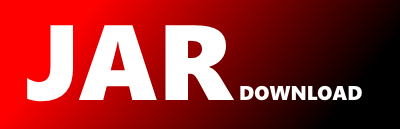
es.prodevelop.gvsig.mini.geom.impl.base.Point Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Geometries model for gvSIG Mini
The newest version!
/* gvSIG. Geographic Information System of the Valencian Government
*
* Copyright (C) 2007-2008 Infrastructures and Transports Department
* of the Valencian Government (CIT)
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
* MA 02110-1301, USA.
*
*/
/*
* Prodevelop, S.L.
* Pza. Juan de Villarrasa, 14 - 5
* Valencia
* Spain
* Tel: +34 963510612
* e-mail: [email protected]
* http://www.prodevelop.es
*
*/
package es.prodevelop.gvsig.mini.geom.impl.base;
import com.vividsolutions.jts.geom.Geometry;
import bm.core.tools.StringTokenizer;
import es.prodevelop.gvsig.mini.exceptions.BaseException;
import es.prodevelop.gvsig.mini.geom.api.IGeometry;
import es.prodevelop.gvsig.mini.geom.impl.base.Point;
import es.prodevelop.gvsig.mini.utiles.Utilities;
/**
* This class represents a Point with x and y coordinates of the real world
*
* @author Alberto Romeu Carrasco ([email protected])
*/
public class Point implements IGeometry {
private double x;
private double y;
private boolean visible = true;
public Point() {
}
/**
* The constructor
*
* @param x
* X Coordinate
* @param y
* Y coordinate
*/
public Point(final double x, final double y) {
this.x = x;
this.y = y;
}
/**
* The constructor
*
* @param coords
* An array with the x and y coordinate
*/
public Point(final double[] coords) {
this.x = coords[0];
this.y = coords[1];
}
/**
* Does nothing
*/
public void destroy() {
}
/**
* True if is visible
*
* @return
*/
public boolean isVisible() {
return visible;
}
/**
* Sets to visible or not
*
* @param visible
*/
public void setVisible(final boolean visible) {
this.visible = visible;
}
/**
*
* @return A new instance of this Point
*/
public Object clone() {
return new Point(this.x, this.y);
}
/**
* Compares the coordinates of two Points
*
* @param point
* The point to be compared
* @return True if the coordinates are the same
*/
public boolean equals(final Point point) {
return (this.x == point.x && this.y == point.y);
}
/**
* Adds the x and y values to the x and y coordinates of this instance
*
* @param x
* The x coordinate to add
* @param y
* The y coordinate to add
* @return A new Point
*/
public Point add(final double x, final double y) {
return new Point(this.x + x, this.y + y);
}
/**
* Substracts the x and y values from the x and y coordinates of this
* instance
*
* @param x
* The x coordinate to substract
* @param y
* The y coordinate to substract
* @return A new Point
*/
public Point sub(final double x, final double y) {
return new Point(this.x - x, this.y - y);
}
/**
* Adds the x and y coordinates of this instance and the one specified
*
* @param point
* The Point to add
* @return A new Point
*/
public Point add(final Point point) {
return new Point(this.x + point.getX(), this.y + point.getY());
}
/**
* Substract the x and y coordinates of the specified pixel from this
* instance
*
* @param point
* The point to substract
* @return A new Point
*/
public Point sub(final Point point) {
return new Point(this.x - point.getX(), this.y - point.getY());
}
/**
*
* @return A new point with the abs values of the coordinates of this
* instance
*/
public Point abs() {
return new Point(Math.abs(this.x), Math.abs(this.y));
}
public String toString() {
return new StringBuffer().append(this.getX()).append(",")
.append(this.getY()).toString();
}
public String toShortString(int length) {
String x = "";
String y = "";
x = Utilities.trimDecimals(String.valueOf(this.getX()), length);
y = Utilities.trimDecimals(String.valueOf(this.getY()), length);
return new StringBuffer().append(x).append(",").append(y).toString();
}
/**
* @return the x
*/
public double getX() {
return x;
}
/**
* @param x
* the x to set
*/
public void setX(final double x) {
this.x = x;
}
/**
* @return the y
*/
public double getY() {
return y;
}
/**
* @param y
* the y to set
*/
public void setY(final double y) {
this.y = y;
}
/**
* Calculates the distance from this Point to another
*
* @param p
* The Point to calculate the distance
* @return The distance
*/
public double distance(final Point p) {
double a1 = (x - p.getX()) * (x - p.getX());
// if (DEBUG)
// System.out.println("a1: " + a1);
//
double a2 = (y - p.getY()) * (y - p.getY());
// if (DEBUG)
// System.out.println("a2: " + a2);
//
double a3 = a1 + a2;
// if (DEBUG)
// System.out.println("a3: " + a3);
//
if (a3 < 0)
return -1;
double res = (double) (Math.sqrt(a3));
// if (DEBUG)
// System.out.println("res: " + res);
//
return res;
}
/**
* Calculates the distance from this Point to the coordinates of the array
*
* @param xy
* X and y coordinates to calculate the distance
* @return The distance
*/
public double distance(final double[] xy) {
return this.distance(new Point(xy[0], xy[1]));
}
/**
* Calculates the distance from this Point to the coordinates
*
* @param x
* x coordinate
* @param y
* y coordinate
* @return The distance
*/
public double distance(final double x, final double y) {
return this.distance(new Point(x, y));
}
/**
*
* @return An array with one Point with the coordinates of this instance
*/
public Point[] getCoordinates() {
return new Point[] { this };
}
/**
* parses a String into a Point. The String should have the same format as
* the method toString() returns.
*
* @param extent
* @return
*/
public static Point parseString(final String point) {
StringTokenizer st;
try {
st = new StringTokenizer(point, ",");
if (st.length < 2) {
return null;
}
return new Point(Double.parseDouble(st.nextToken()),
Double.parseDouble(st.nextToken()));
} catch (final Exception e) {
e.printStackTrace();
return null;
} finally {
st = null;
}
}
/**
* Sets the x y coordinates of this instance
*
* @param coords
* An array with one Point containing the coordinates
*/
public void setCoordinates(final Point[] coords) {
try {
if (coords != null) {
this.x = coords[0].x;
this.y = coords[0].y;
}
} catch (final Exception e) {
e.printStackTrace();
}
}
/**
* Sets the xy coordinates of this instance
*
* @param coords
* An array with the coordinates
*/
public void setCoordinates(final double[] coords) {
try {
this.x = coords[0];
this.y = coords[1];
} catch (final Exception e) {
e.printStackTrace();
}
}
public boolean isValid() {
return this.getX() != 0 && this.getY() != 0;
}
public Geometry getGeometry() throws BaseException {
throw new BaseException("No JTS Geometry");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy