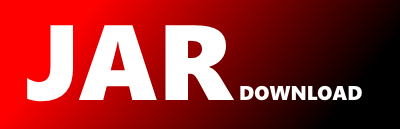
es.prodevelop.gvsig.mini.geom.impl.base.MultiLineString Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Geometries model for gvSIG Mini
The newest version!
/* gvSIG. Geographic Information System of the Valencian Government
*
* Copyright (C) 2007-2008 Infrastructures and Transports Department
* of the Valencian Government (CIT)
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
* MA 02110-1301, USA.
*
*/
/*
* Prodevelop, S.L.
* Pza. Juan de Villarrasa, 14 - 5
* Valencia
* Spain
* Tel: +34 963510612
* e-mail: [email protected]
* http://www.prodevelop.es
*
*/
package es.prodevelop.gvsig.mini.geom.impl.base;
import com.vividsolutions.jts.geom.Geometry;
import es.prodevelop.gvsig.mini.exceptions.BaseException;
import es.prodevelop.gvsig.mini.geom.api.IGeometry;
import es.prodevelop.gvsig.mini.geom.impl.base.LineString;
import es.prodevelop.gvsig.mini.geom.impl.base.Point;
/**
* An array of LineString
* @author Alberto Romeu Carrasco ([email protected])
*/
public class MultiLineString implements IGeometry {
private LineString[] lineStrings;
private boolean visible = true;
/**
* The constructor
* @param lineStrings An array of lineStrings
*/
public MultiLineString(final LineString[] lineStrings) {
this.lineStrings = lineStrings;
}
/**
* Clears this instance
*/
public void destroy() {
final int size = lineStrings.length;
final LineString[] lineStrings = this.lineStrings;
for (int i = 0; i < size; i++) {
lineStrings[i].destroy();
}
this.lineStrings = null;
}
/**
*
* @return True if is visible
*/
public boolean isVisible() {
return visible;
}
/**
* Sets this instance to visible or not and its array of LineString
* @param visible
*/
public void setVisible(final boolean visible) {
this.visible = visible;
final int size = this.lineStrings.length;
final LineString[] lineStrings = this.lineStrings;
for (int i = 0; i < size; i++) {
lineStrings[i].setVisible(visible);
}
}
/**
*
* @return The array of LineString
*/
public LineString[] getLineStrings() {
return lineStrings;
}
/**
* Sets the array of LineString
* @param lineStrings An array of LineString
*/
public void setLineStrings(final LineString[] lineStrings) {
this.lineStrings = lineStrings;
}
/**
*
* @return An array of points with the coordinates of the array of LineString
*/
public Point[] getCoordinates() {
int size = 0;
final LineString[] lineStrings = this.lineStrings;
final int length = lineStrings.length;
for (int i = 0; i < length; i++) {
size += lineStrings[i].getXCoords().length;
}
Point[] coordinates = new Point[size];
LineString line;
for (int h = 0; h < length; h++) {
line = lineStrings[h];
final int l = line.getXCoords().length;
for (int j = 0; j < l; j++) {
coordinates[h] = new Point(line.getXCoords()[j], line.getYCoords()[j]);
}
}
return coordinates;
}
/**
* Gets the last LineString and retrieves the x-y coordinates in the
* position specified
* @param pos The position of the coordinates to retrieve
* @return A Point with the coordinates
*/
public Point getLastCoordinate(final int pos) {
final LineString l = this.lineStrings[this.lineStrings.length - 1];
double[] coord = l.getXCoords();
final double x = coord[coord.length - pos];
coord = l.getYCoords();
final double y = coord[coord.length - pos];
return new Point(x, y);
}
/**
* Gets the first LineString and retrieves the x-y coordinates in the
* position specified
* @param pos The position of the coordinates to retrieve
* @return A Point with the coordinates
*/
public Point getFirstCoordinate(final int pos) {
final LineString l = this.lineStrings[0];
double[] coord = l.getXCoords();
final double x = coord[pos];
coord = l.getYCoords();
final double y = coord[pos];
return new Point(x, y);
}
/**
* Does nothing
* @param coordinates
*/
public void setCoordinates(final Point[] coordinates) {
}
public Geometry getGeometry() throws BaseException {
throw new BaseException("No JTS Geometry");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy