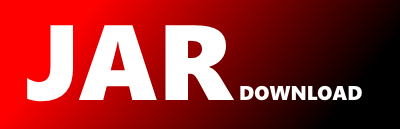
es.tid.topologyModuleBase.plugins.updaters.TopologyUpdaterThread Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of topology Show documentation
Show all versions of topology Show documentation
Traffic Engineering Database, BGP-LS peer, Topology Module
The newest version!
package es.tid.topologyModuleBase.plugins.updaters;
import java.net.Inet4Address;
import java.net.UnknownHostException;
import java.util.LinkedList;
import java.util.concurrent.LinkedBlockingQueue;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import es.tid.ospf.ospfv2.OSPFv2LinkStateUpdatePacket;
import es.tid.ospf.ospfv2.lsa.LSA;
import es.tid.ospf.ospfv2.lsa.LSATypes;
import es.tid.ospf.ospfv2.lsa.OSPFTEv2LSA;
import es.tid.ospf.ospfv2.lsa.tlv.LinkTLV;
import es.tid.ospf.ospfv2.lsa.tlv.subtlv.AvailableLabels;
import es.tid.ospf.ospfv2.lsa.tlv.subtlv.MaximumBandwidth;
import es.tid.ospf.ospfv2.lsa.tlv.subtlv.MaximumReservableBandwidth;
import es.tid.ospf.ospfv2.lsa.tlv.subtlv.UnreservedBandwidth;
import es.tid.ospf.ospfv2.lsa.tlv.subtlv.complexFields.BitmapLabelSet;
import es.tid.ospf.ospfv2.lsa.tlv.subtlv.complexFields.LabelSetField;
import es.tid.ospf.ospfv2.lsa.tlv.subtlv.complexFields.LabelSetParameters;
import es.tid.tedb.DomainTEDB;
import es.tid.tedb.IntraDomainEdge;
import es.tid.tedb.MultiLayerTEDB;
import es.tid.tedb.SimpleTEDB;
import es.tid.tedb.TE_Information;
public class TopologyUpdaterThread extends Thread{
private LinkedBlockingQueue ospfv2PacketQueue;
private Logger log;
private DomainTEDB TEDB;
/**
* Variables used to specify the range of lambdas the PCE is using
* Variables para especificar el rango de lambdas que el PCE esta usando, es decir,
* el rango de lambdas en el que puede calcular caminos
*/
private int lambdaIni=0;
private int lambdaEnd=Integer.MAX_VALUE;
private boolean isCompletedAuxGraph=false;
private boolean multilayer=false;
private int layer=LayerTypes.SIMPLE_NETWORK;
public TopologyUpdaterThread(LinkedBlockingQueue ospfv2PacketQueue, DomainTEDB TEDB, int lambdaIni, int lambdaEnd) {
log=LoggerFactory.getLogger("PCEServer");
this.ospfv2PacketQueue=ospfv2PacketQueue;
this.TEDB=TEDB;
this.lambdaIni=lambdaIni;
this.lambdaEnd=lambdaEnd;
}
public TopologyUpdaterThread(LinkedBlockingQueue ospfv2PacketQueue,DomainTEDB TEDB, int lambdaIni, int lambdaEnd,
boolean isCompletedAuxGraph, boolean multilayer) {
log=LoggerFactory.getLogger("PCEServer");
this.ospfv2PacketQueue=ospfv2PacketQueue;
this.TEDB=TEDB;
this.lambdaIni=lambdaIni;
this.lambdaEnd=lambdaEnd;
this.isCompletedAuxGraph=isCompletedAuxGraph;
this.multilayer=multilayer;
}
@Override
public void run() {
log.info("Starting Topology Upadater Thread");
LinkedList lsaList;
OSPFTEv2LSA lsa;
OSPFv2LinkStateUpdatePacket ospfv2Packet;
while(true){
try {
ospfv2Packet = ospfv2PacketQueue.take();
//log.info("OSPF PACKET READ");
//System.out.println("OSPF AREA: "+ospfv2Packet.getAreaID().toString());
Inet4Address domainId = (Inet4Address) Inet4Address.getByName("0.0.0.1");
TEDB.getReachabilityEntry().setDomainId(domainId);
//System.out.println("MY AREA: "+TEDB.getReachabilityEntry().getDomainId());
// Filtered by domain ID
if ((TEDB.getReachabilityEntry().getDomainId())!=null){
if(ospfv2Packet.getAreaID().toString().equalsIgnoreCase(this.TEDB.getReachabilityEntry().getDomainId().toString())){
//System.out.println("Adding OSPF Message");
// If ospf packet is from my domain
Inet4Address localInterfaceIPAddress = ospfv2Packet.getRouterID();
lsaList = (ospfv2Packet).getLSAlist();
for (int i =0;i< lsaList.size();i++){
if (lsaList.get(i).getLStype() == LSATypes.TYPE_10_OPAQUE_LSA){
lsa=(OSPFTEv2LSA)lsaList.get(i);
//log.info("Starting to process LSA");
LinkTLV linkTLV = lsa.getLinkTLV();
if (linkTLV!=null){
//log.info("Link TLV ha llegado "+lsa.toString());
//System.out.println(linkTLV.toString());
if (localInterfaceIPAddress == null){
localInterfaceIPAddress = linkTLV.getLocalInterfaceIPAddress().getLocalInterfaceIPAddress(0);
}
log.debug("Local InterfaceIPAddress: "+localInterfaceIPAddress);
Inet4Address remoteInterfaceIPAddress = linkTLV.getLinkID().getLinkID();
//Inet4Address remoteInterfaceIPAddress =linkTLV.getRemoteInterfaceIPAddress().getRemoteInterfaceIPAddress(0);
log.debug("Remote InterfaceIPAddress: "+remoteInterfaceIPAddress);
AvailableLabels newAvailableLabels = linkTLV.getAvailableLabels();
IntraDomainEdge edge = null;
if (multilayer){
if (isCompletedAuxGraph){
//tenemos que realizar la conversión a nodos y links del grafo auxiliar
if (newAvailableLabels!=null){
//System.out.println("MODIFICACIÓN GRAFO PARA ÓPTICA");
//Es de las capas 1-Numlabels
//Modificación en parte óptica
int lambda = 0;
lambda = ((BitmapLabelSet)linkTLV.getAvailableLabels().getLabelSet()).getDwdmWavelengthLabel().getN();
//System.out.println("Modificamos Grafo para la lambda :"+lambda);
((MultiLayerTEDB)TEDB).notificationEdgeOPTICAL_AuxGraph(localInterfaceIPAddress, remoteInterfaceIPAddress, lambda);
}
else{
//System.out.println("MODIFICACIÓN GRAFO PARA IP");
TE_Information informationTEDB = new TE_Information();
MaximumBandwidth maximumBandwidth = linkTLV.getMaximumBandwidth();
if (maximumBandwidth!= null){
informationTEDB.setMaximumBandwidth(maximumBandwidth);
//System.out.println("Meto el maximumBandwidth :"+maximumBandwidth.getMaximumBandwidth());
}
UnreservedBandwidth unreservedBandwidth = linkTLV.getUnreservedBandwidth();
if (unreservedBandwidth!=null){
informationTEDB.setUnreservedBandwidth(unreservedBandwidth);
//System.out.println("Meto el unreservedBandwidth :"+unreservedBandwidth.getUnreservedBandwidth()[0]);
}
MaximumReservableBandwidth maximumReservableBandwidth = new MaximumReservableBandwidth();
maximumReservableBandwidth.setMaximumReservableBandwidth(unreservedBandwidth.getUnreservedBandwidth()[0]);
if (maximumReservableBandwidth!= null){
informationTEDB.setMaximumReservableBandwidth(maximumReservableBandwidth);
//System.out.println("Meto el MaximumReservableBandwidth :"+maximumReservableBandwidth.maximumReservableBandwidth);
}
//Es de las capas "NumLabels + 1" o "NumLabels + 2"
((MultiLayerTEDB)TEDB).notificationEdgeIP_AuxGraph(localInterfaceIPAddress, remoteInterfaceIPAddress, informationTEDB);
}
}
else {
if (newAvailableLabels== null){
// caso Multilayer CAPA MPLS
//System.out.println("Link de "+localInterfaceIPAddress+" al dest "+remoteInterfaceIPAddress);
edge=((MultiLayerTEDB)TEDB).getUpperLayerGraph().getEdge(localInterfaceIPAddress, remoteInterfaceIPAddress);
layer = LayerTypes.UPPER_LAYER;
//System.out.println("Actualización grafo IP --> CAPA SUPERIOR");
}
else{
// caso MULTILAYER capa OPTICA
edge=((MultiLayerTEDB)TEDB).getLowerLayerGraph().getEdge(localInterfaceIPAddress, remoteInterfaceIPAddress);
layer = LayerTypes.LOWER_LAYER;
//System.out.println("Actualización grafo ÓPTICO --> CAPA INFERIOR");
}
}
}
else{
edge=((SimpleTEDB)TEDB).getNetworkGraph().getEdge(localInterfaceIPAddress, remoteInterfaceIPAddress);
layer = LayerTypes.SIMPLE_NETWORK;
}
boolean newEdge=false;
boolean newVertex =false;
boolean newEdge_virtual=false;
boolean changeWavelength = false;
boolean lighpath_flag = false;
BitmapLabelSet previousBitmapLabelSet=null;
BitmapLabelSet newBitmapLabelSet=null;
if (edge!=null){
//System.out.println("ACTUALIZACIÓN ÓPTICA O IP/MPLS SIN AÑADIR NUEVO LIGTH PATH");
}
if ((edge == null) && !(isCompletedAuxGraph)){
log.info("ADDING EDGE");
edge= new IntraDomainEdge();
if (layer == LayerTypes.SIMPLE_NETWORK){
((SimpleTEDB)TEDB).getNetworkGraph().addVertex(localInterfaceIPAddress);
((SimpleTEDB)TEDB).getNetworkGraph().addVertex(remoteInterfaceIPAddress);
if(linkTLV.getLinkLocalRemoteIdentifiers()!=null){
long src_if_Id = linkTLV.getLinkLocalRemoteIdentifiers().getLinkLocalIdentifier();
long dst_if_Id = linkTLV.getLinkLocalRemoteIdentifiers().getLinkRemoteIdentifier();
edge.setSrc_if_id(src_if_Id);
edge.setDst_if_id(dst_if_Id);
}
((SimpleTEDB)TEDB).getNetworkGraph().addEdge(localInterfaceIPAddress, remoteInterfaceIPAddress, edge);
}
else if (layer == LayerTypes.LOWER_LAYER){
((MultiLayerTEDB)TEDB).getLowerLayerGraph().addVertex(localInterfaceIPAddress);
((MultiLayerTEDB)TEDB).getLowerLayerGraph().addVertex(remoteInterfaceIPAddress);
((MultiLayerTEDB)TEDB).getLowerLayerGraph().addEdge(localInterfaceIPAddress, remoteInterfaceIPAddress, edge);
}
else {
//((MultiLayerTEDB)TEDB).getUpperLayerGraph().addEdge(localInterfaceIPAddress, remoteInterfaceIPAddress, edge);
newEdge_virtual = true;
if (newEdge_virtual){
TE_Information informationTEDB = new TE_Information();
MaximumBandwidth maximumBandwidth = linkTLV.getMaximumBandwidth();
if (maximumBandwidth!= null){
informationTEDB.setMaximumBandwidth(maximumBandwidth);
// System.out.println("Meto el maximumBandwidth");
}
UnreservedBandwidth unreservedBandwidth = linkTLV.getUnreservedBandwidth();
if (unreservedBandwidth!=null){
informationTEDB.setUnreservedBandwidth(unreservedBandwidth);
//System.out.println("Meto el unreservedBandwidth");
}
MaximumReservableBandwidth maximumReservableBandwidth = new MaximumReservableBandwidth();
maximumReservableBandwidth.setMaximumReservableBandwidth(unreservedBandwidth.getUnreservedBandwidth()[0]);
if (maximumReservableBandwidth!= null){
informationTEDB.setMaximumReservableBandwidth(maximumReservableBandwidth);
//System.out.println("Meto el MaximumReservableBandwidth");
}
((MultiLayerTEDB)TEDB).notifyNewEdgeIP(localInterfaceIPAddress, remoteInterfaceIPAddress, informationTEDB);
lighpath_flag = true;
}
}
if (layer == LayerTypes.LOWER_LAYER || layer == LayerTypes.SIMPLE_NETWORK){
newVertex=true;
newEdge=true;
}
}
if (lighpath_flag == false && !(isCompletedAuxGraph)){
TE_Information informationTEDB=edge.getTE_info();
if (informationTEDB == null){
informationTEDB = new TE_Information();
}
if (layer == LayerTypes.UPPER_LAYER){
if (newAvailableLabels == null){
MaximumBandwidth maximumBandwidth = linkTLV.getMaximumBandwidth();
if (maximumBandwidth!= null)
informationTEDB.setMaximumBandwidth(maximumBandwidth);
UnreservedBandwidth unreservedBandwidth = linkTLV.getUnreservedBandwidth();
if (unreservedBandwidth!=null)
informationTEDB.setUnreservedBandwidth(unreservedBandwidth);
//AvailableLabels
MaximumReservableBandwidth maximumReservableBandwidth = new MaximumReservableBandwidth();
maximumReservableBandwidth.setMaximumReservableBandwidth(unreservedBandwidth.getUnreservedBandwidth()[0]);
if (maximumReservableBandwidth!= null)
informationTEDB.setMaximumReservableBandwidth(maximumReservableBandwidth);
}
edge.setTE_info(informationTEDB);
}
else if (newAvailableLabels!= null){
if (newAvailableLabels.getLabelSet()!=null){
if (newAvailableLabels.getLabelSet().getAction()==LabelSetParameters.BitmapLabelSet){
//We store here the previous BitmapLabelSet, to know the differences
//log.info("TEMA BITMAP LABEL");
if (informationTEDB.getAvailableLabels() == null){
informationTEDB.setAvailableLabels(new AvailableLabels());
}
//Primera vez que llega la topologia. Creamos el LABELSET
if (informationTEDB.getAvailableLabels().getLabelSet() == null){
//log.info("TEMA BITMAP LABEL POR PRIMERA VEZ");
newBitmapLabelSet = new BitmapLabelSet();
previousBitmapLabelSet=newBitmapLabelSet;
newBitmapLabelSet.createBytesBitMap(((BitmapLabelSet)newAvailableLabels.getLabelSet()).getBytesBitMap());
int numLabels = linkTLV.getAvailableLabels().getLabelSet().getNumLabels();
int numberBytes = getNumberBytes(numLabels);
log.info("NUM LABELS: "+numLabels+" NUMBER BYTES: "+numberBytes);
byte[] bytesBitMapReserved = new byte[numberBytes];
for (int j=0;j
© 2015 - 2025 Weber Informatics LLC | Privacy Policy