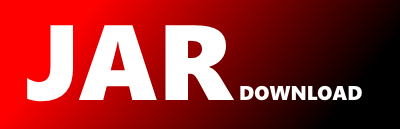
es.prodevelop.gvsig.mini.geom.impl.base.LineString Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Geometries model for gvSIG Mini
The newest version!
/* gvSIG. Geographic Information System of the Valencian Government
*
* Copyright (C) 2007-2008 Infrastructures and Transports Department
* of the Valencian Government (CIT)
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
* MA 02110-1301, USA.
*
*/
/*
* Prodevelop, S.L.
* Pza. Juan de Villarrasa, 14 - 5
* Valencia
* Spain
* Tel: +34 963510612
* e-mail: [email protected]
* http://www.prodevelop.es
*
*/
package es.prodevelop.gvsig.mini.geom.impl.base;
import com.vividsolutions.jts.geom.Geometry;
import es.prodevelop.gvsig.mini.exceptions.BaseException;
import es.prodevelop.gvsig.mini.geom.api.IGeometry;
/**
* A LineString. Usually used to represent routes
* @author Alberto Romeu Carrasco ([email protected])
*/
public class LineString implements IGeometry {
/**
* An array with the x coordinates
*/
private double[] xCoords;
/**
* An array with the x coordinates
*/
private double[] yCoords;
/**
* True if it should be drawn on the screen
*/
private boolean visible = true;
/**
* The constructor
* @param xCoords An array with the x coordinates
* @param yCoords An array with the y coordinates
*/
public LineString(final double[] xCoords, final double[] yCoords) {
this.xCoords = xCoords;
this.yCoords = yCoords;
}
/**
* The constructor
* @param points An array of points to fill the x and y coordinates
*/
public LineString(final Point[] points) {
final int size = points.length;
try {
double[] xCoords = new double[size];
double[] yCoords = new double[size];
for (int i = 0; i < size; i++) {
if (points[i] != null) {
xCoords[i] = points[i].getX();
yCoords[i] = points[i].getY();
}
}
this.xCoords = xCoords;
this.yCoords = yCoords;
} catch (final Exception e) {
e.printStackTrace();
}
}
/**
* Clears this instance
*/
public void destroy() {
this.xCoords = null;
this.yCoords = null;
}
/**
*
* @return True if is visible
*/
public boolean isVisible() {
return visible;
}
/**
* Sets this instance to visible or not
* @param visible
*/
public void setVisible(final boolean visible) {
this.visible = visible;
}
/**
*
* @return The array of x coordinates
*/
public double[] getXCoords() {
return xCoords;
}
/**
* Sets the array of x coordinates
* @param xCoords
*/
public void setXCoords(final double[] xCoords) {
this.xCoords = xCoords;
}
/**
*
* @return The array of y coordinates
*/
public double[] getYCoords() {
return yCoords;
}
/**
* Sets the array of y coordinates
* @param yCoords
*/
public void setYCoords(final double[] yCoords) {
this.yCoords = yCoords;
}
/**
*
* @return An array of points with x and y coordinates
*/
public Point[] getCoordinates() {
Point[] coordinates = new Point[this.xCoords.length];
final int size = this.xCoords.length;
final double[] xCoords = this.xCoords;
final double[] yCoords = this.yCoords;
for (int i = 0; i < size; i++) {
coordinates[i] = new Point(xCoords[i], yCoords[i]);
}
return coordinates;
}
/**
* Sets the x and y coordinates of the LineString
* @param coordinates An array of points
*/
public void setCoordinates(final Point[] coordinates) {
try {
final int size = coordinates.length;
Point p;
double[] xCoords = new double[size];
double[] yCoords = new double[size];
for (int i = 0; i < size; i++) {
p = coordinates[i];
if (p != null) {
xCoords[i] = p.getX();
yCoords[i] = p.getY();
}
}
this.xCoords = xCoords;
this.yCoords = yCoords;
} catch (final Exception e) {
e.printStackTrace();
}
}
public Geometry getGeometry() throws BaseException {
throw new BaseException("No JTS Geometry");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy