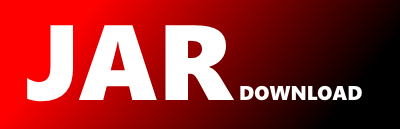
es.ree.eemws.kit.gui.applications.browser.FileHandle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eemws-kit Show documentation
Show all versions of eemws-kit Show documentation
Client implementation of IEC 62325-504 technical specification. eemws-kit includes command line utilities to invoke the eem web services, as well as several GUI applications (browser, editor, ...)
The newest version!
/*
* Copyright 2024 Redeia.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, version 3 of the license.
*
* This program is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTIBIILTY or FITNESS FOR A PARTICULAR PURPOSE. See GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program. If not, see
* http://www.gnu.org/licenses/.
*
* Any redistribution and/or modification of this program has to make
* reference to Redeia as the copyright owner of the program.
*/
package es.ree.eemws.kit.gui.applications.browser;
import java.io.File;
import java.io.IOException;
import java.io.Serializable;
import javax.swing.JCheckBoxMenuItem;
import javax.swing.JFileChooser;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import es.ree.eemws.client.get.RetrievedMessage;
import es.ree.eemws.core.utils.file.FileUtil;
import es.ree.eemws.kit.gui.common.Logger;
/**
* File management.
*
* @author Redeia.
* @version 2.1 01/01/2024
*/
public final class FileHandle implements Serializable {
/** Serial version UID. */
private static final long serialVersionUID = 1198206784017773924L;
/** Xml file extension. */
private static final String XML_FILE_EXTENSION = ".xml"; //$NON-NLS-1$
/** Reference to main window. */
private Browser mainWindow;
/** Logging object. */
private Logger logger;
/** Preset saving folder, prevents asking user every time. */
private String storeFolder = ""; //$NON-NLS-1$
/** Save As... dialogue */
private JFileChooser fcFileChooser = new JFileChooser();
/** Set active to create backup files are automatically. */
private JCheckBoxMenuItem cbmiBackupAuto = new JCheckBoxMenuItem();
/** Set active to save without asking. */
private JCheckBoxMenuItem cbmiStoreAuto = new JCheckBoxMenuItem();
/**
* Creates a new instance of File settings manager.
*
* @param window Reference to the main window.
*/
public FileHandle(final Browser window) {
mainWindow = window;
logger = mainWindow.getLogHandle().getLog();
}
/**
* Gets File menu.
*
* @return 'File' menu option.
*/
public JMenu getMenu() {
/* Create backup option. */
cbmiBackupAuto.setText(MessageCatalog.BROWSER_FILE_BACKUP_MENU_ENTRY.getMessage());
cbmiBackupAuto.setMnemonic(MessageCatalog.BROWSER_FILE_BACKUP_MENU_ENTRY_HK.getChar());
cbmiBackupAuto.setSelected(false);
/* 'save automatically' option. */
cbmiStoreAuto = new JCheckBoxMenuItem(MessageCatalog.BROWSER_FILE_SET_FOLDER_MENU_ENTRY.getMessage());
cbmiStoreAuto.setMnemonic(MessageCatalog.BROWSER_FILE_SET_FOLDER_MENU_ENTRY_HK.getChar());
cbmiStoreAuto.setSelected(false);
cbmiStoreAuto.addActionListener(e -> selectFolder());
/* 'exit' option. */
var miExit = new JMenuItem();
miExit.setText(MessageCatalog.BROWSER_FILE_EXIT_MENU_ENTRY.getMessage());
miExit.setMnemonic(MessageCatalog.BROWSER_FILE_EXIT_MENU_ENTRY_HK.getChar());
miExit.addActionListener(e -> exitApplication());
/* 'file' menu. */
var mnFileMenu = new JMenu();
mnFileMenu.setText(MessageCatalog.BROWSER_FILE_MENU_ENTRY.getMessage());
mnFileMenu.setMnemonic(MessageCatalog.BROWSER_FILE_MENU_ENTRY_HK.getChar());
mnFileMenu.add(cbmiBackupAuto);
mnFileMenu.add(cbmiStoreAuto);
mnFileMenu.addSeparator();
mnFileMenu.add(miExit);
return mnFileMenu;
}
/**
* Opens a dialog window to set a folder to save retrieved messages, thus user
* will not be asked every time a message is retrieved.
*/
private void selectFolder() {
if (cbmiStoreAuto.isSelected()) {
fcFileChooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
var returnVal = fcFileChooser.showSaveDialog(mainWindow);
if (returnVal == JFileChooser.APPROVE_OPTION) {
var file = fcFileChooser.getSelectedFile();
storeFolder = file.getAbsolutePath();
} else {
cbmiStoreAuto.setSelected(false);
}
}
}
/**
* Saves retrieved message using the ID passed as parameter.
*
* @param idMensaje ID for the message to save.
* @param response Request object containing requested message data.
* @throws IOException If cannot write file.
*/
public void saveFile(final String idMensaje, final RetrievedMessage response) throws IOException {
String fileName;
if (response.isBinary()) {
fileName = response.getFileName();
if (fileName == null) {
fileName = idMensaje;
}
} else {
fileName = idMensaje + XML_FILE_EXTENSION;
}
int returnVal;
if (cbmiStoreAuto.isSelected()) {
returnVal = JFileChooser.APPROVE_OPTION;
} else {
fcFileChooser = new JFileChooser();
fcFileChooser.setSelectedFile(new File(fileName));
returnVal = fcFileChooser.showSaveDialog(mainWindow);
}
if (returnVal == JFileChooser.APPROVE_OPTION) {
File file;
if (cbmiStoreAuto.isSelected()) {
file = new File(storeFolder + File.separator + fileName);
} else {
file = fcFileChooser.getSelectedFile();
}
var save = true;
if (file.exists()) {
if (cbmiBackupAuto.isSelected()) {
var nomBackup = FileUtil.createBackup(file.getAbsolutePath());
logger.logMessage(MessageCatalog.BROWSER_FILE_BACKUP_CREATED.getMessage(nomBackup));
} else {
var answer = JOptionPane.showConfirmDialog(mainWindow,
MessageCatalog.BROWSER_FILE_REPLACE_FILE.getMessage(file.getName()),
MessageCatalog.BROWSER_FILE_REPLACE_FILE_TITLE.getMessage(), JOptionPane.YES_NO_OPTION,
JOptionPane.WARNING_MESSAGE);
if (answer != JOptionPane.OK_OPTION) {
logger.logMessage(MessageCatalog.BROWSER_FILE_NO_REPLACE.getMessage(file.getName()));
save = false;
}
}
}
if (save) {
if (response.isBinary()) {
FileUtil.write(file.getAbsolutePath(), response.getBinaryPayload());
} else {
FileUtil.writeUTF8(file.getAbsolutePath(), response.getStringPayload());
}
logger.logMessage(MessageCatalog.BROWSER_FILE_FILE_SAVED.getMessage(file.getName()));
}
}
}
/**
* Asks for user confirmation before exiting application.
*/
public void exitApplication() {
var answer = JOptionPane.showConfirmDialog(mainWindow,
MessageCatalog.BROWSER_FILE_EXIT_APPLICATION.getMessage(),
MessageCatalog.BROWSER_FILE_EXIT_APPLICATION_TITLE.getMessage(), JOptionPane.OK_CANCEL_OPTION,
JOptionPane.QUESTION_MESSAGE);
if (answer == JOptionPane.OK_OPTION) {
System.exit(0); // NOSONAR We want to force application to exit.
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy