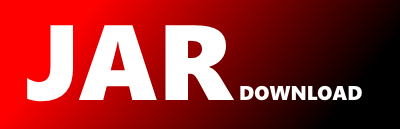
es.tid.tedb.MultiLayerTEDB Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of topology Show documentation
Show all versions of topology Show documentation
Traffic Engineering Database, BGP-LS peer, Topology Module
The newest version!
package es.tid.tedb;
import es.tid.ospf.ospfv2.lsa.tlv.subtlv.complexFields.BitmapLabelSet;
import org.jgrapht.graph.SimpleDirectedWeightedGraph;
import java.net.Inet4Address;
import java.util.*;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class MultiLayerTEDB implements DomainTEDB {
private ArrayList registeredAlgorithms;
/**
* Graph of the Upper Layer Network
*/
/**
*
*/
private SimpleDirectedWeightedGraph
© 2015 - 2025 Weber Informatics LLC | Privacy Policy