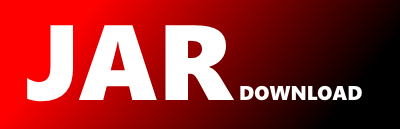
es.prodevelop.gvsig.mini.geom.impl.base.Extent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Geometries model for gvSIG Mini
The newest version!
/* SIGATEX. Gestión de Recursos Turísticos de Extremadura
* Funded by European Union. FEDER program.
*
* Copyright (C) 2008 Junta de Extremadura.
* Developed by: Prodevelop, S.L.
* For more information, contact:
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111- 1307,USA.
*
* For more information, contact:
*
* Junta de Extremadura
* Consejería de Cultura y Turismo
* C/ Almendralejo 14 Mérida
* 06800 Badajoz
* SPAIN
*
* Tel: +34 924007009
* http://www.culturaextremadura.com
*
* or
*
* Prodevelop, S.L.
* Pza. Juan de Villarrasa, 14 - 5
* Valencia
* Spain
* Tel: +34 963510612
* e-mail: [email protected]
* http://www.prodevelop.es
*
*/
package es.prodevelop.gvsig.mini.geom.impl.base;
import java.util.StringTokenizer;
import es.prodevelop.gvsig.mini.geom.impl.base.Extent;
import es.prodevelop.gvsig.mini.geom.impl.base.Point;
/**
* This class represents a minimum bounding rectangle
*
* @author Alberto Romeu Carrasco ([email protected])
* @author aromeu - modified for gvSIG phone
*/
public class Extent {
private double minX;
private double minY;
private double maxX;
private double maxY;
private Point center = null;
public Extent() {
}
/**
* Constructor. It also sets the center according to the parameters.
*
* @param minX
* The minimum x value
* @param minY
* The minimum y value
* @param maxX
* The maximum x value
* @param maxY
* The maximum y value
*/
public Extent(final double minX, final double minY, final double maxX,
final double maxY) {
this.minX = minX;
this.minY = minY;
this.maxX = maxX;
this.maxY = maxY;
center = new Point(0, 0);
this.calculateCenter();
}
/**
* Constructor. It also sets the center according to the parameters
*
* @param leftBottom
* A Point
with the minimum x and y values
* @param rightTop
* A Point
with the maximum x and y values
*/
public Extent(final Point leftBottom, final Point rightTop) {
this.minX = leftBottom.getX();
this.minY = leftBottom.getY();
this.maxX = rightTop.getX();
this.maxY = rightTop.getY();
center = new Point(0, 0);
this.calculateCenter();
}
/**
* sets to NULL the center Point
*/
public void destroy() {
this.center = null;
}
/**
* Clones this instance
*
* @return a cloned instance of this Extent
*/
public Object clone() {
return new Extent(this.minX, this.minY, this.maxX, this.maxY);
}
/**
* Compares this extent whith other
*
* @param extent
* The extent to compare
* @return true if both are equals
*/
public boolean equals(final Extent extent) {
return (this.minX == extent.minX && this.maxX == extent.maxX
&& this.minY == extent.minY && this.maxY == extent.maxY);
}
/**
* Checks if the extent contains the x and y values
*
* @param x
* X value
* @param y
* Y value
* @return true if contains
*/
public boolean contains(final double x, final double y) {
return ((x >= this.minX) && (x <= this.maxX) && (y >= this.minY) && (y <= this.maxY));
}
/**
* Checks if the extent contains the x and y values
*
* @param point
* an array with x and y values
* @return true if contains
*/
public boolean contains(final double[] point) {
if (point == null) return false;
return ((point[0] >= this.minX) && (point[0] <= this.maxX)
&& (point[1] >= this.minY) && (point[1] <= this.maxY));
}
/**
* Check if the extents contains the Point
*
* @param point
* The point to check
* @return true if contains
*/
public boolean contains(final Point point) {
return this.contains(point.getX(), point.getY());
}
/**
* Checks if the extent intersects with other
*
* @param extent
* The extent to check
* @return true if intersects
*/
public boolean intersect(final Extent extent) {
final boolean inBottom = (extent.minY == this.minY && extent.maxY == this.maxY) ? true
: (((extent.minY > this.minY) && (extent.minY < this.maxY)) || ((this.minY > extent.minY) && (this.minY < extent.maxY)));
final boolean inTop = (extent.minY == this.minY && extent.maxY == this.maxY) ? true
: (((extent.maxY > this.minY) && (extent.maxY < this.maxY)) || ((this.maxY > extent.minY) && (this.maxY < extent.maxY)));
final boolean inRight = (extent.maxX == this.maxX && extent.minX == this.minX) ? true
: (((extent.maxX > this.minX) && (extent.maxX < this.maxX)) || ((this.maxX > extent.minX) && (this.maxX < extent.maxX)));
final boolean inLeft = (extent.maxX == this.maxX && extent.minX == this.minX) ? true
: (((extent.minX > this.minX) && (extent.minX < this.maxX)) || ((this.minX > extent.minX) && (this.minX < extent.maxX)));
final boolean res = (this.contains(extent) || extent.contains(this) || ((inTop || inBottom) && (inLeft || inRight)));
return res;
}
/**
* Check if the extent intersects and returns an extent that represent the
* area that intersects
*
* @param extent
* The extent to check
* @param ext
* true or false it doesn't matter
* @return The intersection extent. NULL if the extents don't intersect
*/
public Extent intersect(final Extent extent, final boolean ext) {
if (this.intersect(extent)) {
return new Extent(Math.max(extent.getMinX(), this.getMinX()),
Math.max(extent.getMinY(), this.getMinY()), Math.min(
extent.getMaxX(), this.getMaxX()), Math.min(
extent.getMaxY(), this.getMaxY()));
}
return null;
}
/**
* Checks if the extent contains the other
*
* @param extent
* The extent to check
* @return true if contains
*/
public boolean contains(final Extent extent) {
final boolean inLeft = (extent.minX >= this.minX)
&& (extent.minX <= this.maxX);
final boolean inTop = (extent.maxY >= this.minY)
&& (extent.maxY <= this.maxY);
final boolean inRight = (extent.maxX >= this.minX)
&& (extent.maxX <= this.maxX);
final boolean inBottom = (extent.minY >= this.minY)
&& (extent.minY <= this.maxY);
return (inTop && inLeft && inBottom && inRight);
}
/**
* Calculates the area of the extent
*
* @return The area
*/
public double area() {
return this.getWidth() * this.getHeight();
}
private void calculateCenter() {
this.center.setX((minX + maxX) / 2);
this.center.setY((minY + maxY) / 2);
}
/**
*
* @return An instance of a Point with the minimum x and y values
*/
public Point getLefBottomCoordinate() {
return new Point(this.minX, this.minY);
}
/**
*
* @return * @return An instance of a Point with the maximum x and y values
*/
public Point getRightTopCoordinate() {
return new Point(this.maxX, this.maxY);
}
/**
* Sets the minimum x and y values
*
* @param leftBottom
* A Point with the x and y values to set
*/
public void setLeftBottomCoordinate(final Point leftBottom) {
this.minX = leftBottom.getX();
this.minY = leftBottom.getY();
}
/**
* Sets the maximum x and y values
*
* @param rightTop
* A Point with the x and y values to set
*/
public void setRightTopCoordinate(final Point rightTop) {
this.maxX = rightTop.getX();
this.maxY = rightTop.getY();
}
/**
* This method is used to get a printable String with the x,y values of the
* extent
*
* @return a String with the minimum and maximum x,y values of the extent in
* this format: "minX= 0.0, minY= 0.0, maxX= 1.0, maxY= 1.0"
*/
public String toPrintString() {
return new StringBuffer().append("minX= ").append(this.minX)
.append(", minY= ").append(this.minY).append(", maxX= ")
.append(this.maxX).append(", maxY= ").append(this.maxY)
.toString();
}
/**
* This methos is used to build the URL of a tile request
*
* @return a String with the minimum and maximum x,y values of the extent in
* this format: "0.0, 0.0, 1.0, 1.0"
*/
public String toString() {
return toString(",");
}
/**
* This method is used to build the URL of a tile request
*
* @param separator
* The string separator between coordinates
* @return a String with the minimum and maximum x,y values of the extent in
* this format: "0.0 separator 0.0 separator 1.0 separator 1.0"
*/
public String toString(String separator) {
return new StringBuffer().append(this.minX).append(separator)
.append(this.minY).append(separator).append(this.maxX)
.append(separator).append(this.maxY).toString();
}
/**
* This methos is used to build the URL of a tile request
*
* @return a StringBuffer with the minimum and maximum x,y values of the
* extent in this format: "0.0, 0.0, 1.0, 1.0"
*/
public StringBuffer toStringBuffer() {
StringBuffer s = new StringBuffer();
s.append(this.minX).append(",").append(this.minY).append(",")
.append(this.maxX).append(",").append(this.maxY);
return s;
}
/**
* Calculates the width of the extent
*
* @return The width of the extent
*/
public double getWidth() {
return Math.abs(maxX - minX);
}
/**
* Calculates the height of the extent
*
* @return The height of the extent
*/
public double getHeight() {
return Math.abs(maxY - minY);
}
/**
* @return the minX
*/
public double getMinX() {
return minX;
}
/**
* @param minX
* the minX to set
*/
public void setMinX(final double minX) {
this.minX = minX;
}
/**
* @return the minY
*/
public double getMinY() {
return minY;
}
/**
* @param minY
* the minY to set
*/
public void setMinY(final double minY) {
this.minY = minY;
}
/**
* @return the maxX
*/
public double getMaxX() {
return maxX;
}
/**
* @param maxX
* the maxX to set
*/
public void setMaxX(final double maxX) {
this.maxX = maxX;
}
/**
* @return the maxY
*/
public double getMaxY() {
return maxY;
}
/**
* @param maxY
* the maxY to set
*/
public void setMaxY(final double maxY) {
this.maxY = maxY;
}
/**
* @return the center
*/
public Point getCenter() {
this.calculateCenter();
return center;
}
/**
* @param center
* the center to set
*/
public void setCenter(final Point center) {
this.center = center;
}
/**
* parses a String into a Extent. The String should have the same format as
* the method toString() returns. Uses as separator the string ","
*
* @param extent
* @return
*/
public static Extent parseString(final String extent) {
return parseString(extent, ",");
}
/**
* parses a String into a Extent. The String should have the same format as
* the method toString() returns.
*
* @param extent
* @param separator
* The string separator between coordinates
* @return
*/
public static Extent parseString(final String extent, String separator) {
StringTokenizer s = null;
try {
s = new StringTokenizer(extent, separator);
if (s.countTokens() < 4)
return null;
return new Extent(Double.parseDouble(s.nextToken()),
Double.parseDouble(s.nextToken()), Double.parseDouble(s
.nextToken()), Double.parseDouble(s.nextToken()));
} catch (final Exception e) {
e.printStackTrace();
return null;
} finally {
s = null;
}
}
public boolean isValid() {
boolean isValid = (this.minX <= this.maxX) && (this.minY <= this.maxY)
&& area() > 0;
return isValid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy