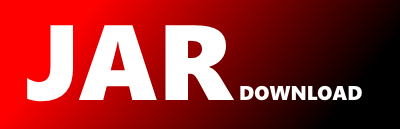
es.tid.tedb.elements.Node Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of topology Show documentation
Show all versions of topology Show documentation
Traffic Engineering Database, BGP-LS peer, Topology Module
The newest version!
package es.tid.tedb.elements;
import java.util.ArrayList;
public class Node {
String nodeID;
ArrayList address;
boolean isPhysical;
ArrayList intfList;
int domain;
Location location;
IPNodeParams ipParams;
String parentRouter;
String layer;
String configurationMode;
String dataPathID;
/**Router Vendor Type*/
String routerType;
String controllerIP;
String controllerPort;
/**IOS version*/
String rotuerModel;
ArrayList unnumberedIntfList=null;
public ArrayList getUnnumberedIntfList() {
return unnumberedIntfList;
}
public void setUnnumberedIntfList(ArrayList unnumberedIntfList) {
this.unnumberedIntfList = unnumberedIntfList;
}
public String getDataPathID() {
return dataPathID;
}
public void setDataPathID(String dpid) {
this.dataPathID = dpid;
}
public String getConfigurationMode() {
return configurationMode;
}
public void setConfigurationMode(String configurationMode) {
this.configurationMode = configurationMode;
}
public String getRouterType() {
return routerType;
}
public void setRouterType(String routerType) {
this.routerType = routerType;
}
public Object getControllerIP() {
return controllerIP;
}
public void setControllerIP(String controllerIP) {
this.controllerIP = controllerIP;
}
public Object getControllerPort() {
return controllerPort;
}
public void setControllerPort(String controllerPort) {
this.controllerPort = controllerPort;
}
public String getRotuerModel() {
return rotuerModel;
}
public void setRotuerModel(String iosVersion) {
this.rotuerModel = iosVersion;
}
/**
* @return the nodeID
*/
public String getNodeID() {
return nodeID;
}
/**
* @param nodeID the nodeID to set
*/
public void setNodeID(String nodeID) {
this.nodeID = nodeID;
}
/**
* @return the address
*/
public ArrayList getAddress() {
return address;
}
/**
* @param address the address to set
*/
public void setAddress(ArrayList address) {
this.address = address;
}
/**
* @return the isPhysical
*/
public boolean isPhysical() {
return isPhysical;
}
/**
* @param isPhysical the isPhysical to set
*/
public void setPhysical(boolean isPhysical) {
this.isPhysical = isPhysical;
}
/**
* @return the intfList
*/
public ArrayList getIntfList() {
return intfList;
}
/**
* @param intfList the intfList to set
*/
public void setIntfList(ArrayList intfList) {
this.intfList = intfList;
}
/**
* @return the domain
*/
public int getDomain() {
return domain;
}
/**
* @param domain the domain to set
*/
public void setDomain(int domain) {
this.domain = domain;
}
/**
* @return the location
*/
public Location getLocation() {
return location;
}
/**
* @param location the location to set
*/
public void setLocation(Location location) {
this.location = location;
}
/**
* @return the ipParams
*/
public IPNodeParams getIpParams() {
return ipParams;
}
/**
* @param ipParams the ipParams to set
*/
public void setIpParams(IPNodeParams ipParams) {
this.ipParams = ipParams;
}
/**
* @return the parentRouter
*/
public String getParentRouter() {
return parentRouter;
}
/**
* @param parentRouter the parentRouter to set
*/
public void setParentRouter(String parentRouter) {
this.parentRouter = parentRouter;
}
public String getLayer() {
return layer;
}
public void setLayer(String layer) {
this.layer = layer;
}
public String toString(){
String temp = "";
temp += "NodeID = " + nodeID +" " ;
if (location!=null)
temp += "Location = (" + location.xCoord + ", " + location.yCoord + ")" + " " ;
temp+= "Addresses (";
for (int i=0;i ();
address = new ArrayList ();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy