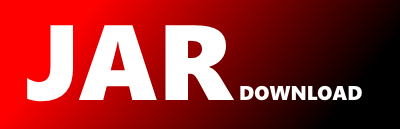
es.prodevelop.pui9.mail.AbstractPuiMailSender Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of es.prodevelop.pui9.services Show documentation
Show all versions of es.prodevelop.pui9.services Show documentation
Support for business layer (BO)
package es.prodevelop.pui9.mail;
import java.nio.charset.StandardCharsets;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.core.io.ByteArrayResource;
import org.springframework.core.io.InputStreamSource;
import org.springframework.mail.MailException;
import org.springframework.mail.javamail.JavaMailSenderImpl;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.util.CollectionUtils;
import org.springframework.util.StringUtils;
import es.prodevelop.pui9.services.exceptions.PuiServiceSendMailException;
import es.prodevelop.pui9.services.exceptions.PuiServiceWrongMailException;
/**
* Utility class to send emails. This class is a singleton, and it's configured
* using a file named "pui_email.properties" that should exist in the resources
* folder of the application
*
* @author Marc Gil - [email protected]
*/
public abstract class AbstractPuiMailSender {
protected final Logger logger = LogManager.getLogger(this.getClass());
protected JavaMailSenderImpl mailSender;
protected String from;
/**
* The properties set in the file should be the following:
*
* - pui.mail.from: the email used in the From tag
* - pui.mail.smtp.host: the SMTP server
* - pui.mail.smtp.port: the port of the SMTP server (by default
* 587)
* - pui.mail.smtp.user: the user to authenticate in the SMTP
* server
* - pui.mail.smtp.pass: the password of the SMTP server user
* - pui.mail.smtp.default.encoding: the encoding to be used in the
* email (by default UTF8
* - pui.mail.smtp.auth: if authentication is needed (by default
* true)
* - pui.mail.smtp.starttls.enable: if TLS o SSL connection is needed
* (by default true)
*
*/
protected AbstractPuiMailSender() {
mailSender = new JavaMailSenderImpl();
}
public abstract void configureSender();
/**
* Change the "from" email with the one provided
*
* @param from The new "from" email
*/
public void setFrom(String from) throws PuiServiceWrongMailException {
PuiMailConfiguration.validateEmail(from);
this.from = from;
}
/**
* Send an email providing the following information in the parameters:
*
* @param config The email configuration
* @throws Exception If any exception is thrown while sending the email
*/
public void send(PuiMailConfiguration config) throws PuiServiceSendMailException {
configureSender();
if (CollectionUtils.isEmpty(config.getTo()) && CollectionUtils.isEmpty(config.getCc())
&& CollectionUtils.isEmpty(config.getBcc())) {
throw new PuiServiceSendMailException("Missing receiver email address");
}
logger.debug("Sending email from '" + from + "' to '" + String.join(",", config.getTo()) + "' (bcc: '"
+ String.join(",", config.getBcc()) + "', cc: '" + String.join(",", config.getCc()) + "') [through '"
+ mailSender.getHost() + ":" + mailSender.getPort() + "', username: '" + mailSender.getUsername()
+ "']");
MimeMessage message = mailSender.createMimeMessage();
try {
MimeMessageHelper helper = new MimeMessageHelper(message, !config.getAttachments().isEmpty(),
StandardCharsets.UTF_8.name());
helper.setFrom(from);
helper.setTo(config.getTo().toArray(new String[0]));
helper.setCc(config.getCc().toArray(new String[0]));
helper.setBcc(config.getBcc().toArray(new String[0]));
helper.setSubject(config.getSubject());
helper.setText(config.getContent(), config.isHtml());
// attachments
for (PuiMailAttachment attachment : config.getAttachments()) {
InputStreamSource byteArrayResource = new ByteArrayResource(attachment.getContent());
if (StringUtils.hasText(attachment.getContentType())) {
helper.addAttachment(attachment.getFilename(), byteArrayResource, attachment.getContentType());
} else {
helper.addAttachment(attachment.getFilename(), byteArrayResource);
}
}
} catch (MessagingException e) {
throw new PuiServiceSendMailException(e.getMessage());
}
try {
this.mailSender.send(message);
} catch (MailException e) {
throw new PuiServiceSendMailException(e.getMessage());
}
logger.debug("The email was sent correctly");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy