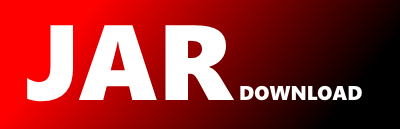
es.ree.eemws.kit.gui.applications.configuration.EnvironmentConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eemws-kit Show documentation
Show all versions of eemws-kit Show documentation
Client implementation of IEC 62325-504 technical specification. eemws-kit includes command line utilities to invoke the eem web services, as well as several GUI applications (browser, editor, ...)
The newest version!
/*
* Copyright 2024 Redeia.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, version 3 of the license.
*
* This program is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTIBIILTY or FITNESS FOR A PARTICULAR PURPOSE. See GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program. If not, see
* http://www.gnu.org/licenses/.
*
* Any redistribution and/or modification of this program has to make
* reference to Redeia as the copyright owner of the program.
*/
package es.ree.eemws.kit.gui.applications.configuration;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Properties;
/**
* Enviroment Configuration container. An environment configuations contains a
* system url a title and a small description. The intention of such
* configuation files is have multiple settings and easily switch between them.
*
* @author Redeia.
* @version 2.1 01/01/2024
*/
public class EnvironmentConfig {
/** Environment config URL key. */
private static final String URL_KEY = "URL";
/** Environment config DESCRIPTION key. */
private static final String DESC_KEY = "DESCRIPTION";
/** Environment config TITLE key. */
private static final String TITLE_KEY = "TITLE";
/** Max title lenght. */
private static final int MAX_TITLE_LENGTH = 30;
/** Max description lenght. */
private static final int MAX_DESC_LENGTH = 200;
/** This environmnet url. */
private URL url;
/** This environmnet description. */
private String desc;
/** This environmnet title. */
private String title;
/** If this configuration is not valid, this will store the reason. */
private String noValidReason = null;
/**
* Creates a new environment configuration reading the given properties file.
*
* @param envFileName Properties file where the configuration is stored.
*/
public EnvironmentConfig(final String envFileName) {
try (InputStream isProps = new FileInputStream(envFileName);) {
Properties envConf = null;
envConf = new Properties();
envConf.load(isProps);
readTitle(envConf);
readUrl(envConf);
readDesc(envConf);
} catch (IOException e) {
noValidReason = MessageCatalog.SETTINGS_SERVER_CANNOT_READ_ENV_FILE.getMessage();
}
}
/**
* Reads and validates the TITLE_KEY
attribute of the enviroment
* file.
*
* @param envConf Current environmnet properties file.
*/
private void readTitle(final Properties envConf) {
title = envConf.getProperty(TITLE_KEY);
if (title == null || title.isBlank()) {
noValidReason = MessageCatalog.SETTINGS_SERVER_ATTRIBUTE_IS_MANDATORY.getMessage(TITLE_KEY);
} else if (title.length() > MAX_TITLE_LENGTH) {
title = title.substring(0, MAX_TITLE_LENGTH);
}
}
/**
* Reads and validates the URL_KEY
attribute of the enviroment
* file.
*
* @param envConf Current environmnet properties file.
*/
private void readUrl(final Properties envConf) {
var urlStr = envConf.getProperty(URL_KEY);
try {
if (urlStr == null || urlStr.isBlank()) {
noValidReason = MessageCatalog.SETTINGS_SERVER_ATTRIBUTE_IS_MANDATORY.getMessage(URL_KEY);
} else {
url = new URL(urlStr);
if (!url.getProtocol().equals("https")) {
noValidReason = MessageCatalog.SETTINGS_SERVER_INVALID_URL_NO_HTTPS.getMessage(urlStr);
}
}
} catch (MalformedURLException e) {
noValidReason = MessageCatalog.SETTINGS_SERVER_INVALID_URL.getMessage(urlStr);
}
}
/**
* Reads the DESC_KEY
attribute of the enviroment file.
*
* @param envConf Current environmnet properties file.
*/
private void readDesc(final Properties envConf) {
desc = envConf.getProperty(DESC_KEY);
if (desc != null && desc.length() > MAX_DESC_LENGTH) {
desc = desc.substring(0, MAX_DESC_LENGTH);
}
}
/**
* Returns if this environment configuration is valid.
*
* @return true
if the configuration is valid. false
* otherwise.
*/
public boolean isValid() {
return noValidReason == null;
}
/**
* Returns this configuration error status.
*
* @return null
if the configuration has no error. Othewise a
* string decribing the errors that this configurations has.
*/
public String getErrorMessage() {
return noValidReason;
}
/**
* Returns this configuration title.
*
* @return This configuration title.
*/
public String getTitle() {
return title;
}
/**
* Returns this configuration description.
*
* @return This configuration description.
*/
public String getDescription() {
return desc;
}
/**
* Returns this configuration URL.
*
* @return This configuration URL.
*/
public URL getURL() {
return url;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy