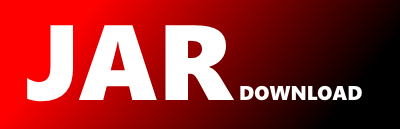
es.prodevelop.pui9.mail.PuiMailConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of es.prodevelop.pui9.services Show documentation
Show all versions of es.prodevelop.pui9.services Show documentation
Support for business layer (BO)
package es.prodevelop.pui9.mail;
import java.io.File;
import java.io.InputStreamReader;
import java.io.StringWriter;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.regex.Pattern;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.IOUtils;
import org.apache.velocity.VelocityContext;
import org.apache.velocity.app.VelocityEngine;
import org.springframework.util.StringUtils;
import es.prodevelop.pui9.classpath.PuiClassLoaderUtils;
import es.prodevelop.pui9.services.exceptions.PuiServiceWrongMailException;
public class PuiMailConfiguration {
private static final Pattern EMAIL_PATTERN = Pattern.compile("^[A-Z0-9._%+-]+@[A-Z0-9.-]+\\.[A-Z]{2,6}$",
Pattern.CASE_INSENSITIVE);
private static VelocityEngine engine;
static {
engine = new VelocityEngine();
engine.addProperty("runtime.log", FileUtils.getTempDirectory().toString() + File.separator + "velocity.log");
engine.init();
}
private List to;
private List cc;
private List bcc;
private String subject;
private String content;
private boolean isHtml;
private List attachments;
public PuiMailConfiguration() {
to = new ArrayList<>();
cc = new ArrayList<>();
bcc = new ArrayList<>();
attachments = new ArrayList<>();
}
public List getTo() {
return to;
}
public void addTo(String to) throws PuiServiceWrongMailException {
addTo(Collections.singletonList(to));
}
public void addTo(List to) throws PuiServiceWrongMailException {
to = validateEmails(to);
for (String email : to) {
this.to.add(email);
}
}
public List getCc() {
return cc;
}
public void addCc(String cc) throws PuiServiceWrongMailException {
addCc(Collections.singletonList(cc));
}
public void addCc(List cc) throws PuiServiceWrongMailException {
cc = validateEmails(cc);
for (String email : cc) {
this.cc.add(email);
}
}
public List getBcc() {
return bcc;
}
public void addBcc(String bcc) throws PuiServiceWrongMailException {
addBcc(Collections.singletonList(bcc));
}
public void addBcc(List bcc) throws PuiServiceWrongMailException {
bcc = validateEmails(bcc);
for (String email : bcc) {
this.bcc.add(email);
}
}
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public boolean isHtml() {
return isHtml;
}
public void setHtml(boolean isHtml) {
this.isHtml = isHtml;
}
public List getAttachments() {
return attachments;
}
public void addAttachment(PuiMailAttachment attachment) {
addAttachment(Collections.singletonList(attachment));
}
public void addAttachment(List attachments) {
this.attachments.addAll(attachments);
}
private List validateEmails(List emails) throws PuiServiceWrongMailException {
List newEmails = new ArrayList<>();
for (String email : emails) {
validateEmail(email);
newEmails.add(email);
}
return newEmails;
}
/**
* Compile the given template (as path) with the given parameters using Velocity
*
* @param templatePath The path of the template
* @param parameters The map with the parameters for velocity
* @return The content compiled
*/
public static String compileTemplateFromClasspath(String templatePath, Map parameters) {
try {
String template = IOUtils.toString(PuiClassLoaderUtils.getClassLoader().getResourceAsStream(templatePath),
StandardCharsets.UTF_8);
return compileTemplate(template, parameters);
} catch (Exception e) {
return null;
}
}
/**
* Compile the given template with the given parameters using Velocity
*
* @param templateThe path of the template
* @param parameters The map with the parameters for velocity
* @return The content compiled
*/
public static String compileTemplate(String template, Map parameters) {
try {
VelocityContext context = new VelocityContext();
for (Entry entry : parameters.entrySet()) {
context.put(entry.getKey(), entry.getValue());
}
InputStreamReader isr = new InputStreamReader(IOUtils.toInputStream(template, StandardCharsets.UTF_8));
StringWriter writer = new StringWriter();
boolean processed = engine.evaluate(context, writer, "PUI_MAIL", isr);
return processed ? writer.toString() : null;
} catch (Exception e) {
return null;
}
}
public static void validateEmail(String email) throws PuiServiceWrongMailException {
if (!StringUtils.hasText(email)) {
throw new PuiServiceWrongMailException(email);
}
email = email.trim();
boolean isValid = EMAIL_PATTERN.matcher(email).matches();
if (!isValid) {
throw new PuiServiceWrongMailException(email);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy