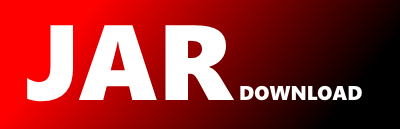
es.prodevelop.gvsig.mini.geom.impl.base.FeatureCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Geometries model for gvSIG Mini
The newest version!
/* gvSIG. Geographic Information System of the Valencian Government
*
* Copyright (C) 2007-2008 Infrastructures and Transports Department
* of the Valencian Government (CIT)
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
* MA 02110-1301, USA.
*
*/
/*
* Prodevelop, S.L.
* Pza. Juan de Villarrasa, 14 - 5
* Valencia
* Spain
* Tel: +34 963510612
* e-mail: [email protected]
* http://www.prodevelop.es
*
*/
package es.prodevelop.gvsig.mini.geom.impl.base;
import java.util.Vector;
import es.prodevelop.gvsig.mini.geom.api.IFeature;
import es.prodevelop.gvsig.mini.geom.impl.base.Feature;
/**
* A Collection of features
* @author Alberto Romeu Carrasco ([email protected])
*/
public class FeatureCollection {
private Vector features;
private boolean visible = true;
/**
* The constructor. Initializes the vector of features.
*/
public FeatureCollection() {
features = new Vector();
}
/**
*
* @return true if is visible
*/
public boolean isVisible() {
return visible;
}
/**
* Sets this instance to visible or not and its features and the geometries
* of every feature.
* @param visible
*/
public void setVisible(final boolean visible) {
this.visible = visible;
if (features == null) return;
final Vector features = this.features;
final int size = features.size();
IFeature f;
for (int i = 0; i < size; i++) {
f = ((IFeature) features.elementAt(i));
if (f != null) {
f.setVisible(visible);
}
}
}
/**
*
* @return The vector of features
*/
public Vector getFeatures() {
return features;
}
/**
* Sets the features
* @param features
*/
public void setFeatures(final Vector features) {
this.features = features;
}
/**
* Adds a feature to the collection
* @param f A feature
*/
public void addFeature(final Feature f) {
if (features == null) {
features = new Vector();
}
this.features.addElement(f);
}
/**
* Deletes the feature from the collection
* @param f A feature
*/
public void removeFeature(final Feature f) {
if (features != null) {
this.features.removeElement(f);
}
}
/**
* Deletes a feature from the collection in the specified index
* @param index The index of the feature in the collection
*/
public void removeFeature(final int index) {
try {
final Vector features = this.features;
if (features != null && index >= 0) {
if (features.size() > index) {
features.removeElementAt(index);
}
}
} catch (final Exception e) {
e.printStackTrace();
}
}
/**
* Clears this instance
*/
public void destroy() {
final Vector features = this.features;
if (features != null) {
final int size = features.size();
IFeature f;
for (int i = 0; i < size; i++) {
f = ((IFeature) features.elementAt(i));
if(f != null)
f.destroy();
}
this.features = null;
}
}
/**
*
* @param index THe index of a feature in the collection
* @return A feature in the specified index
*/
public IFeature getFeatureAt(final int index) {
try {
if (features != null) {
if (features.size() > index && index >= 0) {
return (IFeature) features.elementAt(index);
}
}
return null;
} catch (final Exception e) {
e.printStackTrace();
return null;
}
}
/**
*
* @return The length of the collection
*/
public int getSize() {
if (features != null) {
return features.size();
} else {
return 0;
}
}
/**
* Inserts a Feature at the specified index
* @param o A Feature
* @param index The index where to insert
*/
public void insertElementAt(final Object o, final int index) {
if (features == null) {
features = new Vector();
}
this.features.insertElementAt(o, index);
}
/**
* Replace The feature on the specified index
* @param o The Feature
* @param index The index where set the feature
*/
public void setElementAt(final Object o, final int index) {
if (features == null) {
features = new Vector();
}
this.features.setElementAt(o, index);
}
/**
* Clears the collection of features
*/
public void removeAll() {
if (features != null) {
this.features.removeAllElements();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy