String.format("http method %s is not supported", httpRequest.getMethod()));
}
}
public void shutdown() {
try {
httpClient.close();
} catch (IOException e) {
LOGGER.warn("exception when trying to close the http client", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy