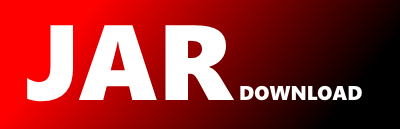
es.tid.pce.server.wson.DeleteReservationTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pce Show documentation
Show all versions of pce Show documentation
Path Computation Element
The newest version!
package es.tid.pce.server.wson;
import java.util.LinkedList;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import es.tid.tedb.DomainTEDB;
public class DeleteReservationTask implements Runnable {
private int wavelength;
boolean bidirectional;
private int m = 0;
boolean isMultipleLambdas;
private Logger log;
private LinkedList wlans;
private boolean isWLAN = false;
public boolean isBidirectional() {
return bidirectional;
}
public void setBidirectional(boolean bidirectional) {
this.bidirectional = bidirectional;
}
private LinkedList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy