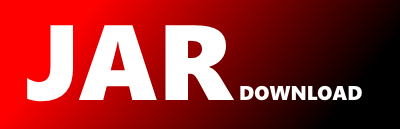
es.prodevelop.gvsig.mini.geom.impl.jts.JTSFeature Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Show all versions of es.prodevelop.gvsig.mini.geom Show documentation
Geometries model for gvSIG Mini
The newest version!
package es.prodevelop.gvsig.mini.geom.impl.jts;
import java.util.ArrayList;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.Geometry;
import com.vividsolutions.jts.geom.GeometryCollection;
import com.vividsolutions.jts.geom.GeometryFactory;
import com.vividsolutions.jts.geom.LineString;
import com.vividsolutions.jts.geom.LinearRing;
import com.vividsolutions.jts.geom.MultiLineString;
import com.vividsolutions.jts.geom.MultiPoint;
import com.vividsolutions.jts.geom.MultiPolygon;
import com.vividsolutions.jts.geom.Point;
import com.vividsolutions.jts.geom.Polygon;
import es.prodevelop.geodetic.utils.conversion.ConversionCoords;
import es.prodevelop.gvsig.mini.exceptions.BaseException;
import es.prodevelop.gvsig.mini.geom.api.IGeometry;
import es.prodevelop.gvsig.mini.geom.impl.base.Feature;
import es.prodevelop.gvsig.mobile.fmap.proj.CRSFactory;
import es.prodevelop.gvsig.mobile.fmap.proj.Projection;
public class JTSFeature extends Feature {
private ArrayList features = new ArrayList();
private JTSEnvelope envelope;
private JTSFeature parentFeature = null;
private IGeometry projectedGeometry = null;
public JTSFeature(IGeometry geometry) {
super(geometry);
envelope = new JTSEnvelope();
}
public void setEnvelope(JTSEnvelope envelope) {
this.envelope = envelope;
}
public void setEnvelope(double minX, double minY, double maxX, double maxY) {
this.envelope.init(minX, maxX, minY, maxY);
}
public JTSEnvelope getEnvelope() {
return this.envelope;
}
public ArrayList getFeatures() {
return features;
}
public JTSFeature getParentFeature() {
return parentFeature;
}
public void setParentFeature(JTSFeature parentFeature) {
this.parentFeature = parentFeature;
}
public IGeometry getProjectedGeometry() {
return projectedGeometry;
}
public void setSRS(String srs) {
if (srs == null) {
int srid = -1;
try {
srid = this.getGeometry().getGeometry().getSRID();
} catch (BaseException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
if (srid != -1) {
this.setSRS("EPSG:" + srid);
}
} else {
super.setSRS(srs);
}
}
/*
* (non-Javadoc)
*
* @see
* es.prodevelop.gvsig.mini.geom.impl.IFeature#setGeometry(es.prodevelop
* .gvsig.mini.geom.IGeometry)
*/
public void setGeometry(final IGeometry geometry) {
super.setGeometry(geometry);
}
public void reprojectGeometry(String SRS) throws BaseException {
Projection destination = CRSFactory.getCRS(SRS);
String originSRS = this.getSRS();
if (SRS.compareTo(originSRS) == 0) {
try {
this.projectedGeometry = new JTSGeometry((Geometry) this
.getGeometry().getGeometry().clone());
} catch (BaseException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return;
}
Geometry geom = this.getGeometry().getGeometry();
if (originSRS != null) {
Projection origin = CRSFactory.getCRS(originSRS);
if (destination != null && origin != null) {
if (geom instanceof Point) {
this.projectedGeometry = new JTSGeometry(
this.reprojectPointsOrLines(geom, origin,
destination, false));
} else if (geom instanceof LineString
|| geom instanceof LinearRing) {
this.projectedGeometry = new JTSGeometry(
this.reprojectPointsOrLines(geom, origin,
destination, false));
} else if (geom instanceof MultiPoint) {
this.projectedGeometry = new JTSGeometry(
this.reprojectPointsOrLines(geom, origin,
destination, false));
} else if (geom instanceof MultiLineString) {
this.projectedGeometry = new JTSGeometry(
this.reprojectMultiLines(geom, origin, destination));
} else if (geom instanceof Polygon) {
this.projectedGeometry = new JTSGeometry(
this.reprojectPolygon(geom, origin, destination));
} else if (geom instanceof MultiPolygon) {
this.projectedGeometry = new JTSGeometry(
this.reprojectMultiPolygon(geom, origin,
destination));
} else if (geom instanceof GeometryCollection) {
this.projectedGeometry = new JTSGeometry(
this.reprojectGeometryCollection(geom, origin,
destination));
}
}
}
}
public Geometry reprojectPointsOrLines(Geometry geom, Projection origin,
Projection destination, boolean forceLinearRing) {
GeometryFactory f = new GeometryFactory();
Coordinate[] coordinates = geom.getCoordinates();
final int size = coordinates.length;
Coordinate c;
double[] projC;
Coordinate newCoordinate;
Coordinate[] newCoordinates = new Coordinate[size];
for (int i = 0; i < size; i++) {
c = coordinates[i];
projC = ConversionCoords.reproject(c.x, c.y, origin, destination);
newCoordinate = new Coordinate(projC[0], projC[1]);
newCoordinates[i] = newCoordinate;
}
if (geom instanceof Point) {
return f.createPoint(newCoordinates[0]);
} else if (geom instanceof LineString && !forceLinearRing) {
return f.createLineString(newCoordinates);
} else if (geom instanceof LinearRing || forceLinearRing) {
return f.createLinearRing(newCoordinates);
} else if (geom instanceof MultiPoint) {
return f.createMultiPoint(newCoordinates);
}
return null;
}
public Geometry reprojectMultiLines(Geometry geom, Projection origin,
Projection destination) {
GeometryFactory f = new GeometryFactory();
MultiLineString lines = (MultiLineString) geom;
final int length = lines.getNumGeometries();
LineString[] projLines = new LineString[length];
LineString line;
for (int j = 0; j < length; j++) {
line = (LineString) lines.getGeometryN(j);
projLines[j] = (LineString) this.reprojectPointsOrLines(line,
origin, destination, false);
}
if (geom instanceof MultiLineString) {
return f.createMultiLineString(projLines);
}
return null;
}
public Geometry reprojectPolygon(Geometry geom, Projection origin,
Projection destination) {
GeometryFactory f = new GeometryFactory();
Polygon poly = (Polygon) geom;
LinearRing exteriorRing = (LinearRing) this.reprojectPointsOrLines(
poly.getExteriorRing(), origin, destination, true);
final int length = poly.getNumInteriorRing();
LinearRing[] projLines = new LinearRing[length];
LinearRing line;
for (int j = 0; j < length; j++) {
line = (LinearRing) poly.getInteriorRingN(j);
projLines[j] = (LinearRing) this.reprojectPointsOrLines(line,
origin, destination, true);
}
if (geom instanceof Polygon) {
return f.createPolygon(exteriorRing, projLines);
}
return null;
}
public Geometry reprojectMultiPolygon(Geometry geom, Projection origin,
Projection destination) {
GeometryFactory f = new GeometryFactory();
MultiPolygon multi = (MultiPolygon) geom;
final int length = multi.getNumGeometries();
Polygon[] polygons = new Polygon[length];
Polygon poly;
for (int j = 0; j < length; j++) {
poly = (Polygon) multi.getGeometryN(j);
polygons[j] = (Polygon) this.reprojectPointsOrLines(poly, origin,
destination, false);
}
if (geom instanceof MultiPolygon) {
return f.createMultiPolygon(polygons);
}
return null;
}
public Geometry reprojectGeometryCollection(Geometry geom,
Projection origin, Projection destination) {
GeometryFactory f = new GeometryFactory();
GeometryCollection multi = (GeometryCollection) geom;
final int length = multi.getNumGeometries();
Geometry[] geometries = new Geometry[length];
Geometry poly;
for (int j = 0; j < length; j++) {
poly = multi.getGeometryN(j);
if (poly instanceof Point) {
poly = this.reprojectPointsOrLines(poly, origin, destination,
false);
} else if (poly instanceof LineString || poly instanceof LinearRing) {
poly = this.reprojectPointsOrLines(poly, origin, destination,
false);
} else if (poly instanceof MultiPoint) {
poly = this.reprojectPointsOrLines(poly, origin, destination,
false);
} else if (poly instanceof MultiLineString) {
poly = this.reprojectMultiLines(poly, origin, destination);
} else if (poly instanceof Polygon) {
poly = this.reprojectPolygon(poly, origin, destination);
} else if (poly instanceof MultiPolygon) {
poly = this.reprojectMultiPolygon(poly, origin, destination);
} else if (poly instanceof GeometryCollection) {
poly = this.reprojectGeometryCollection(poly, origin,
destination);
}
geometries[j] = poly;
}
if (geom instanceof GeometryCollection) {
return f.createGeometryCollection(geometries);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy