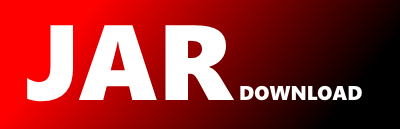
es.ree.eemws.kit.gui.applications.browser.GetMessageSender Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eemws-kit Show documentation
Show all versions of eemws-kit Show documentation
Client implementation of IEC 62325-504 technical specification. eemws-kit includes command line utilities to invoke the eem web services, as well as several GUI applications (browser, editor, ...)
The newest version!
/*
* Copyright 2024 Redeia.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, version 3 of the license.
*
* This program is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTIBIILTY or FITNESS FOR A PARTICULAR PURPOSE. See GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program. If not, see
* http://www.gnu.org/licenses/.
*
* Any redistribution and/or modification of this program has to make
* reference to Redeia as the copyright owner of the program.
*/
package es.ree.eemws.kit.gui.applications.browser;
import java.io.IOException;
import java.io.Serializable;
import java.math.BigInteger;
import java.net.URL;
import javax.swing.JOptionPane;
import es.ree.eemws.client.get.GetMessage;
import es.ree.eemws.core.utils.operations.get.GetOperationException;
import es.ree.eemws.kit.gui.common.Logger;
/**
* Sends 'Get' message to server.
*
* @author Redeia.
* @version 2.1 01/01/2024
*/
public final class GetMessageSender implements Serializable {
/** Serial version UID. */
private static final long serialVersionUID = 4453340866823196148L;
/** Message request object. */
private transient GetMessage get;
/** Reference to main window. */
private Browser mainWindow;
/** Reference to status bar. */
private StatusBar status;
/** Log object. */
private Logger logger;
/**
* Constructor. Creates new instance of request messages handler.
*
* @param url URL to which requests are sent.
* @param principal Reference to main window.
*/
public GetMessageSender(final URL url, final Browser principal) {
get = new GetMessage();
mainWindow = principal;
status = principal.getStatusBar();
logger = principal.getLogHandle().getLog();
setEndPoint(url);
}
/**
* Sets URL of the system to which requests are made.
*
* @param url URL of the system to which requests are made.
*/
public void setEndPoint(final URL url) {
get.setEndPoint(url);
}
/**
* Retrieves currently selected message/s.
*/
public void retrieve() {
var dataTable = mainWindow.getDataTable();
var selectedRows = dataTable.getSelectedRows();
var len = selectedRows.length;
if (len == 0) {
String msg;
if (dataTable.getModel().getRowCount() == 0) {
msg = MessageCatalog.BROWSER_NO_MESSAGES_TO_GET.getMessage();
} else {
msg = MessageCatalog.BROWSER_SELECT_MESSAGES_TO_GET.getMessage();
}
logger.logMessage(msg);
status.setStatus(msg);
JOptionPane.showMessageDialog(mainWindow, msg,
es.ree.eemws.kit.gui.common.MessageCatalog.MSG_INFO_TITLE.getMessage(),
JOptionPane.INFORMATION_MESSAGE);
} else if (len > 1) {
var msg = MessageCatalog.BROWSER_RETRIEVING_SEVERAL_MESSAGES.getMessage(len);
logger.logMessage(msg);
status.setStatus(msg);
mainWindow.enableScreen(false);
for (var cont = 0; cont < len; cont++) {
retieveWhenDisabled(dataTable.getSelectedRows()[cont]);
}
mainWindow.enableScreen(true);
} else {
mainWindow.enableScreen(false);
var row = dataTable.getSelectedRow();
Long codigo = ((BigInteger) dataTable.getModel().getAbsoluteValueAt(row, ColumnsId.CODE.ordinal()))
.longValue();
var idMensaje = getMessageId(row, dataTable);
var answer = JOptionPane.showConfirmDialog(mainWindow,
MessageCatalog.BROWSER_RETRIEVE_MESSAGE_CONFIRMATION.getMessage(idMensaje, codigo),
es.ree.eemws.kit.gui.common.MessageCatalog.MSG_CONFIRM_TITLE.getMessage(),
JOptionPane.YES_NO_OPTION, JOptionPane.QUESTION_MESSAGE);
if (answer == JOptionPane.OK_OPTION) {
retieveWhenDisabled(row);
}
mainWindow.enableScreen(true);
}
}
/**
* Performs the message retrieval once the graphic components are disabled.
*
* @param row Row index.
* @see #retrieve().
*/
private void retieveWhenDisabled(final int row) {
var dataTable = mainWindow.getDataTable();
var idMensaje = getMessageId(row, dataTable);
Long codigo = ((BigInteger) dataTable.getModel().getAbsoluteValueAt(row, ColumnsId.CODE.ordinal())).longValue();
var msg = MessageCatalog.BROWSER_RETRIEVING_FILE.getMessage(idMensaje, codigo);
logger.logMessage(msg);
status.setStatus(msg);
try {
var response = get.get(codigo);
msg = MessageCatalog.BROWSER_RETRIEVED_FILE.getMessage(idMensaje, codigo);
logger.logMessage(msg);
status.setStatus(msg);
var ficheroHandle = mainWindow.getFileHandle();
ficheroHandle.saveFile(idMensaje, response);
} catch (GetOperationException e) {
msg = MessageCatalog.BROWSER_UNABLE_TO_GET.getMessage(e.getMessage());
status.setStatus(msg);
logger.logMessage(msg);
JOptionPane.showMessageDialog(mainWindow, msg,
es.ree.eemws.kit.gui.common.MessageCatalog.MSG_ERROR_TITLE.getMessage(), JOptionPane.ERROR_MESSAGE);
} catch (IOException e) {
msg = es.ree.eemws.kit.cmd.MessageCatalog.UNABLE_TO_WRITE.getMessage(idMensaje);
status.setStatus(msg);
logger.logMessage(msg);
JOptionPane.showMessageDialog(mainWindow, msg,
es.ree.eemws.kit.gui.common.MessageCatalog.MSG_ERROR_TITLE.getMessage(), JOptionPane.ERROR_MESSAGE);
}
}
/**
* Returns message identification as + "." + . If the message has
* no version only the part will be returned.
*
* @param row Table view row number.
* @param dataTable Data table.
* @return Identification of the message that appears at row row
*/
private String getMessageId(final int row, final DataTable dataTable) {
var idMensaje = new StringBuilder()
.append((String) dataTable.getModel().getAbsoluteValueAt(row, ColumnsId.ID.ordinal()));
var version = (BigInteger) dataTable.getModel().getAbsoluteValueAt(row, ColumnsId.VERSION.ordinal());
if (version != null) {
idMensaje.append(".").append(version.toString()); //$NON-NLS-1$
}
return idMensaje.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy