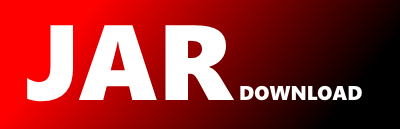
es.ree.eemws.kit.folders.FolderManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eemws-kit Show documentation
Show all versions of eemws-kit Show documentation
Client implementation of IEC 62325-504 technical specification. eemws-kit includes command line utilities to invoke the eem web services, as well as several GUI applications (browser, editor, ...)
The newest version!
/*
* Copyright 2024 Redeia.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, version 3 of the license.
*
* This program is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTIBIILTY or FITNESS FOR A PARTICULAR PURPOSE. See GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program. If not, see
* http://www.gnu.org/licenses/.
*
* Any redistribution and/or modification of this program has to make
* reference to Redeia as the copyright owner of the program.
*/
package es.ree.eemws.kit.folders;
import java.rmi.RemoteException;
import java.util.List;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
import es.ree.eemws.core.utils.config.ConfigException;
/**
* Main class. Creates all the objects and schedules.
*
* @author Redeia.
* @version 2.1 01/01/2024
*
*/
public final class FolderManager {
/** Logging. */
private static final Logger LOGGER = Logger.getLogger(FolderManager.class.getName());
/** Override sleep value if list is made by date instead of code. */
private static final long SLEEP_BY_DATE = 30 * 1000L;
/**
* Private constructor. Utility classes should not have a public constructor.
*/
private FolderManager() {
/* Utility classes should not have a public constructor. */
}
/**
* Main method, creates the application objects and schedules.
*
* @param args Command line parameters (ignored)
*/
public static void main(final String[] args) {
try {
var config = new MagicFolderConfiguration();
config.readConfiguration();
if (ExecutionControl.isRunning(config.getInstanceID())) {
if (StatusIcon.getStatus().isInteractive()) {
JOptionPane.showMessageDialog(null, MessageCatalog.MF_ALREADY_RUNNING.getMessage(),
MessageCatalog.MF_TITLE_ERROR.getMessage(), JOptionPane.ERROR_MESSAGE);
}
if (LOGGER.isLoggable(Level.INFO)) {
LOGGER.info(MessageCatalog.MF_ALREADY_RUNNING.getMessage());
}
StatusIcon.getStatus().removeIcon();
} else {
ScheduledExecutorService scheduler = null;
/* Status images Initialization. */
StatusIcon.getStatus().setIdle();
/* Creates lock handler. */
var lh = new LockHandler(config);
scheduler = Executors.newScheduledThreadPool(config.getMaxNumThreads());
var ics = config.getInputConfigurationSet();
for (InputConfigurationSet ic : ics) {
scheduler.scheduleAtFixedRate(new InputTask(lh, ic), 0, ic.getSleepTime(), TimeUnit.MILLISECONDS);
}
for (List lst : config.getOutputConfigurationSet()) {
var sleep = Long.MAX_VALUE;
var outSetIds = new StringBuilder();
for (OutputConfigurationSet ocs : lst) {
var curr = ocs.getSleepTime();
if (sleep > curr) {
sleep = curr;
}
outSetIds.append(ocs.getIndex());
outSetIds.append("-"); //$NON-NLS-1$
}
outSetIds.setLength(outSetIds.length() - 1);
var setIds = outSetIds.toString();
if (setIds.equals("0")) { //$NON-NLS-1$
setIds = ""; //$NON-NLS-1$
} else {
setIds = "-" + setIds; //$NON-NLS-1$
}
if (System.getProperty(MagicFolderConfiguration.LIST_BY_DATE_KEY) == null) {
if (LOGGER.isLoggable(Level.INFO)) {
LOGGER.info(MessageCatalog.MF_CONFIG_DELAY_TIME_O.getMessage(setIds, sleep));
}
} else {
sleep = SLEEP_BY_DATE;
if (LOGGER.isLoggable(Level.INFO)) {
LOGGER.info(MessageCatalog.MF_CONFIG_DELAY_TIME_O_DATE.getMessage(setIds, sleep));
}
}
scheduler.scheduleAtFixedRate(new OutputTask(lh, lst, setIds), 0, sleep, TimeUnit.MILLISECONDS);
}
/* Create deletion / backup folder. */
if (config.getBackupFolder() != null) {
var dft = new DeleteFilesTask(lh, config);
int numDays = config.getNumOfDaysKept();
scheduler.scheduleAtFixedRate(dft, numDays, numDays, TimeUnit.DAYS);
}
if (LOGGER.isLoggable(Level.INFO)) {
LOGGER.info(MessageCatalog.MF_RUNNING.getMessage());
}
}
} catch (ConfigException ex) {
if (StatusIcon.getStatus().isInteractive()) {
JOptionPane.showMessageDialog(null,
es.ree.eemws.kit.cmd.MessageCatalog.INVALID_CONFIGURATION.getMessage(ex.getMessage()),
MessageCatalog.MF_TITLE_ERROR.getMessage(), JOptionPane.ERROR_MESSAGE);
}
LOGGER.log(Level.SEVERE, es.ree.eemws.kit.cmd.MessageCatalog.INVALID_CONFIGURATION.getMessage(),
ex.getMessage());
// Force exit
StatusIcon.getStatus().removeIcon();
System.exit(0); // NOSONAR Invalid configuration: we need to force application to exit.
} catch (RemoteException ex) {
if (StatusIcon.getStatus().isInteractive()) {
JOptionPane.showMessageDialog(null,
es.ree.eemws.kit.cmd.MessageCatalog.INVALID_CONFIGURATION.getMessage(ex.getMessage()),
MessageCatalog.MF_TITLE_ERROR.getMessage(), JOptionPane.ERROR_MESSAGE);
}
LOGGER.log(Level.SEVERE, MessageCatalog.MF_CANNOT_REACH_REFERENCES.getMessage(), ex.getMessage());
StatusIcon.getStatus().removeIcon();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy