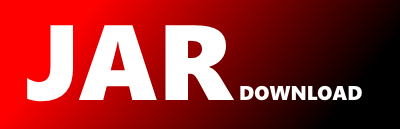
es.prodevelop.gvsig.mini.geom.impl.base.Feature Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Geometries model for gvSIG Mini
The newest version!
/* gvSIG. Geographic Information System of the Valencian Government
*
* Copyright (C) 2007-2008 Infrastructures and Transports Department
* of the Valencian Government (CIT)
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
* MA 02110-1301, USA.
*
*/
/*
* Prodevelop, S.L.
* Pza. Juan de Villarrasa, 14 - 5
* Valencia
* Spain
* Tel: +34 963510612
* e-mail: [email protected]
* http://www.prodevelop.es
*
*/
package es.prodevelop.gvsig.mini.geom.impl.base;
import java.util.ArrayList;
import java.util.HashMap;
import es.prodevelop.gvsig.mini.exceptions.BaseException;
import es.prodevelop.gvsig.mini.geom.api.IFeature;
import es.prodevelop.gvsig.mini.geom.api.IGeometry;
/**
* An abstraction of an entity in the real world
*
* @author Alberto Romeu Carrasco ([email protected])
*/
public class Feature implements IFeature {
private IGeometry geometry;
private boolean visible = true;
private HashMap fields = new HashMap();
private String text;
private String SRS;
/**
* The constructor.
*
* @param geometry
* A geometry
*/
public Feature(final IGeometry geometry) {
this.geometry = geometry;
}
/*
* (non-Javadoc)
*
* @see es.prodevelop.gvsig.mini.geom.impl.IFeature#isVisible()
*/
public boolean isVisible() {
return visible;
}
/*
* (non-Javadoc)
*
* @see es.prodevelop.gvsig.mini.geom.impl.IFeature#setVisible(boolean)
*/
public void setVisible(final boolean visible) {
this.visible = visible;
if (this.geometry != null) {
this.geometry.setVisible(visible);
}
}
/*
* (non-Javadoc)
*
* @see es.prodevelop.gvsig.mini.geom.impl.IFeature#getGeometry()
*/
public IGeometry getGeometry() {
return geometry;
}
/*
* (non-Javadoc)
*
* @see
* es.prodevelop.gvsig.mini.geom.impl.IFeature#setGeometry(es.prodevelop
* .gvsig.mini.geom.IGeometry)
*/
public void setGeometry(final IGeometry geometry) {
this.geometry = geometry;
}
public String getSRS() {
return SRS;
}
public void setSRS(String sRS) {
SRS = sRS;
}
/*
* (non-Javadoc)
*
* @see es.prodevelop.gvsig.mini.geom.impl.IFeature#destroy()
*/
public void destroy() {
try {
this.geometry.destroy();
this.geometry = null;
} catch (final Exception e) {
e.printStackTrace();
}
}
public void addField(String key, String value, int type) {
fields.put(key, new Attribute(value, type));
}
public void addField(String key, Attribute attr) {
fields.put(key, attr);
}
public void addField(Attribute attr) throws BaseException {
if (attr.key != null)
fields.put(attr.key, attr);
else
throw new BaseException("No key set for attribute");
}
public Attribute getAttribute(String key) {
return (Attribute) fields.get(key);
}
public HashMap getAttributes() {
return fields;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public void addFields(String[] keys, String[] values,
HashMap fieldTypes) {
if (keys != null && values != null && fieldTypes != null) {
final int length = keys.length;
if (length != values.length || length != fieldTypes.size())
return;
String key;
for (int i = 0; i < length; i++) {
key = keys[i];
if (key != null)
this.addField(key, values[i], fieldTypes.get(key));
}
}
}
/**
* Get the value object associated with a key.
*
* @param key
* A key string.
* @return The object associated with the key.
* @throws BaseException
* if the key is not found.
*/
public Object get(String key) throws BaseException {
Object o = opt(key);
if (o == null) {
throw new BaseException(key + " not found.");
}
return o;
}
/**
* Get the string associated with a key.
*
* @param key
* A key string.
* @return A string which is the value.
* @throws BaseException
* if the key is not found.
*/
public String getString(String key) throws BaseException {
return get(key).toString();
}
/**
* Get an optional value associated with a key.
*
* @param key
* A key string.
* @return An object which is the value, or null if there is no value.
*/
public Object opt(String key) {
return key == null ? null : this.fields.get(key);
}
/**
* Get the boolean value associated with a key.
*
* @param key
* A key string.
* @return The truth.
* @throws BaseException
* if the value is not a Boolean or the String "true" or
* "false".
*/
public boolean getBoolean(String key) throws BaseException {
Object o = get(key);
if (o.equals(Boolean.FALSE)
|| (o instanceof String && ((String) o)
.equalsIgnoreCase("false"))) {
return false;
} else if (o.equals(Boolean.TRUE)
|| (o instanceof String && ((String) o)
.equalsIgnoreCase("true"))) {
return true;
}
throw new BaseException(key + "is not a Boolean.");
}
/**
* Get the double value associated with a key.
*
* @param key
* A key string.
* @return The numeric value.
* @throws BaseException
* if the key is not found or if the value is not a Number
* object and cannot be converted to a number.
*/
public double getDouble(String key) throws BaseException {
Object o = get(key);
try {
return o instanceof Number ? ((Number) o).doubleValue() : Double
.valueOf((String) o).doubleValue();
} catch (Exception e) {
throw new BaseException(key + " is not a number.");
}
}
/**
* Get the int value associated with a key. If the number value is too large
* for an int, it will be clipped.
*
* @param key
* A key string.
* @return The integer value.
* @throws BaseException
* if the key is not found or if the value cannot be converted
* to an integer.
*/
public int getInt(String key) throws BaseException {
Object o = get(key);
return o instanceof Number ? ((Number) o).intValue()
: (int) getDouble(key);
}
public static class Attribute {
public final static int TYPE_UNKNOWN = -1;
public final static int TYPE_STRING = 0;
public final static int TYPE_INT = 1;
public final static int TYPE_DOUBLE = 2;
public final static int TYPE_LIST_STRING = 3;
public final static int TYPE_LIST_INT = 4;
public final static int TYPE_LIST_DOUBLE = 5;
public final static int TYPE_URL = 6;
public final static int TYPE_PHONE = 7;
public final static int TYPE_DATE = 8;
public final static int TYPE_IMG = 9;
public final static int TYPE_IMG_URL = 10;
public int type = TYPE_UNKNOWN;
public String value;
public String key = null;
public Attribute parentAttribute = null;
public ArrayList attributes = new ArrayList();
public Attribute(String value, int type) {
this.value = value;
this.type = type;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy