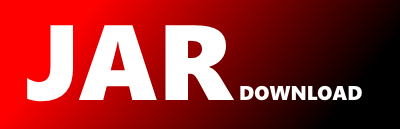
es.ree.eemws.kit.cmd.query.Main Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eemws-kit Show documentation
Show all versions of eemws-kit Show documentation
Client implementation of IEC 62325-504 technical specification. eemws-kit includes command line utilities to invoke the eem web services, as well as several GUI applications (browser, editor, ...)
The newest version!
/*
* Copyright 2024 Redeia.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, version 3 of the license.
*
* This program is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTIBIILTY or FITNESS FOR A PARTICULAR PURPOSE. See GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program. If not, see
* http://www.gnu.org/licenses/.
*
* Any redistribution and/or modification of this program has to make
* reference to Redeia as the copyright owner of the program.
*/
package es.ree.eemws.kit.cmd.query;
import java.io.IOException;
import java.net.MalformedURLException;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import es.ree.eemws.client.querydata.QueryData;
import es.ree.eemws.core.utils.config.ConfigException;
import es.ree.eemws.core.utils.error.EnumErrorCatalog;
import es.ree.eemws.core.utils.file.FileUtil;
import es.ree.eemws.core.utils.iec61968100.EnumFilterElement;
import es.ree.eemws.core.utils.iec61968100.MessageUtil;
import es.ree.eemws.core.utils.operations.query.QueryOperationException;
import es.ree.eemws.kit.cmd.MessageCatalog;
import es.ree.eemws.kit.cmd.ParentMain;
/**
* Sends a query request.
*
* @author Redeia.
* @version 2.1 01/01/2024
*/
public final class Main extends ParentMain {
/** Log messages. */
private static final Logger LOGGER = Logger.getLogger("query"); //$NON-NLS-1$
/** Sets text for parameter startTime
. */
private static final String PARAMETER_START_TIME = MessageCatalog.PARAMETER_START_TIME.getMessage();
/** Sets text for parameter endTime
. */
private static final String PARAMETER_END_TIME = MessageCatalog.PARAMETER_END_TIME.getMessage();
/** Sets text for parameter url
. */
private static final String PARAMETER_URL = MessageCatalog.PARAMETER_URL.getMessage();
/** Sets text for parameter out
. */
private static final String PARAMETER_OUT_FILE = MessageCatalog.PARAMETER_OUT_FILE.getMessage();
/** Sets text for parameter id
. */
private static final String QUERY_PARAMETER_ID = QueryMessageCatalog.QUERY_PARAMETER_ID.getMessage();
/** Token that specifies that the value is a parameter name, not a value. */
private static final String PARAMETER_PREFIX = "-"; //$NON-NLS-1$
/**
* Main. Execute the query action.
*
* @param args command line arguments.
*/
public static void main(final String[] args) {
QueryData query = null;
String outputFile = null;
String urlEndPoint = null;
long init = -1;
try {
/* Reads command line parameters, store its values. */
List arguments = new ArrayList<>(Arrays.asList(args));
/* If the list has duplicates must stop the execution. */
var dup = findDuplicates(arguments, PARAMETER_START_TIME, PARAMETER_END_TIME, QUERY_PARAMETER_ID,
PARAMETER_URL, PARAMETER_OUT_FILE);
if (dup != null) {
throw new QueryOperationException(EnumErrorCatalog.QRY_010,
MessageCatalog.PARAMETER_REPEATED.getMessage(dup));
}
var startTime = readParameter(arguments, PARAMETER_START_TIME);
var endTime = readParameter(arguments, PARAMETER_END_TIME);
var dataType = readParameter(arguments, QUERY_PARAMETER_ID);
urlEndPoint = readParameter(arguments, PARAMETER_URL);
outputFile = readParameter(arguments, PARAMETER_OUT_FILE);
/* Creates a request with all the parameters. */
var msgOptions = new HashMap();
if (dataType != null) {
msgOptions.put(EnumFilterElement.DATA_TYPE.toString(), dataType);
}
var sdf = new SimpleDateFormat(DATE_FORMAT_PATTERN);
if (startTime != null) {
var dateStartTime = validateStartTime(startTime, sdf);
msgOptions.put(EnumFilterElement.START_TIME.toString(), MessageUtil.formatDate(dateStartTime));
}
if (endTime != null) {
try {
var dateEndTime = sdf.parse(endTime);
msgOptions.put(EnumFilterElement.END_TIME.toString(), MessageUtil.formatDate(dateEndTime));
} catch (ParseException e) {
throw new QueryOperationException(EnumErrorCatalog.QRY_009, PARAMETER_END_TIME,
DATE_FORMAT_PATTERN);
}
}
/* Retrieves dinamic parameters from the command line. */
var len = arguments.size();
for (var cont = 0; cont < len; cont++) {
var key = arguments.get(cont);
if (!key.startsWith(PARAMETER_PREFIX)) {
throw new QueryOperationException(EnumErrorCatalog.QRY_010,
QueryMessageCatalog.QUERY_INCORRECT_PARAMETER_LIST.getMessage(key));
}
key = key.substring(1); // remove prefix, keep just the name
if (key.length() == 0) {
throw new QueryOperationException(EnumErrorCatalog.QRY_010,
QueryMessageCatalog.QUERY_INCORRECT_PARAMETER_ID.getMessage());
}
if ((cont + 1) < len) {
var value = arguments.get(cont + 1);
if (value.startsWith(PARAMETER_PREFIX)) { // Next value is other parameter not a value
msgOptions.put(key, null);
} else {
msgOptions.put(key, value);
cont++;
}
} else { // last parameter, with no value
msgOptions.put(key, null);
}
}
/* Sets the url, if no url is provided by arguments, use the one configured. */
urlEndPoint = setConfig(urlEndPoint);
/* Creates and set up a query object. */
query = new QueryData();
query.setEndPoint(urlEndPoint);
init = System.currentTimeMillis();
var response = query.query(msgOptions);
writeResponse(outputFile, init, response);
} catch (QueryOperationException e) {
var code = e.getCode();
if (code.equals(EnumErrorCatalog.HAND_010.name())) {
LOGGER.log(Level.SEVERE,
String.format("%s: %s %s", e.getCode(), e.getMessage(), e.getCause().getMessage()));
/*
* Server returns fault and user wants a response file? Write the fault as
* server response.
*/
if (query != null && outputFile != null) {
var faultStr = query.getMessageMetaData().getRejectText();
writeResponse(outputFile, init, faultStr);
}
} else {
if (LOGGER.isLoggable(Level.SEVERE)) {
LOGGER.log(Level.SEVERE, String.format("%s: %s", e.getCode(), e.getMessage()), e.getCause()); //$NON-NLS-1$
}
/* Bad parameters? show usage! */
if (code.equals(EnumErrorCatalog.QRY_009.name()) || code.equals(EnumErrorCatalog.QRY_001.name())
|| code.equals(EnumErrorCatalog.QRY_010.name())) {
LOGGER.info(QueryMessageCatalog.QUERY_USAGE.getMessage(QUERY_PARAMETER_ID, PARAMETER_START_TIME,
PARAMETER_END_TIME, PARAMETER_OUT_FILE, PARAMETER_URL, new Date()));
}
}
/* Show full stack trace in debug. */
LOGGER.log(Level.FINE, "", e); //$NON-NLS-1$
} catch (MalformedURLException e) {
LOGGER.severe(MessageCatalog.INVALID_URL.getMessage(urlEndPoint));
} catch (ConfigException e) {
LOGGER.severe(MessageCatalog.INVALID_CONFIGURATION.getMessage(e.getMessage()));
/* Shows stack trace only for debug. Don't bother the user with this details. */
LOGGER.log(Level.FINE, MessageCatalog.INVALID_CONFIGURATION.getMessage(e.getMessage()), e);
}
}
private static Date validateStartTime(String startTime, SimpleDateFormat sdf) throws QueryOperationException {
Date date = null;
try {
date = sdf.parse(startTime);
} catch (ParseException e) {
throw new QueryOperationException(EnumErrorCatalog.QRY_009, PARAMETER_START_TIME, DATE_FORMAT_PATTERN);
}
return date;
}
/**
* Writes server's response.
*
* @param outputFile Out abosolute file path.
* @param init Request start time (to print performance)
* @param response Server response.
*/
private static void writeResponse(final String outputFile, final long init, final String response) {
try {
if (outputFile == null) {
LOGGER.info(response);
} else {
FileUtil.writeUTF8(outputFile, response);
if (LOGGER.isLoggable(Level.INFO)) {
LOGGER.info(
MessageCatalog.EXECUTION_TIME.getMessage(getPerformance(init, System.currentTimeMillis())));
}
}
} catch (IOException ioe) {
LOGGER.severe(MessageCatalog.UNABLE_TO_WRITE.getMessage(outputFile));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy