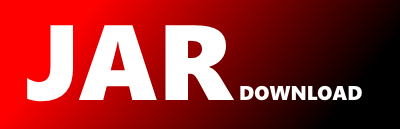
es.ree.eemws.kit.cmd.put.Main Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eemws-kit Show documentation
Show all versions of eemws-kit Show documentation
Client implementation of IEC 62325-504 technical specification. eemws-kit includes command line utilities to invoke the eem web services, as well as several GUI applications (browser, editor, ...)
The newest version!
/*
* Copyright 2024 Redeia.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, version 3 of the license.
*
* This program is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTIBIILTY or FITNESS FOR A PARTICULAR PURPOSE. See GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program. If not, see
* http://www.gnu.org/licenses/.
*
* Any redistribution and/or modification of this program has to make
* reference to Redeia as the copyright owner of the program.
*/
package es.ree.eemws.kit.cmd.put;
import java.io.File;
import java.io.IOException;
import java.net.MalformedURLException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import es.ree.eemws.client.put.PutMessage;
import es.ree.eemws.core.utils.config.ConfigException;
import es.ree.eemws.core.utils.error.EnumErrorCatalog;
import es.ree.eemws.core.utils.file.FileUtil;
import es.ree.eemws.core.utils.iec61968100.EnumMessageFormat;
import es.ree.eemws.core.utils.operations.put.PutOperationException;
import es.ree.eemws.kit.cmd.MessageCatalog;
import es.ree.eemws.kit.cmd.ParentMain;
/**
* Puts a message using the command line.
*
* @author Redeia.
* @version 2.1 01/01/2024
*/
public final class Main extends ParentMain {
/** Log messages. */
private static final Logger LOGGER = Logger.getLogger("put"); //$NON-NLS-1$
/** Sets text for parameter in
. */
private static final String PUT_PARAMETER_IN = PutMessageCatalog.PUT_PARAMETER_IN.getMessage();
/** Sets text for parameter attachment
. */
private static final String PUT_PARAMETER_ATTACHMENT = PutMessageCatalog.PUT_PARAMETER_ATTACHMENT.getMessage();
/** Sets text for parameter url
. */
private static final String PARAMETER_URL = MessageCatalog.PARAMETER_URL.getMessage();
/** Sets text for parameter out
. */
private static final String PARAMETER_OUT_FILE = MessageCatalog.PARAMETER_OUT_FILE.getMessage();
/**
* Main. Executes the put action.
*
* @param args command line arguments.
*/
public static void main(final String[] args) {
String urlEndPoint = null;
String fileIn = null;
String attachment = null;
String outputFile = null;
PutMessage put = null;
long init = -1;
try {
/* Reads command line parameters, store its values. */
List arguments = new ArrayList<>(Arrays.asList(args));
/* If the list has duplicates must stop the execution. */
var dup = findDuplicates(arguments, PUT_PARAMETER_IN, PUT_PARAMETER_ATTACHMENT, PARAMETER_OUT_FILE,
PARAMETER_URL);
if (dup != null) {
throw new PutOperationException(EnumErrorCatalog.PUT_017,
MessageCatalog.PARAMETER_REPEATED.getMessage(dup));
}
fileIn = readParameter(arguments, PUT_PARAMETER_IN);
attachment = readParameter(arguments, PUT_PARAMETER_ATTACHMENT);
outputFile = readParameter(arguments, PARAMETER_OUT_FILE);
urlEndPoint = readParameter(arguments, PARAMETER_URL);
/*
* If the list is not empty means that user has put at least one "unknown"
* parameter. Show only first.
*/
if (!arguments.isEmpty()) {
throw new PutOperationException(EnumErrorCatalog.PUT_017, arguments.get(0));
}
/* Basic parameter validation. */
if (fileIn == null && attachment == null) {
throw new PutOperationException(EnumErrorCatalog.PUT_017,
PutMessageCatalog.PUT_PARAMETER_NO_INPUT_FILE.getMessage());
}
if (fileIn != null && attachment != null) {
throw new PutOperationException(EnumErrorCatalog.PUT_017,
PutMessageCatalog.PUT_PARAMETER_NO_TWO_KINDS_INPUT_FILE.getMessage(PUT_PARAMETER_IN,
PUT_PARAMETER_ATTACHMENT));
}
/* Sets the url, if no url is provided by arguments, use the one configured. */
urlEndPoint = setConfig(urlEndPoint);
/* Creates and set up a put object. */
put = new PutMessage();
put.setEndPoint(urlEndPoint);
init = System.currentTimeMillis();
String response;
/* Sends the message. */
if (fileIn == null) {
var f = new File(attachment);
var fileName = f.getName();
response = put.put(fileName, FileUtil.readBinary(attachment), EnumMessageFormat.BINARY);
} else {
response = put.put(new StringBuilder(FileUtil.readUTF8(fileIn)));
}
/* Prints the response on screen if the user didn't specified an output file. */
if (response == null) {
LOGGER.fine("Response was empty"); //$NON-NLS-1$
} else {
writeResponse(outputFile, init, response);
}
} catch (PutOperationException e) {
var code = e.getCode();
if (code.equals(EnumErrorCatalog.HAND_010.name())) {
if (LOGGER.isLoggable(Level.SEVERE)) {
LOGGER.log(Level.SEVERE,
String.format("%s: %s %s", e.getCode(), e.getMessage(), e.getCause().getMessage())); //$NON-NLS-1$
}
/*
* Server returns fault and user wants a response file? Write the fault as
* server response.
*/
if (put != null && outputFile != null) {
var faultStr = put.getMessageMetaData().getRejectText();
writeResponse(outputFile, init, faultStr);
}
} else {
if (LOGGER.isLoggable(Level.SEVERE)) {
LOGGER.log(Level.SEVERE, String.format("%s: %s", e.getCode(), e.getMessage()), e.getCause()); //$NON-NLS-1$
}
/* Bad parameters? show usage! */
if (code.equals(EnumErrorCatalog.PUT_017.name())) {
LOGGER.info(PutMessageCatalog.PUT_USAGE.getMessage(PUT_PARAMETER_IN, PUT_PARAMETER_ATTACHMENT,
PARAMETER_OUT_FILE, PARAMETER_URL, new Date()));
}
}
/* Show full stack trace in debug. */
LOGGER.log(Level.FINE, "", e); //$NON-NLS-1$
} catch (MalformedURLException e) {
LOGGER.severe(MessageCatalog.INVALID_URL.getMessage(urlEndPoint));
} catch (ConfigException e) {
LOGGER.severe(MessageCatalog.INVALID_CONFIGURATION.getMessage(e.getMessage()));
/* Shows stack trace only for debug. Don't bother the user with this details. */
LOGGER.log(Level.FINE, MessageCatalog.INVALID_CONFIGURATION.getMessage(e.getMessage()), e);
} catch (IOException e) {
if (fileIn == null) {
LOGGER.severe(MessageCatalog.UNABLE_TO_READ.getMessage(attachment));
} else {
LOGGER.severe(MessageCatalog.UNABLE_TO_READ.getMessage(fileIn));
}
}
}
/**
* Writes server's response.
*
* @param outputFile Out abosolute file path.
* @param init Request start time (to print performance)
* @param response Server response.
*/
private static void writeResponse(final String outputFile, final long init, final String response) {
try {
if (outputFile == null) {
LOGGER.info(response);
} else {
FileUtil.writeUTF8(outputFile, response);
if (LOGGER.isLoggable(Level.INFO)) {
LOGGER.info(
MessageCatalog.EXECUTION_TIME.getMessage(getPerformance(init, System.currentTimeMillis())));
}
}
} catch (IOException ioe) {
LOGGER.severe(MessageCatalog.UNABLE_TO_WRITE.getMessage(outputFile));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy